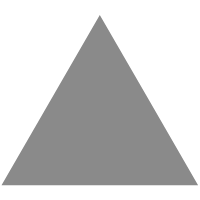
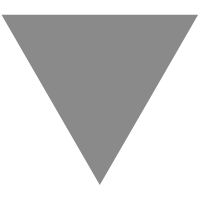
GitHub - Reiryoku-Technologies/Mida: A JavaScript framework for trading in globa...
source link: https://github.com/Reiryoku-Technologies/Mida
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
A JavaScript framework for trading in global financial markets
Introduction
Mida is a JavaScript framework for trading financial assets such as stocks, crypto, forex or commodities. It is designed to provide a solid and scalable environment for creating trading bots, indicators, market analysis tools or just trading applications depending on use cases.
Join the community on Discord and Telegram to get help you with your first steps.
Table of contents
Ecosystem
Project Status Description
A plugin for using cTrader accounts
A plugin providing technical analysis indicators
A library for getting real-time economic data
Supported brokers
Mida is broker-neutral, this means any broker could be easily integrated in
the ecosystem, until now all brokers available on the cTrader platform are supported,
below some of the most long-lived and popular ones,
exchanges are coming soon...
Installation
npm i @reiryoku/mida @reiryoku/mida-ctrader
Usage
Account login
How to login into a cTrader broker account.
const { Mida, } = require("@reiryoku/mida"); // Use the Mida cTrader plugin Mida.use(require("@reiryoku/mida-ctrader")); // Login into any cTrader account const myAccount = await Mida.login("cTrader", { clientId: "", clientSecret: "", accessToken: "", cTraderBrokerAccountId: "", });
To get a clientId
, clientSecret
and accessToken
you have to create an account on
cTrader Open API.
Balance, equity and margin
How to get the account balance, equity and margin.
console.log(await myAccount.getBalance()); console.log(await myAccount.getEquity()); console.log(await myAccount.getFreeMargin()); console.log(await myAccount.getUsedMargin());
Orders, deals and positions
How top open a long position for Bitcoin against USD.
const { MidaBrokerOrderDirection, } = require("@reiryoku/mida"); const myOrder = await myAccount.placeOrder({ symbol: "BTCUSD", direction: MidaBrokerOrderDirection.BUY, volume: 1, }); console.log(myOrder.id); console.log(myOrder.executionPrice); console.log(myOrder.position); console.log(myOrder.deals);
How to open a short position for EUR against USD.
const { MidaBrokerOrderDirection, } = require("@reiryoku/mida"); const myOrder = await myAccount.placeOrder({ symbol: "EURUSD", direction: MidaBrokerOrderDirection.SELL, volume: 0.1, }); console.log(myOrder.id); console.log(myOrder.executionPrice); console.log(myOrder.position); console.log(myOrder.deals);
How to open a short position for Apple stocks with error handler.
const { MidaBrokerOrderDirection, MidaBrokerOrderRejectionType, } = require("@reiryoku/mida"); const myOrder = await myAccount.placeOrder({ symbol: "#AAPL", direction: MidaBrokerOrderDirection.SELL, volume: 1, }); if (myOrder.isRejected) { switch (myOrder.rejectionType) { case MidaBrokerOrderRejectionType.MARKET_CLOSED: { console.log("#AAPL market is closed!"); break; } case MidaBrokerOrderRejectionType.NOT_ENOUGH_MONEY: { console.log("You don't have enough money in your account!"); break; } case MidaBrokerOrderRejectionType.INVALID_SYMBOL: { console.log("Your account doesn't support trading Apple stocks!"); break; } } }
More examples
Symbols and assets
How to retrieve all symbols available for your broker account.
const symbols = await myAccount.getSymbols(); console.log(symbols);
How to retrieve a symbol.
const symbol = await myAccount.getSymbol("#AAPL"); if (!symbol) { console.log("Apple stocks are not available for this account!"); } else { console.log(symbol.digits); console.log(symbol.leverage); console.log(symbol.baseAsset); console.log(symbol.quoteAsset); console.log(await symbol.isMarketOpen()); }
How to get the price of a symbol.
const symbol = await myAccount.getSymbol("BTCUSD"); const price = await symbol.getBid(); console.log(`Bitcoin price is ${price} US dollars`); // or console.log(await myAccount.getSymbolBid("BTCUSD"));
Ticks and candlesticks
How to listen the ticks of a symbol.
const { MidaMarketWatcher, } = require("@reiryoku/mida"); const marketWatcher = new MidaMarketWatcher({ brokerAccount: myAccount, }); await marketWatcher.watch("BTCUSD", { watchTicks: true, }); marketWatcher.on("tick", (event) => { const { tick, } = event.descriptor; console.log(`Bitcoin price is now ${tick.bid} US dollars`); });
How to get the candlesticks of a symbol (candlesticks and bars are generically called periods).
const { MidaTimeframe, } = require("@reiryoku/mida"); const periods = await myAccount.getSymbolPeriods("EURUSD", MidaTimeframe.M30); const lastPeriod = periods[periods.length - 1]; console.log("Last candlestick start time: " + lastPeriod.startTime); console.log("Last candlestick OHLC: " + lastPeriod.ohlc); console.log("Last candlestick close price: " + lastPeriod.close);
How to listen when candlesticks are closed.
const { MidaMarketWatcher, MidaTimeframe, } = require("@reiryoku/mida"); const marketWatcher = new MidaMarketWatcher({ brokerAccount: myAccount, }); await marketWatcher.watch("BTCUSD", { watchPeriods: true, timeframes: [ MidaTimeframe.M5, MidaTimeframe.H1, ], }); marketWatcher.on("period-close", (event) => { const { period, } = event.descriptor; switch (period.timeframe) { case MidaTimeframe.M5: { console.log(`M5 candlestick closed at ${period.close}`); break; } case MidaTimeframe.H1: { console.log(`H1 candlestick closed at ${period.close}`); break; } } });
Trading bots (expert advisors)
How to create a trading bot (or expert advisor).
const { MidaExpertAdvisor, MidaTimeframe, } = require("@reiryoku/mida"); class MyExpertAdvisor extends MidaExpertAdvisor { constructor ({ brokerAccount, }) { super({ brokerAccount, }); } async configure () { // Every expert advisor has an integrated market watcher await this.watchTicks("BTCUSD"); await this.watchPeriods("BTCUSD", MidaTimeframe.H1); } async onTick (tick) { // Implement your strategy } async onPeriodClose (period) { console.log(`H1 candlestick closed at ${period.open}`); } }
How to execute a trading bot.
const { Mida, } = require("@reiryoku/mida"); const { MyExpertAdvisor, } = require("./my-expert-advisor"); const myAccount = await Mida.login(/* ... */); const myAdvisor = new MyExpertAdvisor({ brokerAccount: myAccount, }); await myAdvisor.start();
Technical indicators
Install the plugin providing technical analysis indicators.
const { Mida, } = require("@reiryoku/mida"); // Use the Mida Tulipan plugin Mida.use(require("@reiryoku/mida-tulipan"));
How to calculate SMA (Simple Moving Average).
const { Mida, MidaTimeframe, } = require("@reiryoku/mida"); // Get latest candlesticks on H1 timeframe const candlesticks = await myAccount.getSymbolPeriods("EURUSD", MidaTimeframe.H1); const closePrices = candlesticks.map((candlestick) => candlestick.close); // Calculate RSI on close prices, pass values from oldest to newest const sma = await Mida.createIndicator("SMA").calculate(closePrices); // Values are from oldest to newest console.log(sma);
How to calculate RSI (Relative Strength Index).
const { Mida, MidaTimeframe, } = require("@reiryoku/mida"); // Get latest candlesticks on H1 timeframe const candlesticks = await myAccount.getSymbolPeriods("BTCUSD", MidaTimeframe.H1); const closePrices = candlesticks.map((candlestick) => candlestick.close); // Calculate RSI on close prices, pass values from oldest to newest const rsi = await Mida.createIndicator("RSI").calculate(closePrices); // Values are from oldest to newest console.log(rsi);
License and disclaimer
LICENSE
Trading in financial markets is highly speculative and carries a high level of risk.
It's possible to lose all your capital. This project may not be suitable for everyone,
you should ensure that you understand the risks involved. Mida and its contributors
are not responsible for any technical inconvenience that may lead to money loss, for example a stop loss not being set.
Contributors
Name Contribution GitHub Contact
Vasile Pește Author and maintainer Vasile-Peste [email protected]
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK