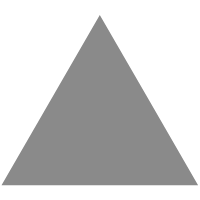
6
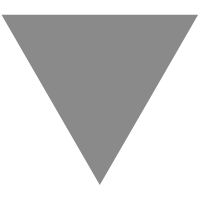
PHP GD图片处理[转换格式-水印-缩略图]
source link: https://blog.ixk.me/post/php-gd-image-processing
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
PHP GD图片处理[转换格式-水印-缩略图]
2019-02-14 • Otstar Lin •
本文最后更新于 268 天前,文中所描述的信息可能已发生改变
最近准备弄一个图床,既然是图床就需要能对图片进行一些常用的操作,比如水印,转换格式,生成缩略图等,前几天刚好接触了 PHP 的 GD 库,于是便花了点时间封装了这个简单的图片处理函数。
1/** 2 * 将图片转换为webp或其他格式,同时可以添加水印 3 * 4 * @author Otstar Lin 5 * 6 * @param String $source 原图片地址 7 * @param String $type 转换后图片格式 8 * @param String $to 转换后图片地址 若为 null 则代表直接输出到浏览器 9 * @param Array $thumbnail_size 缩略图尺寸 10 * @param Integer $thum 要输出到浏览器的图片,当 $to == null 的时候生效, -1 代表使用原尺寸图,其他数字分别代表使用 $thumbnail_size 指定索引的尺寸图 11 * @param Boolean $watermark 是否加水印 12 * @param Integer $watermark_type 水印格式 1:文字 2:图片 13 * @param String $watermark_content 水印内容,若为图片则是水印图片地址 14 * @param Integer $position 水印位置 15 * @param Integer $alpha 水印透明度 16 * @param Array $color 水印内容颜色 17 * @param Integer $font_size 水印文字大小 18 * @param String $font_name 水印字体地址 19 */ 20function image_change($source, $type='webp', $to = null, $thumbnail_size = [], $thum = -1, 21 $watermark = false, $watermark_type = 1, $watermark_content = '', $position = 9, 22 $alpha = 0, $color = [0, 0, 0], $font_size = 14, $font_name = 'Roboto-Medium.ttf') 23{ 24 $image = imagecreatefromstring(file_get_contents($source)); 25 // 生成水印 26 if($watermark) { 27 // 获取原图信息 28 $image_info = getimagesize($source); 29 // 设置颜色 30 $content_color = imagecolorallocatealpha($image, $color[0], $color[1], $color[2], $alpha); 31 // 载入字体 32 putenv('GDFONTPATH=' . realpath('.')); 33 $font = $font_name; 34 $left = 0; 35 $top = 0; 36 // 获取水印占据的空间大小 37 $watermark_content_info = []; 38 if($watermark_type == 1) { 39 $temp = imagettfbbox($font_size, 0, $font_name, $watermark_content); 40 $watermark_content_info[] = $temp[2] - $temp[0]; 41 $watermark_content_info[] = $temp[1] - $temp[7]; 42 } else { 43 $temp = getimagesize($watermark_content); 44 $watermark_content_info[] = $temp[0]; 45 $watermark_content_info[] = $temp[1]; 46 } 47 // 对水印进行定位 48 switch($position) { 49 case 1: 50 $left = 20; 51 $top = 20; 52 break; 53 case 2: 54 $left = $image_info[0]/2 - $watermark_content_info[0]/2; 55 $top = 20; 56 break; 57 case 3: 58 $left = $image_info[0] - $watermark_content_info[0] - 20; 59 $top = 20; 60 break; 61 case 4: 62 $left = 20; 63 $top = $image_info[1]/2 - $watermark_content_info[1]/2; 64 break; 65 case 5: 66 $left = $image_info[0]/2 - $watermark_content_info[0]/2; 67 $top = $image_info[1]/2 - $watermark_content_info[1]/2; 68 break; 69 case 6: 70 $left = $image_info[0] - $watermark_content_info[0] - 20; 71 $top = $image_info[1]/2 - $watermark_content_info[1]/2; 72 break; 73 case 7: 74 $left = 20; 75 $top = $image_info[1] - $watermark_content_info[1] - 20; 76 break; 77 case 8: 78 $left = $image_info[0]/2 - $watermark_content_info[0]/2; 79 $top = $image_info[1] - $watermark_content_info[1] - 20; 80 break; 81 case 9: 82 $left = $image_info[0] - $watermark_content_info[0] - 20; 83 $top = $image_info[1] - $watermark_content_info[1] - 20; 84 break; 85 } 86 // 对文字水印和图片水印进行不同的处理 87 if($watermark_type == 1) { 88 imagettftext($image, $font_size, 0, $left, $font_size + $top, $content_color, $font, $watermark_content); 89 } else { 90 $watermark_image = imagecreatefromstring(file_get_contents($watermark_content)); 91 imagecopy($image, $watermark_image, $left, $top, 0, 0, $watermark_content_info[0], $watermark_content_info[1]); 92 } 93 } 94 // 获取图片文件名(去除后缀) 95 $image_pathinfo = pathinfo($source); 96 $image_name = str_replace('.'.$image_pathinfo['extension'], '', $image_pathinfo['basename']); 97 $path = null; 98 // 输出原图 99 if($to === null) { 100 header('Content-type:image/'.$type); 101 } else { 102 $path = $to.$image_name.'.'.$type; 103 } 104 if($type == 'jepg') { 105 if($thum == -1) imagejpeg($image, $path); 106 } else if($type == 'png') { 107 if($thum == -1) imagepng($image, $path); 108 } else { 109 if($thum == -1) imagewebp($image, $path); 110 } 111 // 输出缩略图 112 foreach ($thumbnail_size as $num => $size) { 113 $set = true; 114 if(!isset($size['height'])) { 115 $set = false; 116 $size['height'] = $size['width'] * $image_info[1]/$image_info[0]; 117 } 118 $thum_img = imagecreatetruecolor($size['width'], $size['height']); 119 imagecopyresampled($thum_img, $image, 0, 0, 0, 0, $size['width'], $size['height'], $image_info[0], $image_info[1]); 120 if($to !== null) { 121 if($type == 'jepg') { 122 imagewebp($thum_img, $to.$image_name.'-'.$size['width'].'.'.$type); 123 } else if($type == 'png') { 124 imagewebp($thum_img, $to.$image_name.'-'.$size['width'].'.'.$type); 125 } else { 126 imagewebp($thum_img, $to.$image_name.'-'.$size['width'].'.'.$type); 127 } 128 } else { 129 if($num == $thum) { 130 if($type == 'jepg') { 131 imagewebp($thum_img); 132 } else if($type == 'png') { 133 imagewebp($thum_img); 134 } else { 135 imagewebp($thum_img); 136 } 137 } 138 } 139 } 140} 141// 缩略图的尺寸 142$thum = [ 143 [ 144 'width' => 200, 145 ], 146 [ 147 'width' => 400, 148 'height' => 200 149 ] 150]; 151image_change('img.png', 'webp', '', $thum, -1, true, 1, 'Otstar Lin', 9, 0);
PHP GD图片处理[转换格式-水印-缩略图]
Otstar Lin
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK