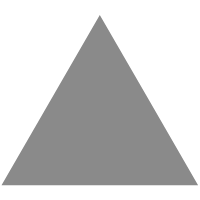
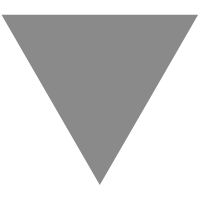
Rotate Images Using C++ [Easy Implementation]
source link: https://www.journaldev.com/60947/rotate-images-using-cpp
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Rotate Images Using C++ [Easy Implementation]
In this article, we will learn to rotate images by 90 degrees using C++. Top recruiters have asked about this problem many times during tech interviews. Not only this, but this particular problem also has considerable significance in our daily lives.
Almost every smartphone has this feature to allow users to rotate images. This feature is of great importance for photo editors and graphic designers. Today we will see how this feature works on the code side of things. What is the algorithm behind it? So without any delay, let’s go through the problem statement.
Problem Statement
Given a two-dimensional matrix of RGB values, rotate the matrix by 90 degrees in the anticlockwise direction.
All the images are simply two-dimensional matrices of RGB(Red, Green, and Blue) values. Rotation of an image implies that we have to rotate the RGB values stored in the image bitmap.
Note: In this article, we will only consider square matrices.
For example,
Input:
1 2 3 4
5 6 7 8
9 10 1112
13 14 15 16
Output:
4 8 12 16
3 7 11 15
2 6 10 14
1 5 9 13

Concept of Rotating Images using C++
There are multiple ways to solve this problem. For ease of understanding, we will only cover the simplest method. We are going to approach this problem without using any extra space. One way can be to map each element with its new position. But this can be quite complex to code. We will follow the steps given below to write the code.
Input:
1 2 3 4
5 6 7 8
9 10 1112
13 14 15 16
Output:
4 8 12 16
3 7 11 15
2 6 10 14
1 5 9 13
Did you notice any relation between the input and the output?
If you did, yo
u've nailed it. There'
s no need to worry
if
you haven't.
Step 1: Reverse the input matrix(row-wise)
1 2 3 4 --> 4 3 2 1
5 6 7 8 --> 8 7 6 5
9 10 1112 --> 12 11 10 9
13 14 15 16 --> 16 15 14 13
Now,
if
you notice carefully, you will find that the output matrix is just a transpose of
this
matrix.
Step 2: Take the transpose
4 3 2 1 --> 4 8 12 16
8 7 6 5 --> 3 7 11 15
12 11 10 9 --> 2 6 10 14
16 15 14 13 --> 1 5 9 13
Now, it’s time to write the code.
Algorithm for Rotating Images using C++
void
rotate_matrix(vector <vector <
int
>> &v)
{
int
n = v.size();
// first reverse the vector row-wise
// we can either use the pre-defined
// stl reverse function or we can use
// for loops
for
(
int
row = 0; row < n; row++)
{
int
start_col = 0;
int
end_col = n - 1;
while
(start_col < end_col)
{
swap(v[row][start_col], v[row][end_col]);
start_col++;
end_col--;
}
}
// now transpose the vector
for
(
int
i = 0; i < n; i++)
for
(
int
j = 0; j < n; j++)
if
(i < j)
swap(v[i][j], v[j][i]);
return
;
}

Implementing Image Rotation Using C++
#include <iostream>
#include <vector>
using
namespace
std;
void
rotate_matrix(vector <vector <
int
>> &v)
{
int
n = v.size();
// first reverse the vector row-wise
// we can either use the pre-defined
// stl reverse function or we can use
// for loops
for
(
int
row = 0; row < n; row++)
{
int
start_col = 0;
int
end_col = n - 1;
while
(start_col < end_col)
{
swap(v[row][start_col], v[row][end_col]);
start_col++;
end_col--;
}
}
// now transpose the vector
for
(
int
i = 0; i < n; i++)
for
(
int
j = 0; j < n; j++)
if
(i < j)
swap(v[i][j], v[j][i]);
return
;
}
void
print_matrix(vector <vector <
int
>> v)
{
cout <<
"The rotated matrix is:"
<< endl;
for
(
int
i = 0; i < v.size(); i++)
{
for
(
int
j = 0; j < v.size(); j++)
cout << v[i][j] <<
" "
;
cout << endl;
}
cout << endl;
}
int
main()
{
cout <<
"Enter the size of the vector"
<< endl;
int
n;
cin >> n;
vector <vector <
int
>> v(n, vector <
int
> (n, 0));
for
(
int
i = 0; i < n; i++)
{
for
(
int
j = 0; j < n; j++)
{
int
ele;
cin >> ele;
v[i][j] = ele;
}
}
rotate_matrix(v);
print_matrix(v);
return
0;
}
Output

Conclusion
In this article, we learned to rotate an image by 90 degrees in the anti-clockwise direction using C++. The concept is simple and just comprises two steps. In the end, we wrote the algorithm, and a driver program using C++. The output shows the example matrix being rotated three times. The final rotation angle of the output matrix is 270 degrees. That’s all for today, thanks for reading.
Further Readings
To learn more about vectors algorithms, you can also go through the following articles.
https://www.journaldev.com/60624/staircase-search-on-sorted-2d-matrices-cpp
https://www.journaldev.com/60799/spirally-read-2d-matrices-cpp
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK