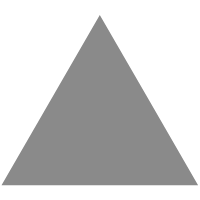
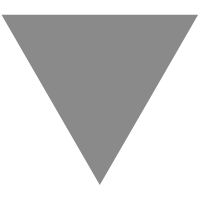
OnScroll Load More in Laravel 8 with Livewire Package
source link: https://www.laravelcode.com/post/onscroll-load-more-in-laravel-8-with-livewire-package
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
OnScroll Load More in Laravel 8 with Livewire Package
In this article, i will share with you how to make onscroll load more functionality in laravel 8 using the live wire package with a simple example.
Load more or infinite scrolling is the very best way to data display when you have a lot of data in your application and you don't want to use pagination in your application to display the data on the front end side. basically, user's no need to click on another link to show the another and the old data. Users only need to stay on one page and scroll the page and see more data on your application. see type functionality you generally see in the e-commerce side.
Here, we make some type of functionality in laravel using the livewire package. if before you never work or use livewire package in laravel then don't worry in this article we make it from scratch so you do need to know details of the livewire package functionality, just follow all steps.
Step - 1 : Create Laravel 8 Application
First, wee need to create the laravel 8 application help of running the following command in your terminal.
composer create-project laravel/laravel laravel-load-more-example --prefer-dist
Step - 2 : Database Setup
Now, you open your .env file and make sure your database setting is perfect.
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=db
DB_USERNAME=root
DB_PASSWORD=
Step - 3 : Create Test Data
Now, we create some test data in the users table using the laravel factory.
User::factory()->count(200)->create()
Step - 4 : Install LiveWire
In this step we install the livewire package in our laravel 8 application.
composer require livewire/livewire
Step - 5 : Build Laravel Load More Component
Now, we need to make our load more components help of running the livewire command in our terminal. livewire provide some console command for easy to use.
make:livewire load-more-user-data
after running the above command in our laravel8 application root path then the following two files crerated automatically in our laravel 8 application.
app/Http/Livewire/LoadMoreUserData.php
resources/views/livewire/load-more-user-data.blade.php
Open and update the below code in app/Http/Livewire/LoadMoreUserData.php file:
namespace App\Http\Livewire;
use Livewire\Component;
use App\Models\User;
class LoadMoreUserData extends Component
{
public $limitPerPage = 10;
protected $listeners = [
'load-more' => 'loadMore'
];
public function loadMore()
{
$this->limitPerPage = $this->limitPerPage + 6;
}
public function render()
{
$users = User::latest()->paginate($this->limitPerPage);
$this->emit('userStore');
return view('livewire.load-more-user-data', ['users' => $users]);
}
}
update the below code in resources/views/livewire/load-more-user-data.blade.php file:
<div>
<table class="table table-striped">
<thead>
<tr>
<th>#Id</th>
<th>Name</th>
<th>Email</th>
</tr>
</thead>
<tbody>
@foreach($users as $user)
<tr>
<td>{{ $user->id }}</td>
<td>{{ $user->name }}</td>
<td>{{ $user->email }}</td>
</tr>
@endforeach
</tbody>
</table>
</div>
Step - 6 : Add Route in Laravel
use Illuminate\Support\Facades\Route;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
*/
Route::get('/load-more-users', function () {
return view('welcome');
});
Step - 7 : Setting Up View
open the resources/views/welcome.blade.php file and add the following code:
<!DOCTYPE html>
<html lang="{{ str_replace('_', '-', app()->getLocale()) }}">
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Laravel Livewire Load More Data on Page Scroll Demo</title>
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet">
<style>
html,
body {
height: 100vh;
}
</style>
</head>
<body>
<div class="container mt-4">
@livewire('load-more-user-data')
</div>
@livewireScripts
<script type="text/javascript">
window.onscroll = function (ev) {
if ((window.innerHeight + window.scrollY) >= document.body.offsetHeight) {
window.livewire.emit('load-more');
}
};
</script>
</body>
</html>
Step - 8 : Test Application
Now, run your laravel application in the browser, just run the following command in your terminal.
php artisan serve
and hit the following url in your browser
http://localhost:8000/load-more-users
i hope you like thi article.
Author : Harsukh Makwana

Hi, My name is Harsukh Makwana. i have been work with many programming language like php, python, javascript, node, react, anguler, etc.. since last 5 year. if you have any issue or want me hire then contact me on [email protected]
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK