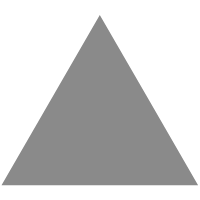
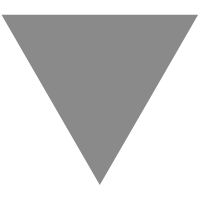
How to AJAX Submit a Form in jQuery
source link: https://www.laravelcode.com/post/how-to-ajax-submit-a-form-in-jquery
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
How to AJAX Submit a Form in jQuery
Use the jQuery $.post()
Method
You can simply use the $.post()
method in combination with the serialize()
method to submit a form using AJAX in jQuery. The serialize()
method creates a URL encoded text string by serializing form values for submission. Only "successful controls" are serialized to the string.
Let's try out the following example to understand how it basically work:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>jQuery AJAX Submit Form</title>
<script src="https://code.jquery.com/jquery-1.12.4.min.js"></script>
<script>
$(document).ready(function(){
$("form").on("submit", function(event){
event.preventDefault();
var formValues= $(this).serialize();
$.post("process_form.php", formValues, function(data){
// Display the returned data in browser
$("#result").html(data);
});
});
});
</script>
</head>
<body>
<form>
<p>
<label>Name:</label>
<input type="text" name="name">
</p>
<p>
<label>Gender:</label>
<label><input type="radio" value="male" name="gender"> Male</label>
<label><input type="radio" value="female" name="gender"> Female</label>
</p>
<p>
<label>Hobbies:</label>
<label><input type="checkbox" value="music" name="hobbies[]"> Music</label>
<label><input type="checkbox" value="sports" name="hobbies[]"> Sports</label>
<label><input type="checkbox" value="dance" name="hobbies[]"> Dance</label>
</p>
<p>
<label>Favorite Color:</label>
<select name="color">
<option>Red</option>
<option>Green</option>
<option>Blue</option>
</select>
</p>
<p>
<label>Comment:</label>
<textarea name="comment"></textarea>
</p>
<input type="submit" value="submit">
</form>
<div id="result"></div>
</body>
</html>
Here is the PHP script of our "process_form.php" file, which simply retrieve and output form elements values submitted by the user, which eventually sent back to the user's browser.
<h2>Here is the information submitted by you:</h2>
<p>Name: <b><?php echo $_POST["name"] ?? ''; ?></b></p>
<p>gender: <b><?php echo $_POST["gender"] ?? ''; ?></b></p>
<p>Favorite Color: <b><?php echo $_POST["color"] ?? ''; ?></b></p>
<p>Hobbies: <b><?php if(isset($_POST["hobbies"])){ echo implode(", ", $_POST["hobbies"]); } ?></b></p>
<p>Comment: <b><?php echo $_POST["comment"] ?? ''; ?></b></p>
Author : Harsukh Makwana

Hi, My name is Harsukh Makwana. i have been work with many programming language like php, python, javascript, node, react, anguler, etc.. since last 5 year. if you have any issue or want me hire then contact me on [email protected]
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK