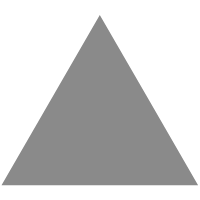
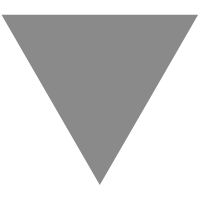
All you need to know about MultiThreading In Scala
source link: https://blog.knoldus.com/all-you-need-to-know-about-multithreading-in-scala/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
A process in which multiple threads execute simultaneously is called multithreading. It allows you to perform multiple tasks independently.
What are Threads in Scala?
Threads are lightweight sub-processes that occupy less memory. A multi-threaded program contains two or more threads that can run concurrently and each thread can handle a different task at the same time making optimal use of the available resources especially when your system(computer) has multiple CPUs. Multithreading is used to develop concurrent applications in Scala.
Threads in Scala can be created by using two mechanisms :
- Extending the Thread class
- Extending the Runnable Interface
Thread creation by extending the Thread class
We create a class that extends the Thread class. This class overrides the run() method available in the Threads class. Threads begin their life inside the run() method. We create an object of our new class and call the start() method to start the execution of threads. Start() invokes the run() method on the Threads object.
class MyThread extends Thread
{
override def run()
{
println("Thread " + Thread.currentThread().getName() +
" is running.")
}
}
object ExampleMultithreading
def main(args: Array[String])
{
for (x <- 1 to 5)
{
var th = new MyThread()
th.setName(x.toString())
th.start()
}
}
}
Output :
Thread 1 is running. Thread 2 is running. Thread 3 is running. Thread 4 is running. Thread 5 is running.
Thread creation by Extending Runnable Interface
We create a new class that extends the Runnable interface and override the run() method. Then we instantiate a Thread object passing the created class to the constructor. We then call the start() method on this object.
class MyThread extends Runnable
{
override def run()
{
println("Thread " + Thread.currentThread().getName() +
" is running.")
}
}
object MainObject
{
def main(args: Array[String])
{
for (x <- 1 to 5)
{
var th = new Thread(new MyThread())
th.setName(x.toString())
th.start()
}
}
}
Output :
Thread 1 is running. Thread 3 is running. Thread 4 is running. Thread 2 is running. Thread 5 is running.
Note: threads need not be running in any sequential order. All the threads run concurrently and independently of each other.
Scala Thread Life Cycle
In between the period of creation and Termination of Scala Threads, the threads undergo various state changes. These constitute the Life Cycle of a Scala Thread. It has the five following states.
- New: This is the first state when the Threads are just created.
- Runnable: This is the state when the Threads have been created but the Threads have not got the chance to start running.
- Running: In this state, the Threads is performing its task.
- Blocked (or Waiting): This is the state when the threads are still alive but are currently unable to run due to waiting for input or resources.
- Terminated: A thread is in a dead state when its run() method exits.
Conclusion: By Reading this Blog you will get the basic idea of how MultiThreading works in Scala
References:
https://www.geeksforgeeks.org/scala-multithreading/
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK