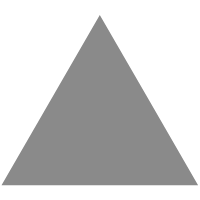
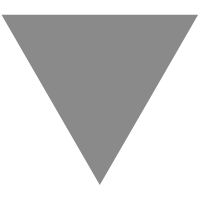
Lambda Expression and functional Interface
source link: https://blog.knoldus.com/lambda-expression-and-functional-interface/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Reading Time: 2 minutes
1. Lambda Expressions
Lambda Expressions were added in Java 8. A lambda expression is a short block of code which takes in parameters and returns a value. Lambda expressions are similar to methods, but they do not need a name and they can be implemented right in the body of a method.
1.1. Syntax
A few basic syntaxes of lambda expressions are:
(parameters) -> expression
(parameters) -> { statements; }
() -> expression
A typical lambda expression example will be like this:
//This function takes two parameters and return their sum
(x, y) -> x + y
Please note that based on the type of x
and y
, we may use the method in multiple places. Parameters can match to int
, or Integer
or simply String
also. Based on context, it will either add two integers or concatenate two strings.
Here demonstration of lambda expression with two arguments.
public class Test { interface FuncInter1 { int operation(int a, int b); } interface FuncInter2 { void sayMessage(String message); } private int operate(int a, int b, FuncInter1 fobj) { return fobj.operation(a, b); } public static void main(String args[]) { FuncInter1 add = (int x, int y) -> x + y; FuncInter1 multiply = (int x, int y) -> x * y; Test tobj = new Test(); System.out.println("Addition is " + tobj.operate(5, 2, add)); System.out.println("Multiplication is " + tobj.operate(5, 2, multiply)); FuncInter2 fobj = message ->System.out.println("Hello " + message); fobj.sayMessage("LambdaExpression"); } }
1.2. Rules for writing lambda expressions
- A lambda expression can have zero, one or more parameters.
- The body of the lambda expression can be either a single expression or more statements.
- The type of the parameters can be explicitly declared if it can be inferred from the context.
- The body of the lambda expressions can contain zero, one, or more statements.
- If the body of lambda expression has a single statement, curly brackets are not mandatory and the return type of the anonymous function is the same as that of the body expression.
2. Functional Interfaces
Functional interfaces are also Single Abstract Method interfaces. As the name suggests, a functional interface permits exactly one abstract method in it.
Java 8 introduces @FunctionalInterface
annotation that we can use for giving compile-time errors it a functional interface violates the contracts.
2.1. Functional Interface Example
//Optional annotation
@FunctionalInterface
public interface MyFirstFunctionalInterface {
public void firstWork();
}
Please note that a functional interface is valid even if the @FunctionalInterface
annotation is omitted. It is only for informing the compiler to enforce a single abstract method inside the interface.
Also, since default methods are not abstract , we can add default methods to the functional interface as many as we need.
Another critical point to remember is that if a functional interface overrides one of the public methods of java.lang.Object, that also does not count toward the interface’s abstract method count since any implementation of the interface will have an implementation from java.lang.Object or elsewhere.
For example, given below is a perfectly valid functional interface.
@FunctionalInterface
public interface MyFirstFunctionalInterface
{
public void firstWork();
@Override
public String toString(); //Overridden from Object class
@Override
public boolean equals(Object obj); //Overridden from Object class
}
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK