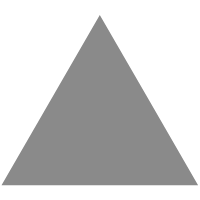
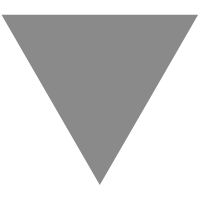
Choosing Between REST and GraphQL
source link: https://dzone.com/articles/choosing-between-rest-and-graphql
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Choosing Between REST and GraphQL
In this article, dive deep into the strengths and weaknesses of both REST and GraphQL so you can decide which API architecture is best for your next project.
Join the DZone community and get the full member experience.
Join For FreeThis is an article from DZone's 2022 Enterprise Application Integration Trend Report.
For more:
To create a data-driven web or mobile app, you'll need an API through which you can access data from your back-end server. The most popular architecture for APIs is REST. But while REST is widely known and generally easy to use, it has some downsides including overfetching and inefficiencies concerning nested data.
GraphQL is a new type of API architecture that is designed to be more flexible and efficient than REST with features like declarative data fetching. While GraphQL has become quite popular, it has not replaced REST as some users find it harder to use and consider it an overengineered solution, especially for smaller apps.
In this article, we'll be diving deeper into the strengths and weaknesses of both REST and GraphQL, so you can decide which API architecture is best for your next project.
The dominant architecture for APIs in modern app development is REST. The majority of back-end frameworks will implement REST, as do most public APIs including PayPal and Mailchimp. REST APIs are typically called using HTTP methods over a collection of URLs known as endpoints like GET /api/articles
or POST /api/articles
.
Example
Let's say you're creating a blog site. On the home page, you display summaries of the latest articles, including a title, image, and a short description. To provide data for this, you set up a REST endpoint on your back-end server, GET/api/articles
, which returns the required data as a JSON array, as in the example below:
// GET /articles
[
{
"id": 1,
"title": "REST is Awesome",
"image": "https://restblog.com/img/dsh9a89.png",
"description": "The benefits of REST"
},
{
"id": 2,
"title": "How REST Works",
"image": "https://restblog.com/img/33szad2.png",
"description": "Learn about REST"
}
]
Advantages of REST
REST has mostly outcompeted older API protocols like SOAP and continues to be popular despite newer alternatives like GraphQL. Let’s look at some of the reasons why.
Easy To Implement
REST is trivial to set up in a web server app, especially if it uses an API framework like Node’s Express or Python’s Requests. For example, setting up a REST endpoint for /articles
in an Express app with MongoDB can be as simple as calling the database and returning the records as JSON, as shown below:
app.get('/api/articles', async (req, res) => {
try {
const articles = await db.articles.find() res.json(articles)
} catch (err) {
res.status(500).send(err)
}
})
Widely Understood
Whether or not GraphQL is superior to REST, most engineers would agree that development is more productive when you use what you know. As of 2022, if you have multiple developers working on your app, or you have a public API, the majority of consumers will be familiar with REST. The same cannot yet be said of GraphQL.
Disadvantages of REST
To understand why GraphQL was created, we need to first look at the shortcomings of REST.
Overfetching
Returning to the example of the blog, let's say you created a mobile site, too. As with the desktop version, you display article summaries on the home page. As mobile has a smaller screen size, the summaries here only need the title and image and can leave out the description. Unfortunately, since the GET /api/articles
endpoint is fixed, the mobile version will still receive the description
field when it calls the API. This inefficiency is known as "overfetching" and becomes a problem when large amounts of data are being sent.
Inefficiency With Nested Data
An object has "nested data" when it contains sub-objects representing related entities. For example, you may have an article object with nested comment objects. Since entities are assigned their own unique endpoints in REST, nested data may need to be populated through separate roundtrips to the API.
For example, to get an article, we'd first use the GET /api/articles
endpoint. To get the comments on this article, we'd need to wait for the article data to populate first, so we knew which specific comments we need to fetch in subsequent requests, as in the code example below. Waiting for these subsequent requests to resolve will increase the time a user has to wait before they can interact with a page.
// GET /articles
[
{
"id": 1,
"title": "REST is Awesome",
"image": "https://restblog.com/img/dsh9a89.png",
"description": "An article about REST",
"comment_ids": [
10,
14,
22
]
},
{ ... }
]
GraphQL
Inefficiencies of REST, like the ones we've just looked at, prompted Facebook engineers to create a new API design in 2015 known as GraphQL. GraphQL quickly became a popular choice for developers and companies, launching an ecosystem of related tools and services. Like REST, GraphQL is not a specific piece of software, but rather a specification for an API design.
How GraphQL Works
In order to understand the advantages of GraphQL, we’ll do a quick overview of how it works. Unlike REST, GraphQL requires a schema that tells both the client and server what data and operations are allowed through the API. These are defined with the GraphQL schema language — a simple language-agnostic format featuring a strong type system.
Example
Let’s return to the example of a blog site with Article
and Comment
entities. In our GraphQL schema, we’d define the Article
type which, has a required integer id
field and optional string fields for title
, image
, and description
, like so:
type Article {
id: Integer!
title: String
image: String
description: String
}
In addition to basic scalar types, schema objects can reference each other. For example, we could create a one-to-many relationship between the Article
type and the Comment
type, as demonstrated below:
type Article {
id: Integer!
title: String
image: String
description: String
comments: [Comment]
}
type Comment {
content: String
article: Article
author: Author
}
Operations
Another important usage of the GraphQL schema is to define operations, which include both queries for reading data and mutations for writing data. Here, we've provided a query for Articles
, which is typed as an array of articles:
type Article {
id: Integer!
title: String
image: String
description: String
comments: [Comment]
}
type Comment {
content: String
article: Article
author: Author
}
type Query {
articles: [Article]
}
Advantages of GraphQL
With a basic understanding of GraphQL, we can now understand its main advantages.
Declarative Data Fetching
The killer feature of GraphQL is declarative data fetching where a client can specify exactly what data it needs. This can include specific fields and even within nested objects. We saw before that operations must be defined on the schema. Within these operations, though, we can specify which fields we want the query to return up to the limits of the schema.
For example, we could create a single query for getting Articles
with only the fields we want, with or without nested Comments
. See the example below:
query {
articles {
id
title
image
description
comments {
content
}
}
}
Here’s the data structure that would be returned from that query. Note that the data received in a GraphQL response will have the same shape as the query requesting it.
{
"data": {
"articles": [
{
"id": 1,
"title": "REST is Awesome",
"image": "https://restblog.com/img/dsh9a8.png",
"description": "An article about REST",
"comments": [
{
"content": "GraphQL is better!"
},
{ ... }
]
}
],
...
}
}
In this way, GraphQL eliminates both overfetching and the need for sequential calls for nested data.
Robustness
Thanks to the strong typing and the requirement for pre-defining queries, GraphQL can provide validation and type checking out of the box. In turn, this means that GraphQL is essentially self-documenting. Once a field, type, or query changes, documentation based on the schema can be automatically updated.
API Without Versioning
Every time an app changes, the API may also need to change. For example, let’s say we decided to rename the description
field in our Article
entity to blurb
. REST handles this by offering multiple versions — for example, /api/v1
and api/v2
— which can be cumbersome for both the API developer and consumers. With GraphQL, deprecated fields can be removed from the schema without impacting existing queries. This provides apps with continuous access to new features and encourages cleaner, more maintainable server code.
Disadvantages of GraphQL
While GraphQL provides an elegant solution to the downsides of REST, consider some of the criticisms that GraphQL has faced.
Overkill
Some developers argue that the problem GraphQL is solving is often overstated. For example, it probably doesn't really matter for most small apps if a few bytes of over-fetched data make it into a payload.
Harder to Work With
Another criticism is that GraphQL implementations end up being a lot harder to code than REST. It also provides a more difficult learning curve for new users.
Difficult to Cache
And finally, GraphQL is often criticized for being more difficult to cache. REST clients get the benefit of HTTP caching since all endpoints are URLs, while GraphQL clients need to implement their own custom solution.
Wrap Up
While the REST architecture has dominated web development over the past decade, its use of set endpoints makes it somewhat inflexible and inefficient. GraphQL solves these issues by providing a strictly typed schema language that consumers can query as required.
This is an article from DZone's 2022 Enterprise Application Integration Trend Report.
For more:
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK