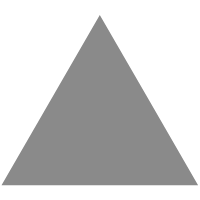
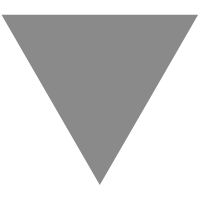
Python MongoDB Tutorial using Docker
source link: https://medium.com/codervlogger/python-mongodb-tutorial-using-docker-52f330852b4c
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Python MongoDB Tutorial using Docker
In this video, I will show how you can use Python to get connected to MongoDB. Using 2 sample programs we will write some information to the database and then receive it and iterate over the data and print to the console output.
We will not install MongoDB locally, instead we will use Docker and run official Mongo image.
Then, using following command we start it
docker run -d -p 27017:27017 --name m1 mongo
Here, one of the important part is -p option:
-p 27017:27107 exposes port 27017 so we can connect to mongodb instance from our local machine.
Once we have MongoDB running, we can start with Python code. First, let’s install PyMongo package:
pip install pymongo
Make sure you run this command within virtualenv so you do not install unnecessary packages globally. Shortly, you can setup virtualenv using following commands:
cd your-project-directory
virtualenv venv -p python3
source ./venv/bin/activate
For more information on pip and venv you can check this video:
Once we have Python project setup. We can use following code snippets to connect to MongoDB database and insert some data:
import pymongo # package for working with MongoDBclient = pymongo.MongoClient("mongodb://localhost:27017/")
db = client["customersdb"]
customers = db["customers"]customers_list = [
{ "name": "Amy", "address": "Apple st 652"},
{ "name": "Hannah", "address": "Mountain 21"},
{ "name": "Michael", "address": "Valley 345"},
{ "name": "Sandy", "address": "Ocean blvd 2"},
{ "name": "Betty", "address": "Green Grass 1"},
{ "name": "Richard", "address": "Sky st 331"},
{ "name": "Susan", "address": "One way 98"},
{ "name": "Vicky", "address": "Yellow Garden 2"},
{ "name": "Ben", "address": "Park Lane 38"},
{ "name": "William", "address": "Central st 954"},
{ "name": "Chuck", "address": "Main Road 989"},
{ "name": "Viola", "address": "Sideway 1633"}
]x = customers.insert_many(customers_list)# print list of the _id values of the inserted documents:
print(x.inserted_ids)
This code will open a connection, then insert some dummy data into so called customersdb database and customers collection (tables in relation database).
To get data and print it we can use following code:
import pymongoclient = pymongo.MongoClient("mongodb://localhost:27017/")
db = client["customersdb"]
customers = db["customers"]for x in customers.find():
print(x)
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK