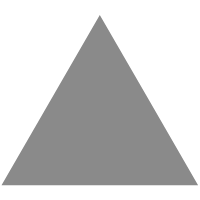
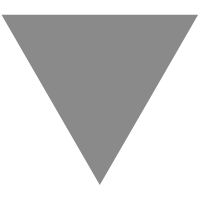
[Golang] Parse Accept-Language in HTTP Request Header
source link: http://siongui.github.io/2015/02/22/go-parse-accept-language/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
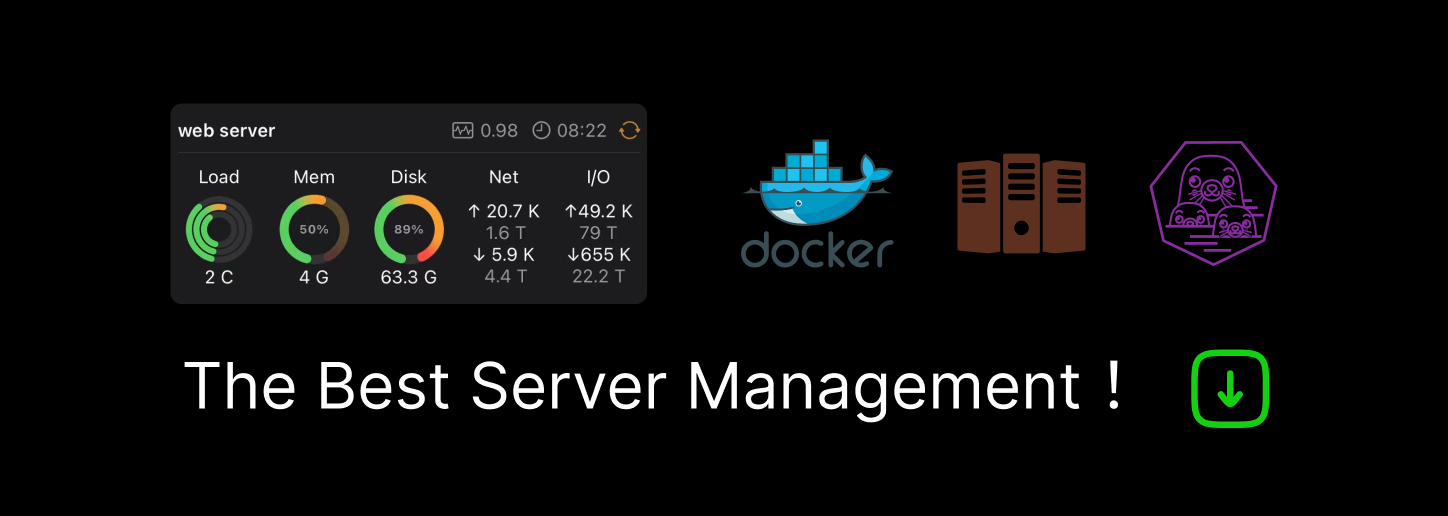
[Golang] Parse Accept-Language in HTTP Request Header
February 22, 2015
This post is the Go version of my previous post "[Python] Parse Accept-Language in HTTP Request Header" [1]. This Go example parses Accept-Language string in HTTP request header and output (languageTag, quality) pairs:
parse.go | repository | view raw
// http://golang.org/pkg/strings/ // http://stackoverflow.com/questions/16551354/how-to-split-string-and-assign // http://stackoverflow.com/questions/22688010/how-to-trim-leading-and-trailing-white-spaces-of-a-string-in-golang package locale import ( "strconv" "strings" ) type LangQ struct { Lang string Q float64 } func ParseAcceptLanguage(acptLang string) []LangQ { var lqs []LangQ langQStrs := strings.Split(acptLang, ",") for _, langQStr := range langQStrs { trimedLangQStr := strings.Trim(langQStr, " ") langQ := strings.Split(trimedLangQStr, ";") if len(langQ) == 1 { lq := LangQ{langQ[0], 1} lqs = append(lqs, lq) } else { qp := strings.Split(langQ[1], "=") q, err := strconv.ParseFloat(qp[1], 64) if err != nil { panic(err) } lq := LangQ{langQ[0], q} lqs = append(lqs, lq) } } return lqs }
Test program for the above code:
parse_test.go | repository | view raw
package locale import "testing" func TestParseAcceptLanguage(t *testing.T) { t.Log("da, en-gb;q=0.8, en;q=0.7") lqs := ParseAcceptLanguage("da, en-gb;q=0.8, en;q=0.7") if lqs[0].Lang != "da" { t.Error(lqs[0].Lang) } if lqs[0].Q != 1 { t.Error(lqs[0].Q) } if lqs[1].Lang != "en-gb" { t.Error(lqs[1].Lang) } if lqs[1].Q != 0.8 { t.Error(lqs[1].Q) } if lqs[2].Lang != "en" { t.Error(lqs[2].Lang) } if lqs[2].Q != 0.7 { t.Error(lqs[2].Q) } t.Log(lqs) t.Log("zh, en-us; q=0.8, en; q=0.6") lqs2 := ParseAcceptLanguage("zh, en-us; q=0.8, en; q=0.6") t.Log(lqs2) t.Log("es-mx, es, en") lqs3 := ParseAcceptLanguage("es-mx, es, en") t.Log(lqs3) t.Log("de; q=1.0, en; q=0.5") lqs4 := ParseAcceptLanguage("de; q=1.0, en; q=0.5") t.Log(lqs4) }
Makefile for automating the development:
Makefile | repository | view raw
# cannot use relative path in GOROOT, otherwise 6g not found. For example, # export GOROOT=../go (=> 6g not found) # it is also not allowed to use relative path in GOPATH export GOROOT=$(realpath ../../../../go) export GOPATH=$(realpath .) export PATH := $(GOROOT)/bin:$(GOPATH)/bin:$(PATH) test: @# -v means verbose, can see logs of t.Log @go test -v fmt: go fmt *.go help: go help
The output after run make:
=== RUN TestParseAcceptLanguage --- PASS: TestParseAcceptLanguage (0.00s) parse_test.go:6: da, en-gb;q=0.8, en;q=0.7 parse_test.go:14: [{da 1} {en-gb 0.8} {en 0.7}] parse_test.go:16: zh, en-us; q=0.8, en; q=0.6 parse_test.go:18: [{zh 1} {en-us 0.8} {en 0.6}] parse_test.go:20: es-mx, es, en parse_test.go:22: [{es-mx 1} {es 1} {en 1}] parse_test.go:24: de; q=1.0, en; q=0.5 parse_test.go:26: [{de 1} {en 0.5}] PASS ok _/home/koan/dev/nstechdev/userpages/content/code/go-accept-language 0.003s
Tested on: Ubuntu Linux 14.10, Go 1.4.
References:
[2]Detect User Language (Locale) on Google App Engine Python
[3]strings - The Go Programming Language
[4]go - How to split string and assign? - Stack Overflow
[5]go - How to trim leading and trailing white spaces of a string in golang? - Stack Overflow
[6]strconv - The Go Programming Language
Recommend
-
13
Accept header vs Content-Type Header I occasionally get confused between the Accept and the Content-Type Headers and this post is a way of clarifying the difference for myself. Let me summarize the difference to start...
-
33
作者个人研发的在高并发场景下,提供的简单、稳定、可扩展的延迟消息队列框架,具有精准的定时任务和延迟队列处理功能。自开源半年多以来,已成功为十几家中小型企业提供了精准定时调度方案,经受住了生产环境的考验。为使更多童鞋受益,现给出开源框架...
-
5
简单认识 HTTP 协议 HTTP 协议是一种基于 TCP 的纯文本协议,一个基本的 HTTP 协议交互过程如下: 使用 nc 命令演示以及 mock 最基本的 http server 交互响应过程: 终端 1: nc -kl 8080 终端 2:...
-
5
[Feature request] Set HTTP header to opt out of FLoC in GitHub PagesFor GitHub Pages, we ought to be able to opt out of computation in Google’s Federated Learning of Cohorts (FLoC) 1.8k. ...
-
7
Introduction Access HTTP Request Header in Golang code running on your browser. This can be done by
-
26
The Accept-Language field in HTTP Request header is important for
-
17
Introduction To detect browser language preference (user locale), one of the solution is to access Accept-Langu...
-
9
[JavaScript] Parse Accept-Language in HTTP Request Header January 22, 2016 ...
-
15
[Golang] Get HTTP Response Header March 06, 2018 Example of printing HTTP resp...
-
11
Available fields and functions for HTTP request header modificationThe available fields when setting an HTTP request header value using an expression are the followi...
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK