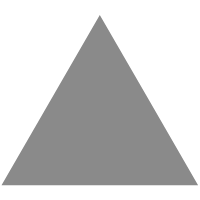
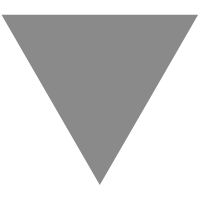
Recommendations for developers: the complete how-to, what-to, and where-to guide
source link: https://www.algolia.com/blog/engineering/recommendations-for-developers-the-complete-how-to-what-to-and-where-to-guide/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Recommendations for developers: the complete how-to, what-to, and where-to guide
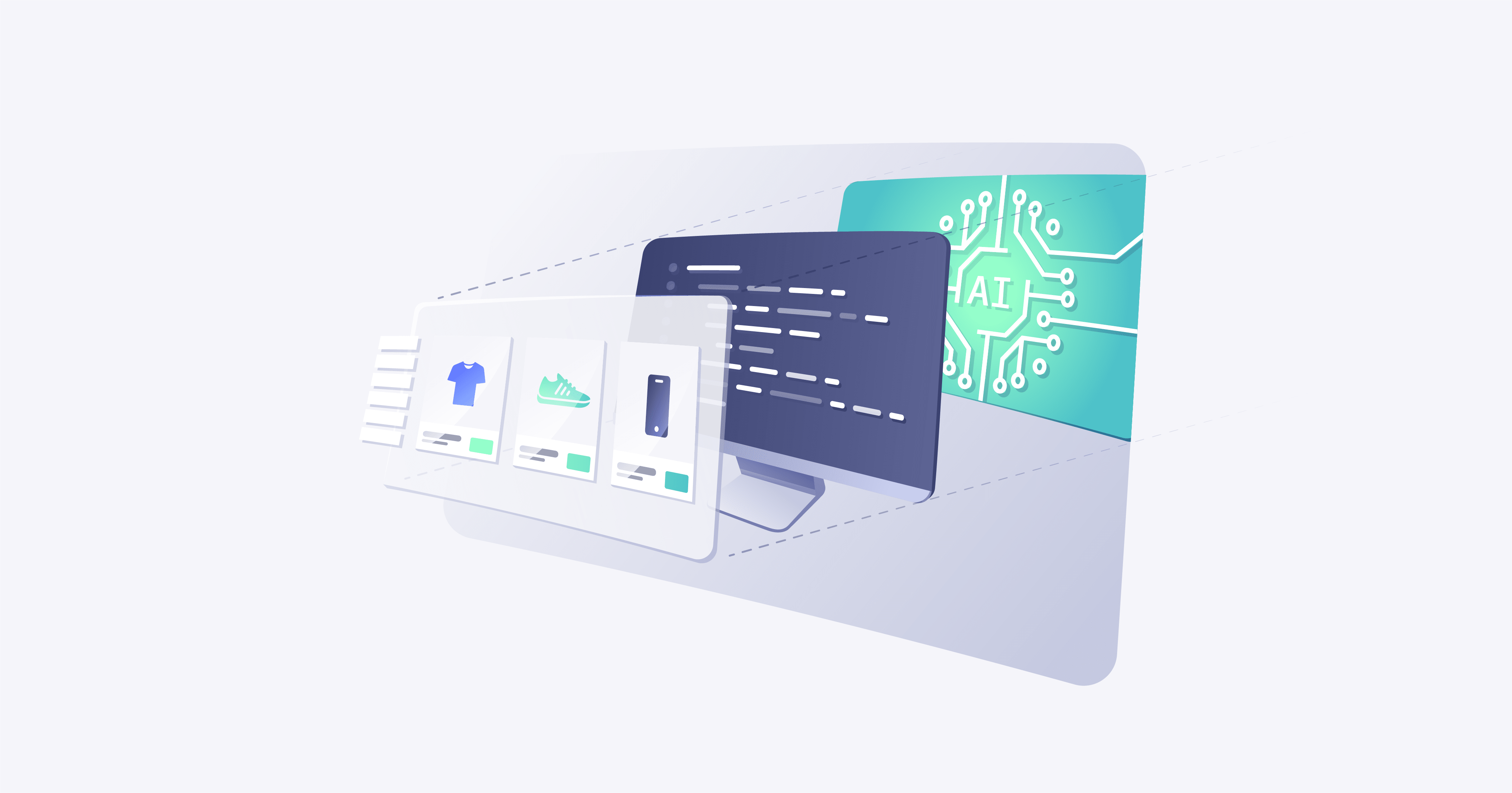

Recommendations are a must-have for online buyers and media consumers. They’ve become integral to finding the best digital content on the web. They show up as we type queries, browse results, view items, and make purchases.
In fact, we’re at a point where many users can’t even imagine shopping, watching movies, reading articles, or doing anything online without clicking on recommendations. There’s either too much content to choose from or the proposed recommendations are simply too relevant to resist.
To quantify this, nearly all online user activity, such as browsing, clicking, and converting, involve either search or recommendations. For example, 43% of shoppers on retail websites go directly to the search bar. Furthermore, recommendations account for a large bulk of the conversions on some leading websites:
- 70% of the content people watch on YouTube
- 35% of what consumers purchase on Amazon
- 80% of what people watch on Netflix
What does this mean for developers? How can developers with no background in machine-learning build the data, algorithms, and user interfaces that deliver such meaningful and relevant recommendations (and business results)?
Let’s take a look!
How to display recommendations on your UI
We’ll look at sample code to display and generate recommendations:
- The API – how to retrieve the recommendations
- The UI – how to display recommendations
- The Data – how to capture usage analytics with user events
When and where to display recommendations
You can display recommendations on any page. For example, on the home page, where you can recommend your most popular and trending items. As you’ll see below, recommendations use various machine-learning models to detect trending items.
But as your users navigate your site, they’ll want to see recommendations relevant to what they’re doing at any given moment. Here’s a list of pages where recommendations can be a great asset. They’re all related to one or more specific items a user is looking at:
- Product Detail Pages
- Search results pages
- Article Pages
- Video Pages
- Podcast Pages
- Check out pages
Finally, facets and categories: You can propose recommendations on items that fall within a certain facet or category. These recommendations can appear on the above list of pages, but they are most relevant on the following pages:
- Category landing pages, where the page shows items within a given facet or category.
- The home and search results pages, in the category carousels that Netflix and other media companies use.
How to retrieve recommendations – the code
Implementing recommendations on any of these pages requires only a single API call. Here are some examples (in JavaScript; see our Recommend API reference for all languages):
For the home page, you can display trending items:
recommendedItems = client.getTrendingGlobalItems([ indexName: 'your_index_name', threshold: 80 |
For the product detail page, you can display related items:
recommendedItems = client.getRelatedProducts([ indexName: 'your_index_name', objectID: 'your_item_id', |
For the check out page, you can display items frequently bought together:
recommendedItems = client.getFrequentlyBoughtTogether([ indexName: 'your_index_name', objectID: 'your_item_id', |
For the category page, you can display trending items within a specific category:
recommendedItems = client.getTrendingItemsForFacet([ indexName: 'your_index_name', threshold: 80, facetName: 'category', facetValue: 'sweaters' |
How to display recommendations – the UI
Finally, for the UI, you can use our InstantSearch front-end libraries to display any of the above API calls with the following 6 lines of code (see video demo below):
recommendedItems = relatedProducts({ container: '#relatedProducts', recommendClient, indexName: 'YourIndexName', objectIDs: ['YOUR_ITEM_ID'], itemComponent, |
See our Trends models feature spotlight article for more information. Or see a full Recommend tutorial here.
Capturing usage analytics with user events
As you’ll see, nearly all recommendations derive from capturing what users do as they click, view, and convert. Though not required to get started with Recommend, capturing relevant usage activity, in the form of Analytics events, and feeding that data into the recommendation engine’s machine-learning models, will up-level your recommendations.
Again, only a single API call is necessary to capture events, whether you send them from the front end or back end.
Here’s the code to send a click event from the front end:
insights_library('clickedObjectIDsAfterSearch', { userToken: 'user-123456', eventName: 'Product Clicked', index: 'products', queryID: 'cba8245617aeace44', objectIDs: ['9780545139700'], positions: [7], |
Here’s the code to send a conversion event after a search:
insights_library('convertedObjectIDsAfterSearch', { userToken: 'user-123456', index: 'products', eventName: 'Product Wishlisted', queryID: 'cba8245617aeace44', objectIDs: ['9780545139700', '9780439785969'] |
Get started with sending analytics events here.
Under the hood – the recommendations data & models in a nutshell
The data
Most of what we search for online involves structured content. That is, we search websites whose data contains clearly-defined items, such as products, articles, and films, where each item (product, film, or article) is a record with attributes. Search engines call this data an index, normally represented as a schema-less collection of records with attributes. Think JSON.
The job of the search engine is to return every record whose attributes match the characters in a query. What’s the job of the recommendation engine? For starters, the recommendation engine uses the same records (and their attributes) as used by your search engine.
There’s also a second dataset that contains the actual recommendations. Because the recommender engine uses multiple learning models, this second dataset must be prepared in a certain way to allow the engine to apply its various learning models. As described below, recommendations use machine-learning models to learn about the items and build relationships between the items.
With this data, the recommendations engine builds relationships between items based on:
- The data (“Content-Based Filtering”)
- User behavior & events (“Collaborative Filtering”)
1. Relationships based on the data
An engine uses the ML model Content-Based Filtering to generate groups of items based on similarity, where the similarity comes from comparing the contents of attributes of all the records. Records whose attributes significantly match each other are considered similar and therefore grouped together. A recommendations engine uses these groups to recommend related records based on their inclusion within the same group.
For example, a recommender engine can read an index and see that some items contain attributes with data like “sneakers” “city dwellers” “runners”; it can do this by looking at the content in the title, description, and other attributes (title takes priority). If it sees enough of this same data, it can group together all records with all three criteria. Let’s call this group “city-runner-sneakers”. Later, when the recommender engine sees that a user is selecting an item in the “city-runner-sneakers” group, it can recommend other items in the group.
One benefit to using content-based filtering is that you can start displaying recommendations as soon as day one – you don’t need to wait for the user analytics required in the next model. However, a content-based filtering model can also be combined with analytics (we call this a “hybrid engine” model) to generate even stronger relationships, as we’ll see now.
2. Relationships based on user behavior & events
An engine can also use the ML model Collaborative Filtering to generate groupings based on what users do with the records in an index. As users click or view or buy or convert in any other way, the recommender engine can start grouping items as “related”, “frequently-bought-together”, or “trending”. These relationships do not need to look at the data; instead, they rely entirely on how users interact with the data.
Collaborative-based recommendations essentially rely on prior user activity to group items that have a similar set of clicks and conversions. Broadly speaking, the idea is that when many users click or convert on different items, the engine can start detecting click and conversion patterns that some items have in common. Items that share the same patterns are considered related. This is referred to as item-based profiling. Here’s an example.
We can create a profile like “Customers who watch rock music documentaries”. This is done by capturing activity such as users’ search terms, clicks, and conversions. If users query the “Beatles” and click on their “Get Back” and ”Let It Be” documentaries, this indicates a pattern. And if many users take similar actions for other musical artists (searching for musical artists and clicking on their documentaries), the recommender engine will confirm that there’s a musical-documentary profile in which many items might fall into. From that point forward, the engine can start recommending the most commonly-viewed musical documentaries whenever a user indicates this preference (by searching for or viewing a musical documentary).
That’s the general logic. Now let’s see exactly what Algolia has to offer.
What recommendation models Algolia Recommend offers
Algolia released its first version of Recommend in 2021 with two models. Since then, Recommend has evolved to offer additional recommendations models. In general terms, our models fall into three broad categories:
- Related content (media content, products)
- Frequently bought (watched, read, …) together
- Trending (items and facets)
Related Content (and Related Products)
Algolia applies a hybrid approach, using both collaborative and content-based filtering models, to generate related content.
Note: you can get started with Recommend models like Related Content and Related Products, solely with content-based filtering, without the need for collaborative (events-based) data. We’ll explain how collaborative filtering (that can be added over time) up-levels the experience, though.
Starting with collaborative filtering, Recommend groups items based on item profiles. The way it works is as follows: whenever an item is clicked, we store the object ID of that item with the ID of the user who clicked on the item. When Recommend captures enough user clicks and conversions to establish an item’s profile – a minimum of 10,000 events by 10 users – it will have enough user events to create meaningful item profiles. At that point, a user viewing a profiled item can receive recommendations for other items that fall within the same item profile.
To strengthen these item relationships, we add content-based filtering, finding similarities in the attributes of the records, as described above. We then combine the results of both models, keeping the strongest relationships of each model.
One important piece here is that since related content uses content-based filtering, you can start displaying recommendations even before you’ve amassed the 10K events needed for the collaborative filtering.
Frequently bought together (or watched, read, etc.)
Based on collaborative filtering (only conversions), Recommend generates item profiles based on the number of users who convert (buy, add to cart, view) two or more items together. Recommend needs at least 1000 conversion events on two or more items by 10 users to start generating meaningful recommendations.
We usually see “Frequently bought together” in three circumstances: when a user is viewing an item, adding an item to a cart, or just after purchasing an item.
Conversion events are agnostic: they can be used to indicate if items have been watched or read together, not only bought. So “frequently done together” is a more general understanding of this model.
Trends
With trends, we drop the need for user ID and focus only on an item’s or facet’s recent popularity. The essential calculation is to use statistics to determine which items have the strongest trend upward of conversions. We offer three kinds of trends:
- Globally trending items
-
- We look at items in the whole catalog and recommend the ones with the strongest trend upward of conversions.
- Trending items, filtered by facets
-
- We look at all items within a facet or category and recommend the ones with the strongest trend upward of conversions.
- Trending facets
-
- We look at facets and recommend the facets whose items have the strongest trend upward of conversions.
The anonymity of recommendations
As you’ve no doubt seen, we rely heavily on capturing user IDs to create profiles. While this raises legitimate concerns, we’ve built our machine-learning models to ensure complete anonymity.
So, whenever we mention user ID, everything is kept anonymous, which means that when we save a user ID, we do not save any personal characteristic other than this unique ID. We’re only interested in the ID’s uniqueness to help group similar items.
The profiles that we create are item profiles, which means that items with similar sets of user behavior are grouped together. Thus, we’re not interested in profiling users, and so we don’t need to store any personal information. We leverage the user ID only to strengthen the model’s generation of item profiles.
Next steps
As we’ve discussed, our models can display recommendations on many different parts of your website. Whatever stage of the customer journey you are looking to optimize, we can help! Sign up for free to try Recommend.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK