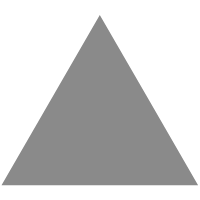
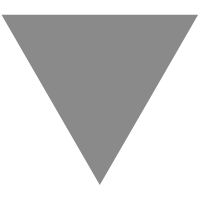
Kotlin Basics | Commissioning and Applications
source link: https://blog.birost.com/a?ID=00000-c5e9d86c-2020-4651-9cd4-00ff5966f68c
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Delegation is a common pattern, it has nothing to do with programming language, that is, delegate what you originally did to another object to do it. Both the decorator pattern and the agent pattern reuse behavior through delegation. Kotlin supports delegation at the language level. This article introduces Kotlin's delegation with examples.
Kotlin's decorator pattern
The decorator pattern and inheritance have the same purpose, both for extending the class, but it uses a more complicated way: inheritance + composition. The decorator pattern extends its functions on the basis of reusing the original types and behaviors. For a detailed analysis of the decorator mode and the agent mode, you can click to use the combined design mode | remote agent mode for chasing girls .
The following is an example of the decorator pattern:
This interface is used to describe an abstract accessory. A specific accessory needs to implement three methods to define the accessory name, price, and category.
Feathers, rings, and earrings are three specific accessories, and their realization is as follows:
class Feather : Accessory{ override fun name () : String = "Feather" override fun cost () : Int = 20 override fun type () : String = "body accessory" }
class Ring : Accessory{ override fun name () : String = "Ring" override fun cost () : Int = 30 override fun type () : String = "body accessory" }
class Earrings : Accessory{ override fun name () : String = "Earrings" override fun cost () : Int = 15 override fun type () : String = "body accessory" } Copy code
Now it is necessary to add feather rings and feather earrings. According to the inherited idea, this can be achieved as follows:
class FeatherRing : Accessory{ override fun name () : String = "FeatherRing" override fun cost () : Int = 35 override fun type () : String = "body accessory" }
class FeatherEarrings : Accessory{ override fun name () : String = "FeatherEarrings" override fun cost () : Int = 45 override fun type () : String = "body accessory" } Copy code
The disadvantage of writing this way is that only the type is reused, and there is no reuse behavior. Every time a new type is added, a subcategory must be added, which will cause subcategories to expand. If you switch to the decorator pattern, you can reduce one subcategory:
Now feather rings and earrings can be expressed like this
Using Kotlin's delegation syntax can be further simplified
Lazy initialization once
Lazy initialization is also a common pattern: the initialization of an object is delayed until it is accessed for the first time. When initialization consumes a lot of resources, lazy initialization is particularly valuable.
Supporting attributes is an idiomatic technique for lazy initialization:
Support attributes
So only when the first visit
The above code is a Kotlin predefined function
Keywords here
Appears after the attribute name, indicating attribute delegation , that is, entrusting the reading and writing of the attribute to another object. The delegated object must meet certain conditions:- When performing property delegation for read-only variables modified by val, the delegated object must implementgetValue()Interface, which defines how to get the variable value.
- When performing attribute delegation for var modified read-write variables, the delegated object must implementgetValue()withsetValue()Interface, which defines how to read and write variable values.
3.ways to achieve attribute delegation
public actual fun <T> lazy (initializer: () -> T ) : Lazy<T> = SynchronizedLazyImpl(initializer)
public interface Lazy < out T > { public val value: T public fun isInitialized () : Boolean } Copy code
In addition to extension functions, there are two other ways to implement the delegated class (assuming the type of proxy is String):
class Delegate { operator fun getValue (thisRef: Any ?, property: KProperty <*>) : String { return "Delegate" }
operator fun setValue (thisRef: Any ?, property: KProperty <*>, value: String ) { } } Copy code
In this way, a new proxy class is created, and keywords are passed in the class
The last way is as follows (assuming the type of proxy is String):
class Delegate : ReadWriteProperty < Any?, String > { override fun getValue (thisRef: Any ?, property: KProperty <*>) : String { return "Delegate" }
override fun setValue (thisRef: Any ?, property: KProperty <*>, value: String ) { } } Copy code
Realize
Then you can use the proxy class like this:
The implementation behind the attribute delegation is as follows:
Newly created
After delegation, when accessing the delegated properties, it is like calling a method of the proxy class:
Commissioned application
1. Easier access to parameters
Delegates can hide details, especially when the details are some template code:
class TestFragment : Fragment () { override fun onCreate (savedInstanceState: Bundle ?) { super .onCreate(savedInstanceState) val id = arguments?.getString( "id" ) ?: "" } }
class KotlinActivity : AppCompatActivity () { override fun onCreate (savedInstanceState: Bundle ?) { super .onCreate(savedInstanceState) val id = intent?.getStringExtra( "id" ) ?: "" } } Copy code
The code to get the value passed to the Activity or Fragment is very template. You can use delegates to hide the following details:
Then you can use the delegate like this:
class TestActivity : AppCompatActivity () { private val id by Extras( "id" , "0" ) }
class TestFragment : Fragment () { private val id by Extras( "id" , "0" ) } Copy code
2. Get the map value more easily
The properties of some classes are not fixed, but sometimes more, sometimes less, that is, dynamic, such as:
The above code can be simplified with delegation:
summary
- Kotlin delegation is divided into class delegation and attribute delegation . KeywordsTo commission.
- Class delegation can use a concise syntax to delegate the implementation of a class to another object to reduce template code.
- Property delegation can delegate access to properties to another object to reduce template code and hide access details.
- There are three ways to implement property delegation , namely extension method and implementationReadWritePropertyInterface, overloaded operator.
Recommended reading
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK