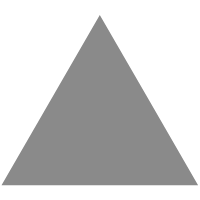
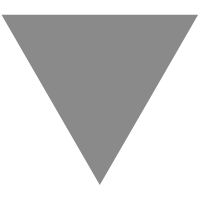
jQuery CSS Method
source link: https://jintechflow.wordpress.com/2021/03/22/jquery-css-method/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Introduction
Suppose you’re enjoying jQuery or just getting to know this jQuery library. In my opinion, it is fantastic to learn how to manipulate the CSS of an element by adding and changing the inline style or adding and removing CSS classes by manipulating their class attributes. That’s why in this article, we’re going to see how we can use the.css()
method of jQuery, including its related-attribute methods.
Background
If you’re an absolute beginner, you must know the concept and difference between an id and a class with an HTML element and a CSS stylesheet. If you know these concepts, you won’t be intimidated. Trust me, most of these methods are easy to understand.
The code sample is available on Github, and if you’re a more visual learner, I have created a video just for you. You can watch the video before you read the entire article or after so I’ll just put the video in the next section.
Ok, let’s get started.
jQuery css Method Video Tutorials
Code Sample
For our readers to easily visualize our screenshots and appreciate the code sample, we’ll provide the HTML, CSS, and jQuery code below. Moreover, to know how the jQuery scripts work is discussed in the next section.
<! DOCTYPE html> < html lang = "en" > < head > < meta charset = "UTF-8" > < meta http-equiv = "X-UA-Compatible" content = "IE=edge" > < meta name = "viewport" content = "width=device-width, initial-scale=1.0" > integrity = "sha256-u7e5khyithlIdTpu22PHhENmPcRdFiHRjhAuHcs05RI=" crossorigin = "anonymous" ></ script > < title >jQuery CSS Methods</ title > < style > .container { margin: auto; width: 30%; } .text-center { text-align: center; } .width-50 { width: 50%; } .box1 { background-color: #f5cd7e; border: 10px solid #e8e8e8; height: 200px; } .borderWithCorner { border-radius: 50px; } .happyDog { background-image: url('img/dog_in_yellow_1.png'); background-position: center; background-repeat: no-repeat; } .menus { padding-top: 10px; } .menus button { border-radius: 5px; width: 100%; margin: 5px; cursor: pointer; } </ style > </ head > < body > < div class = "container" > < h2 class = "text-center" >jQuery css() Method</ h2 > < h3 class = "text-center" >addClass(), removeClass(), hasClass() & toggleClass()</ h3 > < p >This method actually sets and gets the style property of an element</ p > < div class = "box1" ></ div > < div class = "menus" > < button id = "btn1" >Change background-color</ button > < button id = "btn2" >bckgrnd-color, txt-color & bold</ button > < button id = "btn3" >Toggle rounded-corners</ button > < button id = "btn4" >Toggle 2 rounded-corners</ button > < button class = "width-50" id = "btn5" >+</ button > < button class = "width-50" id = "btn6" >-</ button > </ div > </ div > < script > const container = $(".container"), box1 = $(".box1"), button1 = $("#btn1"), button2 = $("#btn2"), button3 = $("#btn3"), button4 = $("#btn4"), button_size = $(".width-50"); let previousSubtractedSize = 0; button1.on('click', function (evt) { //set $(this).css('background-color', '#7ef5af'); //get let color = $(this).css('color').toString(); console.log(color); console.log(color === 'rgb(0, 0, 0)'); console.log('color is black'); }); button2.on('click', function (evt) { // $(this) // .css('background-color', '#7ef5af') // .css('color', '#f57e89') // .css('font-weight', 'bold'); /* * we can optimize this by using * .css({}) by passing an object literal */ $(this) .css({ 'background-color': '#7ef5af', 'color': '#f57e89', 'font-weight': 'bold' }); }); button3.on('click', function (evt) { /* * Check whether if the element * has the class 'borderWithCorner' * if doesn't have add it otherwise remove it. */ if (!$(box1).hasClass('borderWithCorner')) { $(box1).addClass('borderWithCorner'); } else { $(box1).removeClass('borderWithCorner'); } }); button4.on('click', function (evt) { //$(box1).toggleClass('borderWithCorner'); //don't forget the happy dog!!!! $(box1).toggleClass('borderWithCorner happyDog'); }); button_size.on('click', function (evt) { let symbol = $(this).text(); if (symbol === '+') { $(box1).css({ width: function (index, value) { previousSubtractedSize = parseFloat(value) * 0.5; return parseFloat(value) + (previousSubtractedSize); } }); } else { $(box1).css({ width: function (index, value) { return parseFloat(value) - (previousSubtractedSize); } }); } }); </ script > </ body > </ html > |
The css(),addClass(),removeClass() and toggleClass() Methods
In the jQuery API documentation page the .css()
method is categorized as style-properties while .addClass()
, .removeClass()
and .toggleClass()
is categorized as class-attribute.
Please see the screenshots below.

The css() Method
If you want to get or set a particular element’s style properties, this method exactly does that for you.
If you want to get the current value of a given element’s style property, you can pass the property style.
In our code sample, within the button1 click function from line 89 to line 94, we have seen how to get the color value of the button color.
Let’s see the sample below.
//get let color = $( this ).css( 'color' ).toString(); console.log(color); console.log(color === 'rgb(0, 0, 0)' ); console.log( 'color is black' ); |
How about setting the elements CSS?
There are two ways to do it. First, we can pass a single CSS style-property or by calling the .css()
method and passing a single CSS style-property multiple times. Second, we can pass an object literal to the .css()
method.
In our code sample, within the button2 click function from line 99 to 114, we can see how to set the CSS style-properties in two ways.
Let’s see the sample below.
$( this ) .css( 'background-color' , '#7ef5af' ) .css( 'color' , '#f57e89' ) .css( 'font-weight' , 'bold' ); /* * we can optimize this by using * .css({}) by passing an object literal */ $( this ) .css({ 'background-color' : '#7ef5af' , 'color' : '#f57e89' , 'font-weight' : 'bold' }); |
Output
The hasClass(), addClass() and removeClass() Method
According to the jQuery API documentation, these methods inspect and manipulate the CSS classes assigned to elements. Moreover, these methods are easy to understand because the method name is self-defined.
Just remember, .addClass()
method adds a class to a certain element, .hasClass()
method checks for matched class and .removeClass()
method removes the class from an element. Lastly, with .addClass()
and .removeClass()
you can add or remove multiple classes by separating them with a space e.g. $(“class1 class2”)
.
Let’s see an example below on how we can quickly implement these methods.
Please check the button3 click function from line 117 to 130.
/* * Check whether if the element * has the class 'borderWithCorner' * if doesn't have add it otherwise remove it. */ if (!$(box1).hasClass('borderWithCorner ')) { $(box1).addClass(' borderWithCorner '); } else { $(box1).removeClass(' borderWithCorner'); } |
Output
The toggleClass() Method
If you think .hasClass()
, .addClass()
and .removeClass()
can be done with a single method you’re right that’s why we have the .toggleClass()
method. Thereby, instead of having a condition before we can either add or remove a class, we can use it much more efficiently.
Let’s see an example below on how we can implement .toggleClass()
.
Please check the button4 click function from line 132 to 138.
button4.on( 'click' , function (evt) { //$(box1).toggleClass('borderWithCorner'); //don't forget the happy dog!!!! $(box1).toggleClass('borderWithCorner happyDog'); }); |
However, if you’re interested, we can take a sneak peek at the .toggleClass().
See the code block below.
The css() Method by Passing a Function as the Property Value
Since jQuery version 1.4, .css()
method has allowed developers to pass a function as the property value.
Let’s see an example below.
button_size.on( 'click' , function (evt) { let symbol = $( this ).text(); if (symbol === '+' ) { $(box1).css({ width: function (index, value) { previousSubtractedSize = parseFloat(value) * 0.5; return parseFloat(value) + (previousSubtractedSize); } }); } else { $(box1).css({ width: function (index, value) { return parseFloat(value) - (previousSubtractedSize); } }); } }); |
Summary
This post has shown the related CSS methods of jQuery such as .css()
, .addClass()
, .removeClass()
,and .toggleClass().
And of course, we have shown how we can pass a function using the .css()
method.
I hope you have enjoyed this article. Stay tuned for more. Until next time, happy programming!
Please don’t forget to bookmark, like, and comment. Cheers! And Thank you!
Ibahagi ito:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK