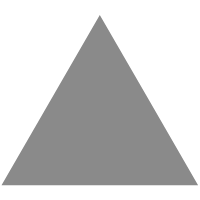
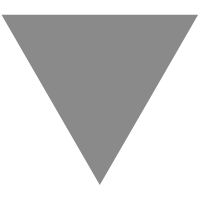
Android development: combining two Java files
source link: https://www.codesd.com/item/android-development-combining-two-java-files.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Android development: combining two Java files
I have two different java files and was wondering if there was a way to combine them so that both codes were in one file.
The below simply creates a dialog box that does nothing when no is clicked and moves to a new activity if yes is clicked:
package com.example.top_tech_deals;
import android.app.Activity;
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.Toast;
public class AlertDialogActivity extends Activity {
/** Called when the activity is first created. */
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.post);
Button btnAlertTwoBtns = (Button) findViewById(R.id.btnAlertWithTwoBtns);
/**
* Showing Alert Dialog with Two Buttons one Positive Button with Label
* "YES" one Negative Button with Label "NO"
*/
btnAlertTwoBtns.setOnClickListener(new View.OnClickListener() {
public void onClick(View arg0) {
// Creating alert Dialog with two Buttons
AlertDialog.Builder alertDialog = new AlertDialog.Builder(AlertDialogActivity.this);
// Setting Dialog Title
alertDialog.setTitle("Confirm Delete...");
// Setting Dialog Message
alertDialog.setMessage("Are you sure you want delete this?");
// Setting Icon to Dialog
alertDialog.setIcon(R.drawable.delete);
// Setting Positive "Yes" Button
alertDialog.setPositiveButton("YES",
new DialogInterface.OnClickListener() {
public void onClick (DialogInterface dialog,int which) {
// Write your code here to execute after dialog
Intent k = new Intent(AlertDialogActivity.this, Camera.class);
startActivity(k);
Toast.makeText (getApplicationContext(), "You clicked on YES", Toast.LENGTH_SHORT).show();
}
});
// Setting Negative "NO" Button
alertDialog.setNegativeButton("NO",
new DialogInterface.OnClickListener() {
public void onClick (DialogInterface dialog, int which) {
// Write your code here to execute after dialog
Toast.makeText (getApplicationContext(), "You clicked on NO", Toast.LENGTH_SHORT).show();
dialog.cancel();
}
});
// Showing Alert Message
alertDialog.show();
}
});
}
}
This second code creates a drop down menu full of items the user can select:
package com.example.top_tech_deals;
import java.util.ArrayList;
import java.util.List;
import android.app.Activity;
import android.os.Bundle;
import android.view.View;
import android.widget.AdapterView;
import android.widget.ArrayAdapter;
import android.widget.Spinner;
import android.widget.Toast;
import android.widget.AdapterView.OnItemSelectedListener;
public class AndroidSpinnerExampleActivity extends Activity implements OnItemSelectedListener{
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.spinex);
// Spinner element
Spinner spinner = (Spinner) findViewById(R.id.spinner);
// Spinner click listener
spinner.setOnItemSelectedListener(this);
// Spinner Drop down elements
List<String> categories = new ArrayList<String>();
categories.add("Automobile");
categories.add("Business Services");
categories.add("Computers");
categories.add("Education");
categories.add("Personal");
categories.add("Travel");
// Creating adapter for spinner
ArrayAdapter<String> dataAdapter = new ArrayAdapter<String>(this, android.R.layout.simple_spinner_item, categories);
// Drop down layout style - list view with radio button
dataAdapter.setDropDownViewResource(android.R.layout.simple_spinner_dropdown_item);
// attaching data adapter to spinner
spinner.setAdapter(dataAdapter);
}
@Override
public void onItemSelected(AdapterView<?> parent, View view, int position, long id) {
// On selecting a spinner item
String item = parent.getItemAtPosition(position).toString();
// Showing selected spinner item
Toast.makeText(parent.getContext(), "Selected: " + item, Toast.LENGTH_LONG).show ();
}
public void onNothingSelected(AdapterView<?> arg0) {
// TODO Auto-generated method stub
}
}
Essentially what I want is one of my xml files to use both a dialog box and a drop down menu, however because I was having trouble putting both these codes into one java file I resorted to creating two different xml files, post.xml which is linked to AlertDialogActivity and spinex.xml which is linked to AndroidSpinnerExampleActivity. I tried linking the two java files to the same xml but I found that only one of the java files seemed to do anything, so I believe the only solution would be combining the two codes into one java file, but I always seem to get errors when I try this.
Each public class must be in a separate file, with filename the same as the class. But if you change one of your classes to be not public, then OK. I think best practice is to keep your files as they are. It's way better for future maintainability to have your classes in separate files, so that you can easily find your classes.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK