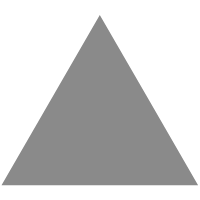
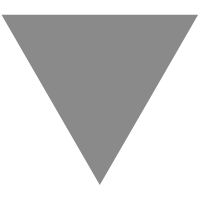
[Golang] Coin sums - Problem 31 - Project Euler
source link: http://siongui.github.io/2018/10/07/go-coin-sums-problem-31-project-euler/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
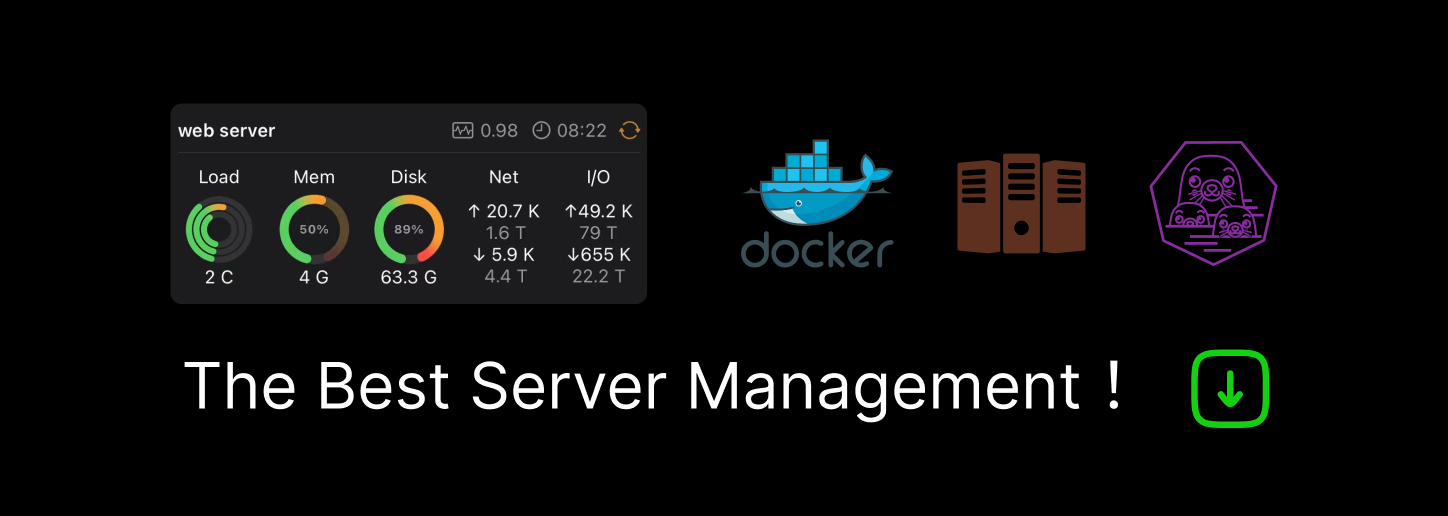
[Golang] Coin sums - Problem 31 - Project Euler
October 07, 2018
Problem: [1]
In England the currency is made up of pound, £, and pence, p, and there are eight coins in general circulation:
1p, 2p, 5p, 10p, 20p, 50p, £1 (100p) and £2 (200p).
It is possible to make £2 in the following way:
1×£1 + 1×50p + 2×20p + 1×5p + 1×2p + 3×1p
How many different ways can £2 be made using any number of coins?
Solution:
73682
My solution is ugly, which uses the brute-force method.
package main import ( "fmt" ) func main() { numberOfWays := 0 // loop for £2 (200p) for a := 0; a <= 1; a++ { sumA := a * 200 if sumA >= 200 { if sumA == 200 { fmt.Println("£2 = £2 *", a) numberOfWays++ } break } // loop for £1 (100p) for b := 0; b <= 2; b++ { sumB := sumA + b*100 if sumB >= 200 { if sumB == 200 { fmt.Println("£2 = £2 *", a, "+ £1 *", b) numberOfWays++ } break } // loop for 50p for c := 0; c <= 4; c++ { sumC := sumB + c*50 if sumC >= 200 { if sumC == 200 { fmt.Println("£2 = £2 *", a, "+ £1 *", b, "+ 50p *", c) numberOfWays++ } break } // loop for 20p for d := 0; d <= 10; d++ { sumD := sumC + d*20 if sumD >= 200 { if sumD == 200 { fmt.Println("£2 = £2 *", a, "+ £1 *", b, "+ 50p *", c, "+ 20p *", d) numberOfWays++ } break } // loop for 10p for e := 0; e <= 20; e++ { sumE := sumD + e*10 if sumE >= 200 { if sumE == 200 { fmt.Println("£2 = £2 *", a, "+ £1 *", b, "+ 50p *", c, "+ 20p *", d, "+ 10p *", e) numberOfWays++ } break } // loop for 5p for f := 0; f <= 40; f++ { sumF := sumE + f*5 if sumF >= 200 { if sumF == 200 { fmt.Println("£2 = £2 *", a, "+ £1 *", b, "+ 50p *", c, "+ 20p *", d, "+ 10p *", e, "+ 5p *", f) numberOfWays++ } break } // loop for 2p for g := 0; g <= 100; g++ { sumG := sumF + g*2 if sumG >= 200 { if sumG == 200 { fmt.Println("£2 = £2 *", a, "+ £1 *", b, "+ 50p *", c, "+ 20p *", d, "+ 10p *", e, "+ 5p *", f, "+ 2p *", g) numberOfWays++ } break } // loop for 1p for h := 0; h <= 200; h++ { sumH := sumG + h*1 if sumH >= 200 { if sumH == 200 { fmt.Println("£2 = £2 *", a, "+ £1 *", b, "+ 50p *", c, "+ 20p *", d, "+ 10p *", e, "+ 5p *", f, "+ 2p *", g, "+ 1p *", h) numberOfWays++ } break } } } } } } } } } fmt.Println("number of ways:", numberOfWays) }
Test on:
References:
Recommend
-
15
[Golang] Largest Prime Factor - Problem 3 - Project Euler May 17, 2017...
-
8
[Golang] Multiples of 3 and 5 - Problem 1 - Project Euler December 16, 2017...
-
11
[Golang] Largest Product in a Series - Problem 8 - Project Euler June 12,...
-
6
[Golang] Longest Collatz Sequence - Problem 14 - Project Euler June 10, 2017...
-
8
[Golang] Power digit sum - Problem 16 - Project Euler December 29, 2017
-
5
[Golang] Distinct powers - Problem 29 - Project Euler October 29, 2018
-
10
[Golang] Amicable Numbers - Problem 21 - Project Euler May 25, 2017 ...
-
8
[Golang] Counting Sundays - Problem 19 - Project Euler October 22, 2018...
-
8
[Golang] Lexicographic permutations - Problem 24 - Project Euler March 02,...
-
10
[Golang] Non-abundant sums - Problem 23 - Project Euler January 22, 2018...
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK