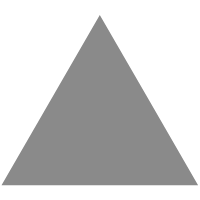
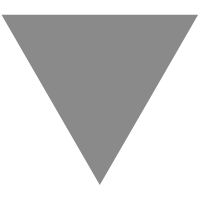
6 Clone Apps You Can Make When Learning C# Windows Forms
source link: https://www.makeuseof.com/windows-forms-create-clone-apps/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
6 Clone Apps You Can Make When Learning C# Windows Forms
Learn to develop Windows Forms applications by re-creating clone apps.
Windows Forms allows you to create desktop applications using Visual Studio. The main components of a Windows Forms application include the canvas and the code-behind.
The canvas is where you can drag and visualize UI elements such as labels onto the app. The code-behind is where you can write your programming logic and handle events.
When learning how to code in a new environment, it can be useful to learn from the experience of others. You can re-create these common desktop applications using Windows Forms, which can help you to learn more about how a Forms app works.
The Benefits of Re-Creating Existing Apps
There are common applications that other developers have created numerous times. This includes calculators, various converters, or to-do list apps. Because of this, these common apps usually have many tutorial guides or YouTube videos that you can follow.
Following these resources allows you to learn the different approaches that developers use to create these apps. This includes their approach to solving problems, or how they architect their app for maintainability.
1. Converter (Temperature, Measurement, and More)
A temperature converter is a great first Windows Forms application to build.
Here you will learn how to add UI elements to the canvas. In this case, you can start by adding two text boxes, which will represent the number to be converted, and the result. Then you can create a button to calculate the result when clicked.
This will also allow you to learn event handling. You can explore event handling in Microsoft's documentation to find out how to pair a button (i.e, the Calculate button) with a function. This function would trigger when the user clicks on the button.
private void button_num_Click(object sender, EventArgs e)
{
// Calculate the result and display it to the user.
}
You will also learn how to deal with invalid inputs. For this, you can explore the examples shown in Microsoft's Control.Visible Property documentation. This will allow you to add a UI label with an error message and only set the visibility to true if the input is invalid.
2. Calculator
Creating a calculator will also help you learn about event handling. This application contains buttons to represent the numbers and mathematical operations. Another UI element is the textbox, which is located at the top and outputs the answer.
When the user clicks on a button, you can keep updating a string that stores the ongoing mathematical equation. When they click on the equals button, you can use the Compute() function. As the Microsoft Compute() documentation explains, this function calculates the result of an expression that you pass to it:
output_result.Text = new DataTable().Compute(formattedCalculation, null).ToString();
You will also be able to learn more about the error and scenario handling. For instance, the user shouldn't be allowed to press the "+" button multiple times in a row. They should also receive an error message if they press the equals button with an invalid formula, such as "+-((4()x". You'll learn to handle all such cases while developing this app.
3. Simple File Manager
A simple file manager is another app that you can create using Windows Forms. To create this app, you can copy or modify the design of the existing Windows file manager on your computer.
You will also have to generate the user interface dynamically, instead of manually dragging UI elements onto the canvas.
For example, you may need to use arrays and dynamically create UI elements such as labels or icons for each row. For this, you can read Microsoft's Button class documentation for examples on how to create a new UI element.
Button button1 = new Button();
Controls.Add(button1);
You can also explore Microsoft's System.IO documentation to learn how to access directories and files from your local computer. The System.IO namespace includes C# classes such as the Directory Class and the File Class.
4. Dice Simulator
Creating a dice simulator is a great way to learn how to render graphics onto a Windows Form application. In this application, you will have buttons labeled from one to six. When the user selects a button, the corresponding face of the die would appear.
There are many ways that you can render the faces of the die. You can draw them yourself using built-in C# classes and methods. You can also source images for each shape of the die, and render them as images instead.
The Microsoft Graphics Class documentation lists methods you can use to draw shapes on the canvas. For example, you can use the FillEllipse method to draw a circle at certain x and y coordinates, with a specified radius.
e.Graphics.FillEllipse(solidBrush, 300, 50, 200, 200);
The Microsoft PictureBox documentation showcases a class that you can use to load and display a set of dice images stored on your computer.
5. Dice Game
There are various dice games that you can make using a Windows Forms Application. This application is more advanced than the dice simulator but gives you the chance to learn more about handling game states.
For instance, what triggers the start and end of the game, as well as certain events during the game based on certain game rules. An example of this would be if your game had a rule where if the user rolls a five, they get to roll the die for a second time.
You can explore the MessageBox class, particularly Microsoft's documentation of the MessageBox.Show method. This will allow you to create pop-ups to inform the user of what is going on in the game.
MessageBox.Show("The computer has rolled the dice. They have rolled a " + totalDiceRoll);
6. Flag Generator
This isn't necessarily a common application, but it can still be a very useful exercise. This application is similar to the dice generator. However, instead of generating faces of a die, you are using various shapes to generate different flags instead.
Here, you will need to explore a wider variety of C# methods from the Graphics class. This includes FillRectangle() and FillPolygon() to create different types of shapes. To create lines, you can use the DrawLine method. The Microsoft Graphics documentation includes details of all these methods.
You will also need to ensure you are positioning the shapes at the correct x and y coordinates across the flag.
Create More Apps While Learning Windows Forms
There are many common apps that you can re-create while learning how a Windows Forms application works. This includes various converters, calculator apps, file managers, or dice games. You can start creating these apps from scratch using a new Windows Forms project.
These example apps only touch the basics of desktop applications. You can also continue exploring other types of games you can create, and what platforms you can use to create them.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK