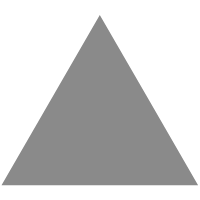
3
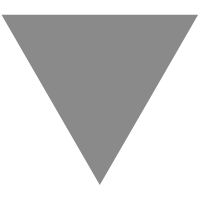
socket.io使用示例–向指定客户端发消息
source link: https://blog.p2hp.com/archives/8444
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
socket.io使用示例–向指定客户端发消息 | Lenix Blog
安装 node
创建一个目录例如socketio
在目录下执行
npm install socket.io
npm install redis
client: 代码如下:
<!doctype html> <html> <head> <meta charset="utf-8"> <title>socket.io</title> <meta name="keywords" content=""> <meta name="description" content=""> </head> <body> <script src="node_modules/socket.io/client-dist/socket.io.js"></script> <script> const socket = io("ws://localhost:3000"); socket.on("connect", () => { console.log(socket.id); // 输出客户端的id }); // send a message to the server socket.emit("hello from client", 5, "6", { 7: Uint8Array.from([8]) }); // receive a message from the server socket.on("hello from server", (...args) => { console.log(args) }); </script> </body> </html>
- <!doctype html>
- <html>
- <head>
- <meta charset="utf-8">
- <title>socket.io</title>
- <meta name="keywords" content="">
- <meta name="description" content="">
- </head>
- <body>
- <script src="node_modules/socket.io/client-dist/socket.io.js"></script>
- <script>
- const socket = io("ws://localhost:3000");
- socket.on("connect", () => {
- console.log(socket.id); // 输出客户端的id
- // send a message to the server
- socket.emit("hello from client", 5, "6", { 7: Uint8Array.from([8]) });
- // receive a message from the server
- socket.on("hello from server", (...args) => {
- console.log(args)
- </script>
- </body>
- </html>
<!doctype html> <html> <head> <meta charset="utf-8"> <title>socket.io</title> <meta name="keywords" content=""> <meta name="description" content=""> </head> <body> <script src="node_modules/socket.io/client-dist/socket.io.js"></script> <script> const socket = io("ws://localhost:3000"); socket.on("connect", () => { console.log(socket.id); // 输出客户端的id }); // send a message to the server socket.emit("hello from client", 5, "6", { 7: Uint8Array.from([8]) }); // receive a message from the server socket.on("hello from server", (...args) => { console.log(args) }); </script> </body> </html>
Server.mjs 代码如下: 使用 redis 存储客户端socket.id,参考https://socket.io/docs/v4/rooms/
import { createServer } from "http"; import { Server } from "socket.io"; import redis from 'redis'; const httpServer = createServer(); const io = new Server(httpServer, { cors: { origin: "*" } }); httpServer.listen(3000); var client = redis.createClient()//用于存储客户端socket.id /* createClient({ url: 'redis://alice:[email protected]:6380' });*/ await client.connect(); client.on("error", function (err) { console.log("Error " + err); }); io.on("connection", (socket) => { // send a message to the client console.log(socket.id);//打印客户端socket的id socket.emit("hello from server", 1, "2", { 3: Buffer.from([4]) }); // receive a message from the client socket.on("hello from client", async (...args) => { // Save the socket id to Redis so that all processes can access it. await client.set("mastersocket", socket.id); const value = await client.get('mastersocket'); console.log('ssssssssssss',value); setInterval(function(){ io.to(value).emit("hello from server", 1, value, { 3: Buffer.from([4]) });//向指定客户端发送消息 }, 3000); console.log('message: ' + args); }); });
- import { createServer } from "http";
- import { Server } from "socket.io";
- import redis from 'redis';
- const httpServer = createServer();
- const io = new Server(httpServer, {
- cors: {
- origin: "*"
- httpServer.listen(3000);
- var client = redis.createClient()//用于存储客户端socket.id
- createClient({
- url: 'redis://alice:[email protected]:6380'
- });*/
- await client.connect();
- client.on("error", function (err) {
- console.log("Error " + err);
- io.on("connection", (socket) => {
- // send a message to the client
- console.log(socket.id);//打印客户端socket的id
- socket.emit("hello from server", 1, "2", { 3: Buffer.from([4]) });
- // receive a message from the client
- socket.on("hello from client", async (...args) => {
- // Save the socket id to Redis so that all processes can access it.
- await client.set("mastersocket", socket.id);
- const value = await client.get('mastersocket');
- console.log('ssssssssssss',value);
- setInterval(function(){
- io.to(value).emit("hello from server", 1, value, { 3: Buffer.from([4]) });//向指定客户端发送消息
- }, 3000);
- console.log('message: ' + args);
import { createServer } from "http"; import { Server } from "socket.io"; import redis from 'redis'; const httpServer = createServer(); const io = new Server(httpServer, { cors: { origin: "*" } }); httpServer.listen(3000); var client = redis.createClient()//用于存储客户端socket.id /* createClient({ url: 'redis://alice:[email protected]:6380' });*/ await client.connect(); client.on("error", function (err) { console.log("Error " + err); }); io.on("connection", (socket) => { // send a message to the client console.log(socket.id);//打印客户端socket的id socket.emit("hello from server", 1, "2", { 3: Buffer.from([4]) }); // receive a message from the client socket.on("hello from client", async (...args) => { // Save the socket id to Redis so that all processes can access it. await client.set("mastersocket", socket.id); const value = await client.get('mastersocket'); console.log('ssssssssssss',value); setInterval(function(){ io.to(value).emit("hello from server", 1, value, { 3: Buffer.from([4]) });//向指定客户端发送消息 }, 3000); console.log('message: ' + args); }); });
运行node Server.mjs测试
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK