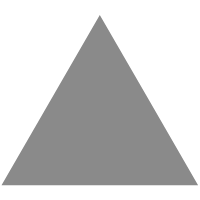
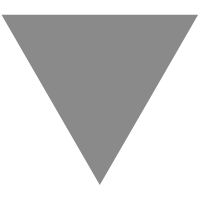
Memorizing a programming language using spaced repetition software
source link: https://sive.rs/srs
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
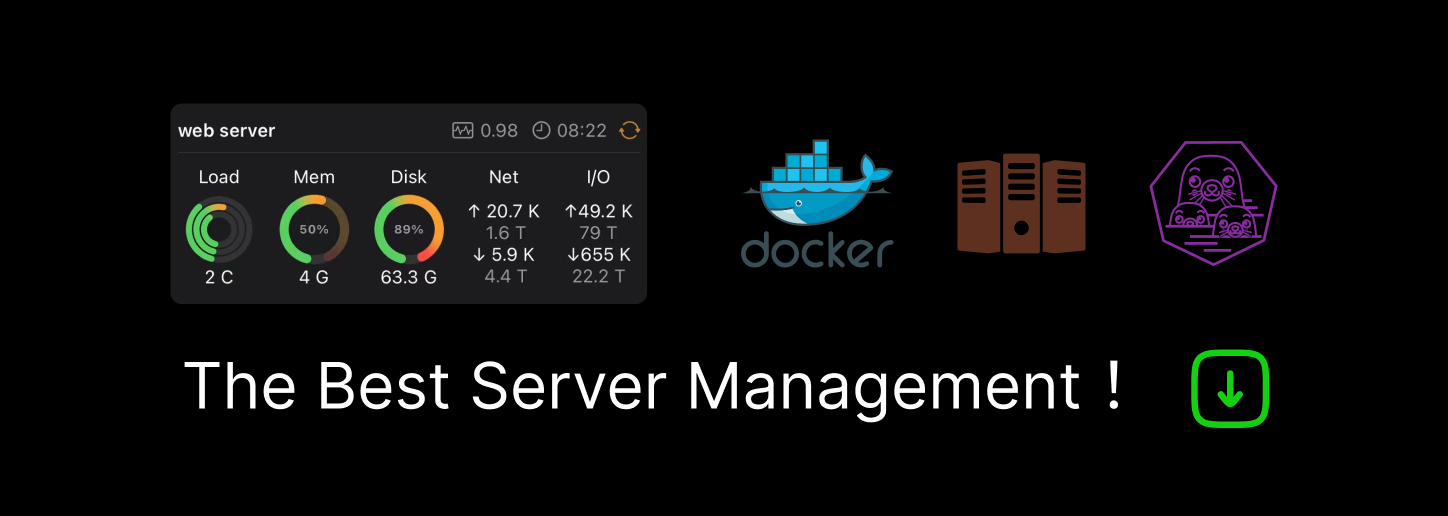
Memorizing a programming language using spaced repetition software
2013-01-06
I’ve been doing this for a year, and it’s the most helpful learning technique I’ve found in 14 years of computer programming.
Background:
I’m an intermediate programmer. I didn’t go to school for it. I just learned by necessity because I started a website that just kept growing and growing, and I couldn’t afford to hire a programmer, so I picked up a few books on PHP, SQL, Linux, and Apache, learned just enough to make it work, then used that little knowledge for years.
But later, when I worked along side a real programmer, I was blown away by his vocabulary! All of these commands and functions just flowing effortlessly out of his fingers. We were using the same language, but he had memorized so much of it, that I felt like a child next to a university professor. I really wanted to get that kind of fluency.
It made me think about how much I’ve learned then immediately forgotten, over the years. I read books or articles about some useful feature, try it once, but then I get distracted, forget about it, and go about my normal way of doing things.
I wanted to deeply memorize the commands and techniques of the language, and not forget them, so that they stay at the forefront of my mind whenever I need them.
Spaced Repetition:
When you hear a new fact, it’s forgotten pretty quickly unless it’s brought back to the forefront of your mind repeatedly.
You can do this haphazardly by immersing yourself in a language, for example, where the new words you learn will be brought up by chance occasionally.
But memory research shows that the most effective and efficient time for a new fact to be remembered is right before you were about to forget it.
Say if you learn a new word in a foreign language, you’d want to practice it again a few minutes after hearing it, then a few hours, then the next day, then in 2 days, then 5 days, then 10 days, 3 weeks, 6 weeks, 3 months, 8 months, etc. After a while it’s basically permanently memorized with a rare reminder.
Spaced Repetition Software does this for you, so you can just give it a bunch of facts you want to remember, then have it quiz you once a day, and it manages the intervals based on your feedback. After each quiz question, if you say that one was easy, it won’t be introduced for a long time, but if you were stumped, then it’ll ask it again in a few minutes, until you’ve got it.
Go to apps.ankiweb.net and download Anki. It’s a free, open source, popular spaced repetition software program.
As for programming, you get where I’m going with this.
What if you could memorize everything about the programming language of your choice? Every command, every parameter, every function. Every solution to hundreds of the most common problems, all completely memorized at your fingertips? Imagine going through the documentation or a book, and permanently remembering every single thing in it?
Enough of the intro, let’s get to the HOW-TO:
First, learn!
Flash cards are for remembering what you’ve learned.
Before you create a flash card for something, you need to actually learn and understand it. Create the flash card after you’ve really understood.
(This is why it’s not that helpful to look at someone else’s deck. Those are just reminders.)
Convert Knowledge into Small Facts:
You’re going to be making a bunch of flash cards. Question on the front. Answer on the back.
If you were just using this to memorize foreign language vocabulary, then the formatting would be easy. The front would have a word or phrase, and the back would have its translation, and vice-versa.
But if you’re learning anything else, you’re going to have to put a little craft and creativity into making your own flash cards.
It takes some effort to read through paragraphs of stuff you want to remember, pick out the key facts, break them down into their smallest form, and turn them into questions for quizzing your future self.
Here are my best time-saving tips from a year of doing this:
Turn prose into code
If you’re reading a tutorial about programming, and come across a paragraph describing a feature.
“The add (+) operator... if only one operand is a string, the other operand is converted to a string and the result is the concatenation of the two strings.”
You test it out yourself, play around with it, and understand it. So you make a flashcard to remember it.
var a = 5 + '5'; // what is a?
'55' If either side of + is a string, the other is converted to a string before adding like strings.
Try to trick your future self
Sometimes you learn a “gotcha” — a common mistake or surprising feature.
“If the new Array constructor is passed a single number, it creates an empty Array with a length of that number. Any other combination of arguments creates an Array of those arguments.”
You test it out yourself, play around with it, and understand it. Then make two flash cards to try to trick your future self.
var a = new Array('5'); // what is a?
An array with one item, the string '5': ['5'];... and then an almost-identical question ...
var a = new Array(5); // what is a?
An empty array with a length of 5.
When the program quizzes you, it will shuffle the cards, so that hopefully your examples will intentionally catch you by surprise.
You can also try to trick yourself with more complicated examples, to keep these gotchas fresh in your mind:
var a = [20, 10, 5, 1]; // what is a.sort()?
[1, 10, 20, 5] // sort treats all values as strings
Don’t forget to quiz yourself on the solution, too:
var a = [20, 10, 5, 1]; // sort these in numeric order
function compare(v1, v2) { return(v1 — v2); } a.sort(compare);
Save the cool tricks
If you find a cool trick you want to remember, turn it into the answer of a small challenge.
var albums = [ {name: 'Beatles', title: 'White Album', price: 15}, {name: 'Zeppelin', title: 'II', price: 7}]; // make this work: albums.sort(by('name')); albums.sort(by('title')); albums.sort(by('price'));
function by(propName) { return function(obj1, obj2) { v1 = obj1[propName]; v2 = obj2[propName]; if (v1 < v2) { return -1; } else if (v1 > v2) { return 1; } else { return 0; } }; }
Make the answer require multiple solutions
If there’s more than one way of doing something, and you want to remember both, make your future self come up with more than one solution, so you can keep both alternatives in mind.
s = 'string like this' # In Ruby, show two ways to turn it into 'String Like This'
s.split.map(&:capitalize).join(' ') s.gsub(/\b\S/) {|x| x.upcase}
Turn broad concepts into succinct examples
Say you just spent 20 minutes learning something that’s more conceptual, and not as much about remembering specific functions. Sometimes all you need is one succinct example to remind yourself of the concept.
/(a(b)((c)d))/.match('abcd') # What will $1, $2, $3, $4 be?
$1 = 'abcd' $2 = 'b' $3 = 'cd' $4 = 'c'Another example:
class C self end class D < C end d1 = D.new # which object is self?
class D
Read “20 Rules of Formulating Knowledge” by Piotr Wozniak
The best advice on this stuff is an article called “20 Rules of Formulating Knowledge” by Piotr Wozniak at supermemo.com/en/articles/20rules. So please read that one.
Run Through it Daily
For most efficient results, turn on your spaced repetition software once a day. If you go too long without, you’ll screw up all the timings, and have to re-learn stuff you would have remembered.
You can remember thousands of these facts in only 20 minutes a day. I just make it a morning routine. Make a cup of boiling tea. Do my Anki. Drink my tea.
It’s fun when quizzing yourself to add a little adrenaline, and make yourself go as fast as you can.
It’s like a mental visit to the gym. A little intense 20 minutes a day is so worth it for the immediate and long-term results.
Add some new cards whenever you can, and you’ll be amazed that everything you saved stays fresh in your mind.
Conclusion
I’ve been doing this for a year, and it’s been a HUGE boost to my fluency. I highly recommend it, as you can tell.
Feel free to email me anytime to let me know how it goes for you.
Read more:
The “Janki Method Refined” by Jack Kinsella is a great article on similar approach,and explains it even better than I did here.
You can also use Anki to learn all kinds of things.
“Augmenting Long-term Memory” by Michael Nielsen is an amazing article about using Anki for learning anything.
Read the interview with Piotr Wozniak at wired.com for a story about a guy taking this to the limit.
© 2013 Derek Sivers. ( « previous || next » )
Copy & share: sive.rs/srs
Comments
- Victor Hou (2013-01-06) #
Awesome post and really hits the spot, because I know I feel the same way and know a lot of people also feel the same way. Just like any other things in the world, some people can learn it easily and it's more difficult for some, everyone remembers things differently in different ways, but as any startup nowadays it's so critical to at least have an idea of basic coding, if not at least the language and reasons behind it.
I've been reading your blogs and watching the videos of your speeches, what intrigues me and look up to you is you are a leader, and have a passion for not only changing the world but people. Inspiring people to believe and creating products that help people achieve a piece of their dreams. Those are all the same principles and inspiration I hope to build with www.trendstartr.com
Thank you for your advice on the move to Singapore in a previous email, I know you are really busy and now focused on your own startup, but look forward to staying in touch via email and hopefully get your feedback on what we are building with TrendStartr - Gloria Hylton (2013-01-06) #
good info...could of used this when learning italian before my trip to italy...i remember hardly any of it these days ;~)
- Dan Nuffer (2013-01-06) #
I do the same thing. I started just using anki cards, but found that using the normal anki cards to be less effective because thinking the answer to the card and programming are different contexts, making it harder to apply the anki knowledge, so I make a modification and now use anki to remind me to do actual programming tasks. I have a git repo with various exercises. https://github.com/dnuffer/dpcode
In the anki deck I record how long it took me to do each exercise and then when I re-do it I compare the times and use my improvement or lack thereof to determine the next interval.
Very interesting twist! Thanks for the comment. — Derek - rada neal (2013-01-06) #
I think it's the same idea in memorizing music or specific arrangements. After learning the Bach Italian Concerto and continually studying and disecting the piece I can learn anything. Problem is I prefer to use those brain cells for music. I'm a pianist who doesn't know the lettering on a computer keyboard....though I use more than 2 fingers....and I believe I mispelled a word above....maybe two......btw...I have 3 SS #'s memorized besides countless other bank and card #'s. You learn what's important to you.
rada - Terry Dossey (2013-01-06) #
Or, just (a) study the programming language in college (where they teach you most of the frequently used functions, syntax and algorithms), then (b) do it day in and day out for a living. You'll remember everything you use, no need for memory cards.
Once you've worked for a few years as a programmer, you'll have assembled a pretty large library of code you've written. Provided you've commented it well and organized it for quick access, you're golden. Most programming is recombining / adapting / extending code you've written before. Once in a while you need to open the manual.
But you're right, it's important to start with a large vocabulary in the (programming) language. You can't remember what you never knew. That's how your professional programmer friend did it, I'm guessing.
One thing I used to do for at least half an hour each day when I worked as a programmer, then systems analyst, then senior systems analyst was to open the (huge) manuals at random to some function, subroutine, or syntax I knew nothing about, then write a very short program using it to add to my library. - Sean Dillman (2013-01-06) #
Not personally that big a believer in memorization: didn't really feel the need in years of programming (more important in c++, java's so well-documented). Likewise didn't use flashcards or other memorization techniques in grad school, still finished near 4.0.
Would definitely like to try this with natural language(s), as others have noted. - Ian Clay (2013-01-06) #
Hi Derek,
Thanks for the tip.
I'm currently learning Objective C, Python and Javascript. Over the next 2 years I wont be doing much else :) - Anne Sete (2013-01-06) #
OMG! This is amazing! I'm going to jump on board with this one! THANK YOU!
- Adam Smith (2013-01-06) #
Wow! Excellent stuff, this should be shown to Elementary school kids. Thanks for the links and overview.:-)
- gwern (2013-01-06) #
Like every good idea, it's been invented by many people.
Wozniak suggested spaced repetition for programming in 1993: http://www.supermemo.com/articles/programming.htm
I began using it for occasional programing bits back in, dunno, 2009 or so.
Jack Kinsella wrote about his uses in 2011: http://www.jackkinsella.ie/2011/12/05/janki-method.html
I'm sure this list is far from complete.
Awesome! I can't believe I never noticed that Wozniak article before. Thank you for posting the link! — Derek - Amandah Jantzen (2013-01-06) #
I used Anki to learn Japanese Hiragana symbols--it worked great--after not being exposed to them for over 6 months, I can still recognize and name the various characters.
- Jason Sebring (2013-01-06) #
Pimsleur is just that.
http://www.pimsleur.com/The-Pimsleur-Method
Yep! And very natural at it. I really like Pimsleur. — Derek - Bill Davis (2013-01-06) #
Interesting, Derek!
I've spent decades as a language learning consultant (real human language), so reading this got me to thinking. I don't know any programming languages, although I used to write pretty complex macros in WordPerfect years and years ago. *smile*
It seems to me that what you're talking about here is a hybrid kind of learning. There is vocabulary and syntax, as in any language, but the programmer is also learning new definitions for English words (e.g. I have no idea what the English examples which speak of "arrays" are talking about!). Plus, there are other conceptual layers to be learned. In other words, first the programmer needs to know what they can/need do, then how to "say" a command in one of a variety of langue ages to make it happen. The conceptual part is primary, and doesn't seem like it would to all be relearned to learn a second or third programming language, which many people do.
Now, on to language learning. Spaced Repetition is indeed a valid principle for learning and memorizing, and even for learning language, but the mistake is to think at language is learned simply by memorization. It isn't.
There would be more effective ways to "review" programming commands, and to use Spaced Repetition than to use flash cards (paper or electronic). These other, situationally-based, interactive and cognitive activities would produce even better (and more long-lasting) results/learning, and would take advantage of other, equally valid principles of learning, such as the Iceberg Principle.
Anyway, you've got my mind running on how it would be to apply those language learning principles to a very different kind of language (programming)! - T Scott Walker (2013-01-06) #
Thank-you Derek - your information is always tops- I'm working on CDL and realestate licenses at the same time- plus I do music shows,host a radio show and promote ad sales for Everything Lake Magazine- I can sure use some memorization help- tks again- T Scott
- Oswald (2013-01-06) #
This is great! Them having a mobile application makes it even better. Learning on the go. Can be used to inculcate whatever new habits too =D
- Renee Frances Conn (2013-01-06) #
Oh, Derek!
This post made me so happy. As soon as I saw your name in my inbox and opened it to these words,
"New article I think you might like:
Memorizing a programing language using spaced repetition software
I'd love to hear your thoughts, so feel free to leave a reply there on the site. Also feel free to copy, forward, quote, or anything else."
These three lines - hold great heft
Respectful request to audience
Derek, you are a cosummate teacher. Laser sharp focus, truly in keeping with your minimalistic style. You walk your talk and freely share what you learn. That is really cool and punk!
Happy Merry Festivas and Happy New Year to you and yours.
For the past few years, you have been a kind nundging teacher to me and and dare I say friend.
I'm excited to sink my teeth into this article.
:-)Renee - paduraru (2013-01-06) #
Spaced repetition boost fluency for sure!
- Jeff Marx (2013-01-06) #
NERD!!!!!!!!!!!!!!!!!!!!!
- Nikhil Gopal (2013-01-06) #
As someone who is multilingual, not just in world languages but computer languages, I think the real key to learning any language is immersion. SRS seems to be a method that exploits this tactic to a degree. Pretty cool stuff.
- Jean-Baptiste Collinet (2013-01-06) #
Great!
That's an amazing concept. I'll test it today already. Wow, the day starts marvelously!
I have some rather unpleasant health issues related to epilepsy, but without seizures. It's like if my brain is a harddrive formatting itself from time to time thus wiping all the content. Cannot tell you how frustrating it is when you work in OOP languages... Sounds useful for music too. Will put my students to it :)
I have the feeling that those cards can do wonders for me.
Thank you Derek!
JB - charlie (2013-01-06) #
a huge thanks
a bit adhd
gonna wanna try it - Lee (2013-01-06) #
Anki is amazing. I found it six months ago when I was studying for my General Class ham radio licence. It made such an impressive difference in my retention of material. In addition to the spaced repetition, I loved how it automatically would only give me twenty new cards to remember each day. Looking at a stack of 500 cards is overwhelming, but a bite of 20 per day, along with the reviews from the previous learned material was so completely doable. I've been using it since to memorize other material for my work. I need to take the next step and incorporate the mobile version into my routine so I can take advantage of those little odd bits of down time throughout the day.
- Adam Cole (2013-01-06) #
Thanks, Derek,
I'll try this with Spanish vocab and Bebop licks!
Love,
Adam - Martin (2013-01-06) #
Fantastic! I will use this on my third attempt to learn Chinese :)
- Chip Mounger (2013-01-06) #
I think I need a Red Bull.
- Amanda (2013-01-06) #
Wow. I love the concept of the timing of repetition affecting memorization. Reading that code made me realize how ignorant I am about that language. Truly amazing to see such a foreign tongue in plain English.
- A.J. Palluck (2013-01-06) #
Fantastic breakdown of something that I think we all intrinsically know: To master something (from the standpoint of memorization) requires structure. I will definitely check out that software. Thanks again!
- Lee Kim (2013-01-06) #
That is a very fascinating chart to see the spacial relationship of time and retention.
I am sure just the act of thinking of the question to be on the card ingrains the info a little deeper.
I will definitely be using this system.
Thanks for the great tool Derek! - Jake (2013-01-06) #
It made me think about how much I've learned then immediately forgotten, over the years. I read books or articles about some useful feature, and try it once, but then I get distracted, forget about it, and go about my normal way of doing things.
Depending on what you're trying to remember and why, Steven Berlin Johnson's article about how he uses Devonthink Pro might be more useful. He describes how he relies on software to come up with novel connections between disparate pieces of written text. I've been using the same system for years and found it very useful. Friends and readers occasionally ask me how I "know" such weird stuff.
Much of the time, I don't—at least, I don't in the way they think I do.
As far as I know, there are similar programs for Windows and Linux. Evernote is commonly mentioned, though I've never used it.
Very interesting! Thanks for the link. — Derek - Hank (2013-01-06) #
Which was the book you bought for start?i wanna prove php and i want to do the same with english.i ll try this method.thanks for sharing.thanks for inspire us!
- Jonathan (2013-01-06) #
I'll have to read through this article again but this looks awesome. Ironically I was using this same technique a while back when I was making flash cards to learn Hiragana when I was living in Japan. I would concentrate on a small set of Hiragana characters and continually go through them until I knew them all like the back of my hand. If there was a few I didn't know I'd set them aside and then go through that stack until I knew them all. I did this until I knew all the characters and it worked. I really want to learn Javascript and while I'm not particularly fond of programming I know it's needed for some of the things I want to do (namely web design and game design). Great article Derek! I too am learning things as I go for my businesses.
- Jackie Britton Lopatin (2013-01-06) #
Oh my. Flashcards I get; programming language? WING!!! Right over my head.
I'll try coming back to this and see if any of it sinks in, but boy, is this esoteric! - John Walsh (2013-01-06) #
Derek, thank you! I get the concept, the coding not so much. The big takeaway is that you just gave me a big box of ideas. Your generosity is always inspiring!
- Scott (2013-01-06) #
Awesome. Thanks for the tips!
- Aaron Yoshitake (2013-01-06) #
Thanks for the SRS tips, Derek. I've been using Anki for a while but I tend to forget to open it up. Your tea-and-Anki morning routine sounds like a winner; I'll give it a shot.
Also, any chance you have Anki decks you recommend, or which you'd like to share? I'd love to quiz myself with JavaScript cards like the ones you've described here (and those which other commenters have described - jQuery API flashcards, anyone?). Collaboratively building Anki decks would also help lessen the time spent creating flashcards. - jeremy l. mix (2013-01-06) #
So many places to apply this in life. Repetition is a great discipline, but smart repetition yields the best results and puts you in the best place to maximize opportunity when it strikes.
you continue to be a gem sir! - Paul (2013-01-06) #
Hi Derek,
It's interesting to see your application of SRS for learning programming languages.
On learning programming languages, this article by Peter Norvig has been cited so many times, but I would cite it again: Teach YourSelf Programming in 10 Years (http://norvig.com/21-days.html)
I would recommend the web-based SRS: http://leerit.com.
Thanks for an interesting article. It's always awesome to bring a product to a new field.
Yes! I love that article. Thanks for the reminder. — Derek - roberto malinverni (2013-01-06) #
Thanks for this tip, Derek.
I just found out that Anki is in the repos of my Linux distro :D - Vasudev Ram (2013-01-06) #
Great article. I 'm going to try this method for new subjects I learn, both a new programming language as well as other things.
- Gerald Epling (2013-01-06) #
Derek,
It is great to see you using so many meaningful pathways to memory. The timed testing echos Ebbinghaus. The "little adrenaline" that you add to your memory routine certainly helps punctuate the thoughts and set a memory in place. It seems that you have a lot of what is needed to make a memory in this plan. Thank you for your story and for posting links.
It makes sense that repeatedly testing yourself helps form memory. It is hard to get interested in remembering something that may never see again?
After carefully studying memory for some time, I tend to index into memory formation based upon a handful of experiences and processes that are likely to affect memory. Mood is important. We tend to recall memory better when the mood that we experience is similar to the mood that we had when we learned. The second thing that I look for is attention. You have to pay attention or at least notice something or someone in order to create a memory. The third thing is really three to five things that fall under the heading of organization.
Organization contains distinctive memory for individual items. Organization also includes the relationships of items, such as within-item relationships and between-item relationships. Relational processing appears to vary by intent or understanding of what constitutes a whole. A lot of this comes together with examples from face recognition.
All of this organization and distinctiveness shows how wonderfully we are made, the beauty and intricacy of the mind.
This is an interesting extension of the importance of environment on learning. We affect the environment and the environment may "remember" our being in the environment. The Anki program mirrors the actions that could be accomplished by an observant pet or the plants growing in the environment. The stimulation of memory may even come as a reflection of our thoughts returning to us from the environment.
Ebbinghaus once wrote that he had put away his nonsense syllables for some time. When he returned to them, some of them were like old friends. Here is within the relationship of friend and not within the relationship of friend processing. - Chrisg (2013-01-06) #
I knew whenever I receive an email with the name Derek Sivers on it, I'm about to absorb something very insightful. I wish there are other blogs like yours that provide tremendous value without a selfish hidden agenda attached, but anyway I'm just thankful I found this blog. Going to try ankisrs thanks to you. Chris
- Andrew Jason / Technoloco, Inc. (2013-01-06) #
Thanks a lot for this and all the cool stuff you write!
- Ivan Fioravanti (2013-01-06) #
Thanks Derek! Great article as always! I'll start immediately to follow your suggestions
- Pasi (2013-01-06) #
Thanks for sharing this Derek! I am mesmerized!
I got into rule 10 out of 20 and remembered that I need to get kids dressed and out:) Almost. This is kind of new to me. I believe I've used parts of all these different techniques unconsciously earlier. But its great to find new names and facts. And a new thing to memorize:) Cheers!
Sir Derek is ...
A. Nice - AndreaGerak (2013-01-06) #
Happy New Year Derek and thanks for sharing!
Languages have been always an exciting game for me, so I was curious to see what are you going to tell us about programming language - an area which is pretty much far from me. Thanks Gods, the Hostbaby folks do all the programming work I need :-)))
This sounds very interesting, but for me, way too technical.
If we talk about learning languages in general, beyond my mother tongue, I learned 6 languages to various degrees, so I have a little base of comparison and experience of what works and what doesn't. And this is what I found so far:
1. The WHY is more important than the HOW. If one has enough motivation (or need) to learn or understand a language, if one is keenly interested and has a strong purpose of actually USING the language, the method is almost indifferent. Keeping in mind why will it be good for you to speak that language, picturing yourself using that skill will help tremendously.
If you have a strong desire to learn it and simply LOVE learning it, look at it as a fun game and not as something you MUST do, it will be easy, no matter what methods you use.
To illustrate this, I could tell several stories for example about being able to understand a history lesson explained to me in English when I was in Turkey, years before I began learning English at all, or being able to speak some very basic Italian and communicate with the locals on the 4th week - and starting to speak Swedish only after having been in the country for about 5 years...
Another side of this is that one can learn any language to any excellent degree - but if one will not USE it, it will be forgotten quickly. Languages exist for COMMUNICATION.
2. One method I did find the most useful was when I actually went to an English language course: they used L. Ron Hubbard's study technology - yes, the controversial Scientology stuff you might have heard of. From an absolutely beginner, zero level, in 7 months (3x2hours + homework) I got to a level where I got translation jobs!
Since then, I use that super simple method to learn new songs, combined with point #1 - it goes incredibly faster that way.
You guys are many singers, musicians here, right? How about learning a repertoire of 16-17 totally new songs, in a completely different genre that you have never done before - and you rock the stage with them in just 2 weeks? :-) - Arne Jenssen (2013-01-06) #
Great article.
If you are interested in spaced repetition learning. You should also check out my new chrome extension MemoButton, that makes it easy to capture learning from internet. - Adam Hoek (2013-01-06) #
Ive been looking into learning music theory. I remembered there was a way to do it like this, but I hadn't heard of the open source Anki before. This + the 20 rules link + the 4 hour Chef book should put me on the right path I think!
Thanks Derek. - Diane Porcella (2013-01-06) #
i have the attention span of a gnat-- so remembering to do something once nevermind repeating it is sort of a big deal for me.
however i have been having some fun/success with this:
http://www.memrise.com/ - Cam McNaughton (2013-01-06) #
Hi Derek:
I'll come back to your post and the comments in due course, but I had to laugh as I was listening to the songs created by Shannon as a part of my spaced repetition listening, while glancing at your post.
I've been listening and listening to the songs on a spaced repetition basis for months now; assessing, listening, pondering and surmising along the way; it will be interesting to go back to read your article/notes in more detail, along with the comments, to see if there might be any co-relation to what I'm doing via my listening.
I guess what I'm doing is not just learning the nuances I might pick up in the songs, listening at different times, being in different moods; but, also, listening for whether or not the message and musical experience of the songs might be useful, in some way or other, to others; trying to assess that.
I'm definitely doing some kind of spaced repetition in my listening process, though; almost allowing for the space of learning, in some way; perhaps that's one of the secrets here, giving space to learning, in time. Space, in effect, can encapsulate so much, as well; all in one moment of time; then another; then another.
I might be way off base with my reflections here, but when I have some time I'll come back to your blog post and the comments that have developed here, to see if anything makes sense; of course, I might have to come at it a few times to do so; especially if I then want to try to articulate something about it; if there's something there, after all, to articulate.
Spaced repetition learning, some might say re-incarnation might fall into that type of learning; perhaps it's something our souls do regularly ... : - )
All the best
Cam - anonymous (2013-01-06) #
Great article. Thanks for giving me a new perspective on this Derek!
- Rosevita (2013-01-06) #
Thanks for bringing this up. Too little people know about this, and of those who do, only a fraction has the discipline to actually use it long-term.
Our brains are pattern machines, and the best way to build castles with your mind is learning lots of building blocks by heart.
Spaced review is also the foundation of our nonprofit project LearnThatWord, applying this concept to English vocabulary and spelling. So simple, so effective... and so overlooked.
We hope to be able to do some more research over the next few years to see if the formula can be optimized and if other variables can be added to improve it.
For now, we offer the program free to invite people from all backgrounds, ages and skill levels to join. Hopefully we can contribute to the quest for the "learning formula" in a few years! - Mark Whitty (2013-01-06) #
You were obviously a good muso Derek. Music is full of maths. An old bloke I worked for in the 1950's once told me, "Mark, there is no substitute for ability!"
That ability is sorely missed at a music distribution facility up Portland way these days.
I turned 75 on Friday In all those years I have never seen better ability and true friendship from from such a smooth and happy bloke as you Derek. No snobbery Best wishes for all the good luck in the world you have truly earned.
MTMM of OZ - Sasha aka MaximAL (2013-01-06) #
Nice article. I use this technique.
Small technical note: if you use HTML5, and `max-width: 100%` for images, don’t specify width and height attributes on tag.
This causes disproportional scaling at various screen resolutions and browser window widths.
HTML5 makes these attributes non-necessary.Cool. Thank you. Old habit. — Derek
- Vlad Koshelyev (2013-01-06) #
We are what we repeatedly do. As R. Greene put it in his Mastery book, one repetition after another, with each tiny step we get closer to perfection.
- Lucas (2013-01-06) #
What a coincidence, you published this article literally hours after I was doing a little research on that, for the first time after a couple of years :)
Anyway, this method really works and when I was using SuperMemo in the past learned thousands of new words in several months. Repetitions are very important to retain that knowledge.
You can download free (2004) version of SuperMemo here: http://www.supermemo.com/english/down.htm (I believe it's the most advanced and versatile software of this type (it's about 20 years old). Lots of stats in advanced mode, although UI a little bit crude. I've got on of the packages which is more 'modern' and slimlined)
Thank your for the article! - Meghashyam (2013-01-06) #
Splendid. I am learning a new language right now and this couldn't have come at a more apt time! I am especially glad that you recommended that software because that is the tool I was looking for but didn't know existed.
- Tom Bannister (2013-01-06) #
I knew the concept of SR learning but being mindful of it an putting it into practice was another thing. Should have guessed there was SR software - these days if you can imagine a useful tool chances are it's been implemented and just waiting for you to find it.
BTW my best time for learning is also first thing in the morning while I drink my coffee.
I seem to have developed a fond keenness for reading your articles! Thanks and all the best to you and yours.
Sincerely,
Tom - Ben Scherrey (2013-01-06) #
This technique (which I think I first heard of from you long ways back) certainly works great for anything that can be served by rote memorization. But I'd caution anyone about thinking that they know a language simply because they can understand it's syntax and remember a bunch of keywords and standard library calls. Language design is one of the hardest things in the computer field to get right. The trade offs between expressability and simplicity as well as knowing what architectural drivers caused the language to be developed in the first place, what idioms and how it implements them are more important than knowing precise syntax or function calls. Indeed when you know those aspects of a language you can often predict the simple stuff like library calls and parameters. That is knowing a language.
Just like human languages. So much is idioms. You can't really know the language without some understanding of the culture because literal translations are nonsensical. But knowing the culture strongly often allows you to navigate parts of the language that you may have previously had no exposure to.
I find, of the languages I frequently encounter, C++ and python have attained this status quite well from a language design/culture perspective. Java and C# have struggled with it and PHP never had such original intent and suffers greatly for those who try to use it beyond it's original strength and purpose. For the true geeks - Motorola's 6800X ASM was soooo much better designed than Intel's so there's significant differences even at languages with almost no syntax. - Craig Tilley (2013-01-06) #
Hey Derek. Love your posts. This post reminds me of an amazing video from Seth Godin that compelled me to share. Not that I am saying we should never memorize anything, but he said an interesting comment about kids learning. "Anything worth learning is worth looking up."
You can watch the video here - http://www.youtube.com/watch?v=sXpbONjV1Jc&feature=youtu.be
Thanks again for your valuable perspectives and Happy New Year. - Oliver Antosch (2013-01-06) #
Good to see you bring up this topic. Did you have time to look up my website? I use a similar system but with a loop.
- Pierre-Marc (2013-01-06) #
I am also learning Javascript these days, would you mind sharing your deck ?
Thanks!
Pierre-Marc
Happy to. Email me. — Derek - Dom Goold (2013-01-06) #
i use synthedit every day. if you want to move
forwards you have to work every day, because
you always have new questions to supercede
yesterday's question. your ideas move faster
than your learning and grasp of concepts, so it
is frustrating.
if you are just learning a new human language,
you may not have this frustration, because the
reward is always instantaneous: you are communicating effectively and get an immediate response or correction from your interlocutor.
computers aren't like that, unfortunately: they
have no empathy mode. - Hongying (2013-01-06) #
Oh, your post reminds me that I already installed it some month ago!
Thanks - Alexandra Lajoux (2013-01-06) #
Very interesting Derek. This is a keeper. It has broad applications beyond computer programming - and so for that matter does computer programming!
- Paul Apelgren (2013-01-06) #
Thanks for the great tips. This seems applicable to almost anything you want to learn. I'm so excited to try it. Thanks for being so generous with what you learn!
- Jon England (2013-01-06) #
(Smile) - this comment/response stream indicates you know a WHOLE bunch more geek/nerds than I knew existed in the world!
Then again - being an 40+ year ear piano-player who has over 5,000 tunes instantly at my recall...perhaps I've subconciously used 'some' kind of system myself! - Scott Kantner (2013-01-06) #
I've been looking for a way to retain the iOS SDK as I continue to learn more of it. This method seems very promising and is definitely worth a shot. Thanks so much Derek!!
- Mary Ann Hurst (2013-01-06) #
I learned Chinese at the Defense Language Institute in Monterey, California years ago. They utilized this method of learning and it is great. Thank you for the post, you're always on the cusp of something new and interesting.
- Ahmad Zaky (2013-01-06) #
"A lot of work time is spent on unplanned and parasitic activities. Phone calls, interruptions, meetings, and just plain gossiping eat up obscene amounts of time. While these events may ultimately contribute towards good circuits, they do so in a very oblique way. Worse yet, they rob psychological momentum, breaking up design time into chunks instead of allowing continuous periods of concentration. When I'm at work I do my job. When I'm at home in the lab is where the boss and stockholders get what they paid for. It sounds absurd, but I have sat in meetings praying for 6 o'clock to come so I can go home and get to work. The uninterrupted time in a home lab permits persistence, one of the most powerful tools a designer has."
--Jim Williams, Analog Engineer
Learn is all about continuous periods of concentration.
But yea... different people have a different technique.
Just another choice for efective learning. - Tim Orton (2013-01-06) #
A wise man once told me that memory is like a cup. One adds memories to the cup until it is full. From then on as new memories are added, older memories spill out and are forgotten. I have reached that point.
I have long found that, for example when learning Morse code, I hear a sound and write down an R. I hear it again and write down another R. I hear it a third time and I have no idea what letter that sound represents. Similarly, I can play a riff on my bass perfectly. I play it again, also perfectly. The third time, I mess it up. - Ahmad Zaky (2013-01-06) #
"You can know the name of a bird in all the languages of the world, but when you're finished, you'll know absolutely nothing whatever about the bird... So let's look at the bird and see what it's doing — that's what counts. I learned very early the difference between knowing the name of something and knowing something."
--Richard Feynman, http://en.wikiquote.org/wiki/Richard_Feynman
Another way for learning programming for me is look at the library of the compiler.
If it's Javascript, I suggest to look at source code library of firefox browser.
We will know why the creator use any symbol to represent any function.
See the Feynman quote about him Learning Japanese, we will understand why learning will better by knowing the concept instead of by rote. - Thameera Senanayaka (2013-01-06) #
I use email + followupthen to achieve this. No software required, no need to remember to review, no hassle. Here's my post on this:
http://thameera.wordpress.com/2013/01/06/spaced-repetition-like-a-boss/ - Nick (2013-01-06) #
This article has energized me at a very interesting point in my nearly 35 year old life. I have found learning new languages so challenging lately. I cannot wait to try this. Thanks so much!
- Brett Warner (2013-01-06) #
Once you start using spaced repetition software like Anki the applications for it start opening up all around you. I constantly think of new things I want to drop in there to make sure I can repeat them.
- Ahmad Zaky (2013-01-06) #
3 years ago I learn about HTML.
I learn something like .
I just trying to memorizing the function of "br" tag.
But something feels wrong... I don't know.
Why I use "br"? Where the "br" come from?
Patrick Naughton (Java Origin) said that one of the exact way to got a lot of money is making you as a standard... it's monopoly market.
Standard can organize, but sometimes it fools us to trust something as a right way.
That's why lecturer at university suggest us using Java, because it's a standard for having a job. - Ahmad Nadimi (2013-01-06) #
Greetings Derek,
As always your mind and heart are very creative.
Harmonically yours, Ahmad - Dominik Rodler (2013-01-06) #
Reading your post is both delightful and shocking to me.
Having founded an e-learning start-up in 2008, which had spaced repetition as one of its foundational concepts, I have never been able to understand why all these sites focused on learning to program online pop up that are praised like the next revolution and receive funding - sometimes in the tens of millions - and not one of them pays any attention about helping their customers to convert the information presented into reliable knowledge. I have tested those services myself and some of them truly exceed at *explaining* new subjects, but after only a few days, the newly acquired knowledge has completely vanished again.
Put simple, learning basically consists of 1) understanding and 2) remembering (add applying as a third aspect if you wish). Unfortunately, remembering is the less cool part and takes continuous work. The promise to watch a video once and then master your exam or professional work is just so ridiculuous and has failed in every imaginable way.
Let´s hope we will see some services in the future that take a more balanced approach and help you both understand and remember what you are trying to learn. - Randall Williams (2013-01-06) #
Pure awesomeness. I'ma gonna go populate an Anki deck with the stuff I missed on the last practice FAA knowledge test. :)
- Chemiztry - The Dope Beat Maker (2013-01-06) #
I Been Looking for Something Like this!!!!! Thanks Derek!!!
- Postcard Helicopters (2013-01-06) #
The email that brought me here gets a gold star.
There are several things in my "to learn" list: linux, python, music theory, audio production, Chinese, rules of photography, etc., so I'm looking forward to giving this software a try.
Thanks, Derek! - James (2013-01-06) #
Excellent learning method. I have always been a fan of flash cards, and using repetition to learn new skills. I will check out this software. Is there an android app for something like this you recommend?
Yep. Anki has an Android app, too. Go to ankisrs.net to see. — Derek - Bruce Chenoweth (2013-01-06) #
Every communication from you has proven to be a jewel of great value!
- Connie Oestreich (2013-01-06) #
you lost me on this one. I was excited about it until I got to the first graph. Then once I got past that I was excited again until I got to the "flash cards."
Im thinking your IQ is just a tad bit higher than mine. This one was hard to follow.
I love the concept. Interval training for memorization sounds great and very useful. Maybe I will check out some of these programs and give it a go... on topics that are not Chinese or code. lol - Dave Feder (2013-01-06) #
I think I'd like to use this for faces to names recognition. I wonder if the program will allow the use of images. Would Dale Carnegie approve?
Great post Derek
Yes! Great idea. I was thinking of doing that, too. It would definitely work. — Derek - Pete Fegredo (2013-01-06) #
Hi Derek
A very Happy New Year to you and your wife. Thank you for sharing your knowledge. Unfortunately, i cannot comment usefully here as 'am not a computer bod one bit. Don't know much about software and stuff. It is not my field. My computer skills are basic. - Andrew (2013-01-06) #
Ahh thanks for sharing.
I was taking a break from memorizing mccoy tyner and gospel esque slash chords on guitar.
I'm going to use this program to memorize the combinations of chords over specific root notes and the results they bring...nice. - Phil Hilger (2013-01-06) #
Great advice.
The issue comes when you want to know (and remember) too many things... Then 20 minutes become hours ;-) - Larry Botha (2013-01-06) #
Awesome tips, Derek!
Would you be willing to share your Anki decks?
Would be great if they were versioned on github too! - Therese Hubrach (2013-01-06) #
Thank you !!! Love to read your articles !! Great ideas. Plese remember changing my email into: [email protected] ::)))
- Ahmad Zaky (2013-01-06) #
3 years ago I read a lot of self help book, maybe 50-70 books.
At that time, I thought that if I can remember more, I can solve any situation that comes to me in day to day job.
For me, it didn't work... even in technical detail.
Last year, I tried to learn Japanese from :
http://www.guidetojapanese.org/learn/grammar
It's a great source. You can download her (Tae Kim) book for free or buy the paperback.
"My advice to you when practicing Japanese: if you find yourself trying to figure out how to say an English thought in Japanese, save yourself the trouble and quit because you won't get it right almost 100% of the time. You should always keep this in mind: If you don't know how to say it already, then you don't know how to say it. Instead, if you can, ask someone right away how to say it in Japanese including a full explanation of its use and start your practice from Japanese. Language is not a math problem; you don't have to figure out the answer. If you practice from the answer, you will develop good habits that will help you formulate correct and natural Japanese sentences."
--Tae Kim, Guide Japanese Grammar
Tae Kim change my mind a lot about how to learn almost everything.
That's why Richard Feynman gave up learning Japanese, because in Japanese for one meaning sentence we can speak it in a lot of different way depend on "who" and the politeness.
Just share :-)
Very interesting advice! — Derek - Jeff (2013-01-06) #
I'm also interested in seeing the decks. I am learning javascript too. Would love to have a start to put into Anki and also see how to setup decks for some libraries I am learning.
Great read!
Jeff - Makell Bird (2013-01-06) #
Derek, I've never really done the memory trick. Although with PHP I probably should. But I remember way back in the day HARd CODING web pages with HTML code. Alot of the code I just knew because it was the basic set-up structure. I always kept around txt documents with little pieces of code that I would pop up from time-to-time. Although I can't picture myself using old HTML code ever again. :P
- Makell Bird (2013-01-06) #
Derek I just reread this article and had an epiphany. you could actually FORCE yourself to read a book (any book) one paragraph at a time... Just space each paragraph to appear 3-6 hours apart... You could even quiz yourself at the end of the day based on the paragraphs you read for that day. And reminder quizes later.... genius!
- Jim Zachar (2013-01-06) #
Nice article Derek. I'm going to try this with my Grandson. He is having a real problem in math so maybe this will help him remember the steps to answer the questions correctly.
Thanks!
Jim - Saurabh Hooda (2013-01-06) #
I believe that programming is more practicing and less memorizing. The more you practice the better you get (and obviously with more practice you do repeat earlier learnt stuff more so that might be unintentionally spaced repeated).
I use spaced repetition technique for learning lessons in life. For example if I find some good quote/statement then I repeat it in my mind after reading/listening it. And then I intentionally try to use it once or twice in first 1 week. And then it sticks with me :) - Jeff E (2013-01-07) #
Thank you for this, Derek. I find it really difficult to learn new programming langauges and frameworks, and it seems like there is a new one every 6 months! I'm going to try this method and I hope it helps me.
- Ross (2013-01-07) #
I can see this adapted to memorize shortcuts and key combinations for IDEs also. I just switched to IDEA products from Eclipse, so I am going to give it a try. Hopefully it will help me be more efficient.
Yes! I used it to memorize more commands in vim - the text editor - very similar to IDE shorcuts. — Derek - Bayberry L. Shah (2013-01-07) #
Thanks for putting this together Derek. I love to learn but am often frustrated by how quickly it leaks out and doesn't become permanent. I am going to definitely install Anki on my phone and fill it up with items I want to remember. Just today I was telling a friend about museums I enjoyed visiting in Boston like the Isabella Stewart Gardener house and I couldn't remember which paintings were stolen even though I had read a book about it. I looked it up and remembered that the paintings included a Rembrant, Vermeer, Degas and Manet. I am trying to see all ~35 Vermeers which exist, so I should have been able to remember that his painting, "The Concert" was one of the paintings stolen in 1990 and has not been recovered.
Useless trivia maybe... but as an artist, I wish I could hold onto such knowledge once gained. - Lee Cutelle (2013-01-07) #
Thanks for the great tips. The whole programming thing has had me intrigued for years.
- Kellie Frazier (2013-01-07) #
Hmmmm....after a brain injury in '07 it took me several years to regain comprehension and relearn memorization. I painted by numbers, put puzzles together and did all the other rehabilitation methods suggested, but I believe using flash cards helped my brain rebuild its neurotransmitters faster than any other method of learning. I could picture my mirrored neurons firing off every time I memorized something. Your post makes perfect sense to me...well... nearly perfect sense outside of programming speak. In truth, your posts generally make me want to aspire to greater writings. So grateful.
- Tomasz P. Szynalski (2013-01-07) #
It's nice to see the software community discover SRS. Being from Poland (same as Piotr Wozniak, the inventor of SRS), I was lucky to be exposed to SuperMemo in the early 90s. Reviewing English and German items was my daily routine all throughout high school. We used the DOS version of SuperMemo back then.
I've written a fair bit about using SRS for learning human languages (with an emphasis on English as a foreign language) here:
http://www.antimoon.com/how/sm.htm
Very cool. Helpful article. Thanks for posting the link! — Derek - Viktar Zaitsau (2013-01-07) #
An interesting article. I need to think now where I can use that information now.
- Bruce Maier (2013-01-07) #
Mostly over my simple mind Derek but you've never led me astray so I will put the time in. Thank you for all you do
- India Holden (2013-01-07) #
I'm not planning to learn to program because that wouldn't be a good use of my skill set, but, boy, can I use these flash cards. So simple and brilliant a way to learn. I never even thought this existed. I really appreciate you for sharing your resources. Makes my life better.
- sami (2013-01-07) #
Am learning RoR now, and I really needed to read an article like this, thanks Derek, your posts always talk to me and come at the right time!
Now the challenge is to commit to this program! Installing now!
One way to help me memorize things is to have an incentive to repeat what I am learning, and this can be done by teaching what you have learned. I always found teaching very useful in learning, this is why I have started my blog to teach what I am learning in RoR - martin (2013-01-07) #
Sir, tkxs for the marvelous brilliant way to learn the program , my interests are similar to what you did in cdbaby, wld appreciate to knw how to start and what books to get, well Governer, I really respect your talent, integrity and hard work and what you ve done in your life. May you prosper in all your undertakings.
Blessings
martin - Crabmeat Thompson (2013-01-07) #
Thanks! I will use with language-learning (mine) and students (of mine).
- Olu Badejo aka TheOMan (2013-01-07) #
I'll be trying this technique out and will convince some developers to do the same, thanks for this!
- Taher shaikh (2013-01-07) #
its really great stuff and story of your life is great
- Tony Cisek (2013-01-07) #
This is great information, how can I implement it on a ultra small scale. Like learning the lyrics to a song, or memorizing a short story?
I read through most of the page and skipped to the bottom.....lots of info and I have other items on my to do list today....
What trick can I use NOW to begin implementing?
Yep. You can use this approach to remember anything. The trick is knowing how to break it down into the flashcard style facts. See the Piotr Woznak “20 Rules” link for advice on that. — Derek - Rich Baumann (2013-01-07) #
I will make some flash card reminders to quit goofing off and make the calls to book more gigs.
- Debra Russell (2013-01-07) #
Reminds me of using flash cards for learning Organic Chemistry. I created this deck of cards, one side had the reaction. The other side had the rules of the reaction (what reagent you needed, different responses in different situations, etc.).
I would spend about 15 minutes 2-3 times/day, and just go through about 5 cards each time. By the time exams came up, I could just pull out of my memory what I needed.
O-Chem was the flunk out program. My fellow students hated me because I screwed the curve :-) But really, I just used my cards... - Richard Sneed (2013-01-07) #
Memorizing a programming language ("fluency") is a worthy aspiration. Pragmatically speaking, though, I find myself eager to become conversational as soon as possible--fluency can wait, and will take care of itself over time.
The theme of Timothy Ferris' _The 4-Hour Chef_ is rapid learning. He's an advocate for deconstructing a subject. In spoken languages, for instance, you start with the most commonly used words and the most useful phrases.
It seems Ferris' approach would be a great way to learn a programming language, too. What aspects yield the greatest utility? Give me the vocabulary I need to find a bathroom and order a cup of coffee. Give me a phrasebook (examples of patterns), arranged in a way that gets me conversational right away.
The nuances--like how to do Martian trinary bubble sorts on non-dimensional arrays--can wait.
Yep. Agreed that's a more effective way to begin. And since that's what I did last time, this time I wanted to try the other way.— Derek
- Kathryn Frederick (2013-01-07) #
Thank you Derek, once again for your brillance and for sharing it. I'm going to use it for learning Italian.
- Claude S. (2013-01-08) #
Thank you!!!! I have decided to learn Adobe After Effects.. so I got a few vids, but some of the concepts are a bit difficult to remember.. going to give this a shot.. Something tells me.. It will work!!!! :D Thanks, man.. you are still a hero to all of us that bear the weight of being independent and strong.
Claude - Andre Kibbe (2013-01-08) #
"the Anki card is not where you learn - it's more of a reminder you give your future self about something you have already learned."
Ah. That's been my biggest conceptual stumbling block with SRS. I've been trying to commit information to cards during or before committing it to understanding—memorizing before learning.
Up until now, I've always used Anki to memorize broad definitions and concepts, not specific examples that reinforce and understanding of those definitions and concepts.
Thanks for ramping up my learning curve! - Julie May (2013-01-08) #
Just a note that this is a well-known model and has been used for a long time among trainers. Great to see some software for personal goals though. Check out Kirkpatrick's model and other adult learning models for even more fun.
- Capt. Alaska (2013-01-08) #
Derek, thanks for these insights!
I am not sure if it is appropriate to mention here, but I use the following approach to learn foreign languages: I prepare a piece of text that I want to memorize and type it in a special editor which lets you hide every second word so that you have to recall this word before you can move forward. Details and screenshots are available if you click at this comment's author mentioned above (Alaska Capt). - SeanG (2013-01-08) #
I used to tell my guitar students the best practice habit they could have was to practice when they got home from their lesson. It was obvious when students would go home an not pick up the guitar again for two or three days.
- matt harrison (2013-01-08) #
I would love Kindle integration with this... hint hint amazon
- Steve Boller (2013-01-08) #
The company I work for has developed a game engine that uses spaced repetition to help learners memorize facts quickly. It functions similarly to the Anki software, but it is a web app designed for corporations to train sales reps and support teams on product features, jargon, industry facts, etc. By taking the spaced repetition principle and placing it in a game setting, memorizing new information becomes efficient and enjoyable.
I am a major believer in spaced repetition... glad to hear you are seeing great results as well!
Oh, and you can try it out yourself if you want at http://theknowledgeguru.com. - Jai Shekhar (2013-01-09) #
This is a fantastic idea, I want to know if you / anybody can help me able to remember all different software products and the purpose of these products using this methodology.
- Tulio Teixeira (2013-01-09) #
Awesome post once again Derek. I'm readying about learning more efficiently as well. calnewport.com/blog/ and www.scotthyoung.com/blog/ are other great places to go to.
- Ryan Chilcote (2013-01-09) #
So this was my cool app idea I had for a few years; so see what happens when you wait? However, I now don't have to wait to use it! This will help me and my students.
P.S. Good call on keeping responses (comments) private. I assume I'm not the first to reply to this blog :) - Patti Rayfield (2013-01-10) #
Thank you so much Derek for such a wonderful piece , it is so helpful for me just now I am learning a lot of new stuff.
Wishing and your family a great year ahead - Tim Anderson (2013-01-10) #
Thanks a lot, Derek - absolutely brilliant concept!
I'm now learning Norwegian on my Android phone... - Rip Rowan (2013-01-10) #
Terrific and thought-provoking article, Derek. Please forgive the rambling reply. The first part deals primarily with learning as it applies to computer languages, then I rabbit-trail off to the topic of learning in general.
It is interesting that you chose programming as an example for your article. I've been a programmer for over 30 years. I've worked with some assembler, PL/1, COBOL, C, C+, C++, C#, BASIC in all forms, Pascal (really!), Java, Javascript, HTML in all forms, SQL, NoSQL, PHP, Perl, and a crapton of of other stuff (for a while I was a rockstar of the Lotus @formula language). Next up is probably Python. I'm not Linus Torvalds, but I've done some decent work and even had three projects nominated for Computerworld awards. In short, I've been around long enough to have to have done some pretty decent work, and to have forgotten most of it.
Almost everything I know was learned as-needed. I would venture to say this is the norm among career software designers.
When I want to learn a new language, I *start* by writing a program in that language - not an "exercise" program but something that I plan on actually using. And how do I get started? By looking at someone else's code and using it as a starting point. I have written very, very few programs that started with a blank page. Even if I reuse only a little piece - some declarations, a subroutine, *anything* - I almost always start with a little of someone else's work.
One of the reasons I learn in this way is that I'm very ADD. Rote learning does not provide enough stimulation to keep me focused. But I also recognized early on that to really "know" a language deeply might be a double-edged sword for several reasons.
When I was in school, you learned languages like COBOL (in MIS classes) and c (in comp-sci classes). Well, COBOL is dead and c as it existed when I was in school (pre-ANSI-c) is also (essentially) dead/evolved past recognition. I was out of college for less than 5 years before I was exclusively using technologies that were not even available for study while I was in college.
Besides the issue of obsolescence, I would go so far to say that for a career software designer, fully mastering one language might actually be counterproductive to the job. Why would I say that? As a different commenter already mentioned, computer languages grow from a particular culture and need. Want to write an operating system? Choose c. Want to write a concurrent realtime system? Consider Erlang. Want to code a web page? Use HTML and Javascript.
The problem is, when you have a hammer, everything looks like a nail. I have run into many people who are experts at one particular language, and their deep language expertise shows in two ways: (1) they construct ordinary code in that language a lot faster than me (2) they are unable to think creatively about ways to solve their problems because they are trapped in the solution spaces suggested by the language they know. I have seen #2 happen. A. Lot.
So as someone who has been in the field for decades, and who has watched languages and technologies come and go, how much syntax and language do *I* want to commit to *my* neurons? *As little as it takes*. The idea of learning "everything" about a language sounds... horrible, actually. Painful. Pointless. A waste of time and mental space. A guaranteed path to obsolescence. I think I speak for a lot of software designers when I say this.
So instead of learning how to program in a given language, I suppose I've learned a different skill: how to program when you don't really know any language very deeply at all.
Of course this does not invalidate your article. Far from it. But it does open the door to some interesting questions about learning styles and the applicability of different learning methods. Specifically, how could I apply a memorization technique like SRS to an experiential learning process? Is that even possible?
To look at the issue completely differently, I am currently in Italy and learning Italian. Now, I may be comfortable with an unfamiliar programming languages, but learning natural languages has always been very difficult for me. And speaking Italian is not like speaking SQL. I can't copy and paste a useful sentence from another conversation.
I can quickly see how something like Anki could be useful to the task of memorizing Italian words and grammar. Curiously, however, the world seems to have moved on past memorization as an effective way to learn "practical" information (by "practical" I mean "information you plan to use right away").
I enrolled in language classes here in Italy last year, and interestingly, there is no text, no drills, no tests, no workbook, no grades, and zero memorization. Just full immersion with handout exercises - most of which are not even completed much less graded or checked, because the whole thing is just a vehicle for getting students to read, write, and talk as much as possible. "Correctness" is completely optional and comes later. And almost everything in class is done in groups of two or three, with individuals serving alternately as student and teacher.
This feels very much like the way I learn programming languages. And it works - I took 4 years of high-school French in a very good school, but I learned more Italian in one month of full-immersion here in Italy than I did in four years of memorization, drills, and tests.
Being a nerd, I also have checked into Rosetta Stone and other software for language learning. I ultimately did not stick with RS because I really don't want to have to be at a computer. However it bears noting that in RS as in my language school in Italy, there is almost no "overt learning" - you just jump in and start doing - with zero memorization. The learning is subliminal.
This also feels like the way I learn programming languages.
I have always felt as though there were two rooms in my brain. One room contains "intellectually-acquired" information - things I learned by reading, memorizing, etc.. Call this "overt learning." The other room contains "experientially-acquired" information - the things I learned by doing. Let's call this "covert learning."
It always seems to me that these rooms are separated by a long hallway that makes it challenging to move information from one room to another. For example you could master 10,000 words of Italian solely through memorization and find it VERY difficult to construct a context sentence on the fly. Conversely, you could be fluent in speaking solely through experience but struggle with verb tense forms, spelling, and nitpicky language rules.
Everyone knows the stereotype teachers and practitioners. The stereotype teacher can spend a week telling you everything there is to know about how to tie a shoe and yet not be able to tie a shoe. The stereotype practitioner can tie shoes all day long and not be able to even begin to tell you how they do it, except for that it might have something to do with laces. But when you run across a teaching practitioner - someone who not only "does it" but also "teaches it" - *THAT* is a person who has mastered a subject.
OK. In the words of The Stranger, "aw, look at me. I'm ramblin' again." - Ryan Anderson (2013-01-11) #
Thanks a million for sharing. It's already had a huge impact in the way I remember things. Total game-changer.
- Migue (2013-01-13) #
Derek, what is the software you are using for Chinese learning as shown in the prints?
Would you recommend me a tool for that?
I've been employing a lot of time for character learning, and think that something like this would finally help me getting faster!
Thanks in advance,
Miguel
That's Anki! The best book I found for learning the Chinese characters is Tuttle Learning Chinese Characters. The best audio program I found for learning to understand and speak the language is Michel Thomas Mandarin. — Derek - Leonardo (2013-01-13) #
Very nice article Derek! I'm thinking about using it for technique Piano and Guitar technique exercises ;)
- Désirée Staude (2013-01-15) #
Hello Derek,
Thank you very much for this great information. I have downloaded Anki and will use it for my future learning!
You are really amazing to be so helpful and passing on your information. Thank you very much!
Kind regards from Germany
Désirée - Graham Talley (2013-01-15) #
Just adding my thanks to the list! I'm a non-technical entrepreneur, and went through a very similar process of teaching myself to program.
Looking forward to using Anki!
I go through periods of months sometimes without checking up on the bloggers I like, and yours is the only one where every post is always worthwhile.
Thanks Derek. - Brett Law (2013-01-19) #
Hi Derek,
Stumbled onto your site today. Bookmarked. I'm really interested in this Anki program. More interested in what could be accomplished using the infrastructure in order to teach programming. If you'd like to hear what I have to say about making programming a little easier to learn (this pertains to me because I am currently learning it via codecademy.com, feel free to e-mail me and perhaps we can talk JV.
Regardless, great content! I appreciate it greatly.
Thanks,
Brett - Jeremy Shuback (2013-01-19) #
This is incredible. Scratch that. This is life changing. Thank you so much for sharing Derek.
I was skeptical that SRS would work for me and my terrible memory. I've tried plenty of techniques in the past. For instance, I spent a while learning the peg system in an effort to be able to memorize dates, numbers and lists. While it worked, it serves me more as a party trick than an actual life changing approach to memory.
What I particularly love about using Anki, which I've been doing for a half an hour a day on the bus since reading this article, is I feel the information is actually sticking. Because of that, I want to spend more time learning, and because of that my memory muscle is actually growing stronger. My ability to memorize quicker and quicker is already improving. I've gone from hating the very idea of memorizing to getting excited about learning more. I've always been frustrated wasting time memorizing because I knew it was facts I'd just forget in another couple months. This doesn't feel like that. This feels like I'm gaining a super power.
I did a small test over the course of the last three weeks to see if Anki would really work for me. I didn't want to jump into something ambitious like a programming language before seeing if it actually worked for a small data set. I challenged myself to use it to learn the names of all of the countries in the world. There's less than 200. If Anki could teach me those, I had faith I could then go on to more ambitious challenges.
I loaded a shared deck, and three weeks later, I feel confident I know them all. It took half an hour on the bus every day, but that's a small sacrifice. It will take a few more weeks before it's cemented for life, but that's incredible. Now I'm excited for what I'll be taking on next. (In this order - bones of the body, muscle systems, names of all of my facebook acquaintances, street names in San Francisco, Biblical Hebrew, Music Theory, Wordpress tags - that should take me well into next year)
Life changing Derek. Life changing. Thank you.
One question - does anyone know of a program out there that will automatically sort through your facebook friends and turn them into an Anki deck? I'm terrible at names, and this would be amazing for me.
Awesome! Great to hear. Sorry I don't know of any automatic Facebook → Anki thing. But maybe doing it by hand is the first step of remembering them? — Derek - William Moroney (2013-02-07) #
Wow, Derek! Awesome wavelength you travel on! I'm a singer/songwriter and online guy. I appreciate the hand up with this tool to help me keep so many constant new knowledge and processes together. I just sure do appreciate you taking the time, and, to thank you for CD Baby, too. Great that it's a business for you, but, Very Cool that it gives the new generation of Artists a venue to move forward in their careers. I appreciate your work and considerations.
- Christian Paulsen (2013-02-11) #
Great insight on a process I feel has a lot of nuance. I tried something similar sometime ago and gave up thinking that programming knowledge just didn't fit with flashcards. I see that I gave up too soon. I'm looking forward to giving it another shot.
- Zeeshan Parvez (2013-02-19) #
Have you tried Mnemosyne?
Similar, but I prefer Anki. — Derek - Matthew Bailey (2013-03-07) #
Wow, this is really cool and I'm starting to wonder whether this is how they designed duolingo, which I have been using to learn spanish. Though, not good enough. Need to do it every morning like yourself.
Does this work when learning 2 things? say HTML/CSS and Spanish? - Stephan (2013-04-02) #
Super, thanks for the Anki set for JavaScript :)
- Jonathan (2013-04-30) #
Wow, I've been using Anki for language learning and teaching my English as a Second Language students. But I didn't know it could be used for learning programming languages. Cool!
- Billy Thompson (2013-08-06) #
Thanks for the great post. I am currently trying to learn CSS,PHP and Javascript and although I was reading alot, When it came to putting the knowledge to use I would find my mind going blank. Thanks Derek for taking the time to post the article on memorization.
- Valentin (2013-08-10) #
That is fun. It would be an interesting experiment if tutorials and learning materials would be formed into such exponential drilling standard file format, for use by students.
- Neil Loehlein (2013-08-25) #
Thanks for the post, Derek! I'm going to try this method out. I'm in the process of learning Javascript right now, and I could definitely benefit from a better retention of what I'm learning.
- Theo (2013-08-25) #
Another useful tip for generating relevant flashcards:
Take a blank sheet. Pick a topic (say, arrays). Pretend that you are writing a chapter of a book on the topic. Jot down every related concept, methods, memory model, the most efficient search algorithms, etc, everything to do with arrays.
Then, check a great programming book. See if you missed anything important.
Create flashcards on those gaps. - Peter Williams (2013-08-28) #
Awesome as usual Derek. Put it together with this post on how to avoid procrastination and all excuses evaporate. One question: you mentioned the flash card software is free, it's showing up at $25.99 on my iTunes store (Australia). I'll explore further..
http://blog.bufferapp.com/how-to-stop-procrastinating-by-using-the-2-minute-rule
Go to ankisrs.net and click Download. The Windows, Mac, and Android versions are free. Only the iPhone app costs money. Try the free desktop version for a while, then buy the iPhone app if it seems worth it. — Derek - Masamichi Takigawa (2013-08-28) #
Thank you every Universe.
This is me.
I realize me who I am.
My name is Load of Brilliant Sun.
Emperor of the Sun.
Please realize this dimensions is already in the peace and love.
Keep Riding . Keep Groving, Keep Rocking, - Peter Weis (2013-08-28) #
Derek do you know if this is the same or similar type of algorithm that Duolingo use for language learning?
Sorry I don't know. — Derek - Angèle (2013-09-15) #
This is most excllent!
- Kim Bynum (2013-10-08) #
Hi Derek, I tried to download Anki on my laptop, windows 7. It won't run, getting a message my time and date are set wrong, but they aren't. Do you have any suggestions? thanks for your info. I am currently struggling with a javascript class, and looking for a way to learn it that I can understand! Kim
Sorry I don't know what to recommend. See the Support page on the Anki website or just Google the error. — Derek - Ernie Cordell (2013-12-01) #
You're absolutely right: Learning is a natural process and we often focus on instruction without thinking of that axiom about learning more in play than in study. We learn the most in life as infants, by exploring and re-exploring, by trial-and-error, but mostly error. We learn best when we receive the lesson that we forgot, and when we are fortunate, we are reminded before we make the next mistake. We crave the structure of the classroom because that's what mandatory attendance teaches us. People ask me to teach them, but the successes are testimony to the desire of the pupil and my biggest role is just reminding them not to become discouraged.
- Beto (2013-12-20) #
Great post I've have read in the longest longest time and I don't really reply only if it's mind awakening and it's rare. Thanx! =0)
- TRUEAFRICANS (2013-12-31) #
Sir, you are really amaze and talented. Watching the video of you on TED, especially the exemplification of leadership, encouraged me to do more research about you. Also, I've learned a lot trick and advices from you. As a result, i question myself, "How can people of a third countries get such important advise from and other positive people in this WORLD.
- Michael (2014-01-10) #
Hi Mr.Sivers, we came across this article when we started developing our own flashcard app. It is called cardkiwi - http://cardkiwi.com - and allows students to collaborate on their flashcards. In the background we run the spaced repetition algorithm. We built the site for ourselves as we are two students. Thanks for your awesome blog posts!
- James H. (2014-03-06) #
Just what I needed while I'm preparing for technical job interviews. Thanks!
- Kevin (2014-06-03) #
Thank you for the post. I have taken the same approach and created a large number of flashcards on BrainThud. The flashcards are mainly on the Microsoft stack but also include JavaScript, HTML & CSS. I thought I would post it here in case anyone else would like to use them. I found it very valuable while studying for the MCSD.
BrainThud can be found here: http://www.brainthud.com - William Lee JC (2014-06-30) #
Just what I've been looking for! This should aid my journey in picking up Ruby and Mastering my markup languages; perhaps I would use this technique to expand my multilingual-abilities too!
Thank you very much for sharing this! :D - yo (2014-07-30) #
I completely agree with the effectiveness of using SRS to become more fluent in languages, especially vocabulary, whether it be natural language or programming language.
Anki looks great in terms of features, but usability-wise it looks cumbersome and dated. Would you use a web/cloud-based SRS with a simpler UI, accessible from mobile and browsers, if there was one? Or do you know of any? - yo (2014-08-21) #
Simple, modern web-based flashcards, something like this: https://ankimono.com/app?deck=48#/decks/48/info
(No SRS yet, but I hope you get the idea. I'd love to hear what you think.) - Dominik (2014-08-24) #
Thanks so much for the Anki deck on JS!!
- Steve Luu (2014-08-24) #
Thanks for sharing. I've been wanting to pick up some coding myself and this, along with the other linked articles, are great primers on effective learning.
- Rob Bednark (2014-10-18) #
Nice article! I found your examples very helpful -- the variations of question formats. Thank you for sharing your decks.
- Ytrek (2014-10-31) #
Hi Derek,
Do you still think it's the best thing you did to improve your programming skills ?
Yeah. Of course it's more important to go do lots of programming. But think of this like memorizing a spoken language. You do flashcards to memorize the words and phrases, but then you make it real by getting out into the world and speaking with people. — Derek - Bruno (2015-02-10) #
Second time i came over to this article. I found it very helpful. Thanks
- Milan (2015-03-13) #
Nice tip it's really helpfull.
- Ray (2015-03-25) #
Thanks for posting this! I used SuperMemo 98 way back when to help pass some of my courses back in 2000.
I found this by accident and will be using the latest to study new tasks. - Ibrahim Islam (2015-03-26) #
Very nice! I am starting with Anki right now!
- Bruno (2015-04-05) #
Great Article!, tx a lot
any updates about if you are using it these days?
cheers! - Osama Khan (2015-04-13) #
Any plans to prepare a Python Deck?
No. — Derek - Bhati (2015-04-14) #
Thank you.. this is exactly what I was looking for.
- Gheren (2015-06-18) #
Hi,
I liked your article on spaced repetition and Anki. I like Anki but wish it had more of a learning mode, something before its cramming and filtered decks.
I am a student who has not learned to code. If an Anki like program , with a learning mode were to be written which languages would you use and what would you charge?
Thank you!
gheren - desertwalker (2015-09-09) #
Thanks for bringing this to my attention. It all makes good sense
- Tim Shape (2015-10-17) #
Excellent and useful post! I just started to use Anki for learning PHP and can see the value in adding it to my learning discipline. Thank you.
- Erez Zukerman (2015-12-01) #
Shameless plug -- I developed a tool for this exact purpose (flashcards for programmers), called CodeCode Ninja, at http://codecode.ninja :)
Quite happy with how it came out, would love to hear thoughts from people who are as into this as I am. - Ammar Rai (2015-12-05) #
Thanks! I've actually been using Anki for quite some time. I loved this post post (as well as the readability of your website!)
☺ Thank you. That's the first nice comment about this website's design in a long long time. — Derek - des mccarthy (2016-01-02) #
Thank you so much for this. I have been going around in circles for years while not being able to retain to much of what i have been trying to learn. Thank you!
- 张扬 (2016-01-13) #
worth to try
- kevin carey (2016-01-18) #
It is nice to know that there are people like you who are willing to share their knowledge, and learning thoughts. I for one appreciate it. I wanted to investigate javascript and you have given me a clear path to follow. I will also investigate the learning aids too, Thank you, kc
- jargon (2016-02-05) #
hey im an aspiring freelancer for web development ive been coding in python and c/c++ running the bash shell since i was 21 when i got into computer science this tip is definitely going to help i c that already n i liked how u said u studied in the morning with tea made it seemed more relaxed b4 u started all that gym talk lol... well id also like to keep in contact with you and n e other programmer i meet ive got a github im going to be building a website for linux tutorials and programming its going to be on github soon maybe stop by n give some tips/ideas im just hoping to surround myself with ppl like me and who have a developer job id like so as to possibly land a job doing what i passionately love! well ty 4 the tutorial much appreciated!!!!!!!
- Ron (2016-02-23) #
I so wish I had tried this method a long time ago! I would be so much farther ahead. Hey, I guess it's fate. Thanks a lot for the info.
Ron
Visit Today!
Visit Today!
Visit Today! - bosko (2016-02-24) #
Tnx man. Just started learning Javascript. Anyone from Croatia??
- Mike Tappe (2016-03-08) #
I am an Anki user and have been using it to make language cards. I am attempting to learn Spanish as I word with hispanic crews.
I wonder if you do programming for hire. I am a concrete contractor and really have to spend time on my jobs and don't have time to learn how to do only the basic cards.
I would like to talk to someone like you and be able to tell them what my needs are and they use their toolset to help me make cards.
I would like to be able to use Anki to take concrete manuals and other types of books to make card decks I could use or have employees use.
Would you be interested in talking? I am in need of help and of course willing to pay for that help.
My phone number is 612-919-6800. You could text me and let me know a time to call, if you miss me. - Gavin James (2016-04-20) #
Hi Derek. Great article! What else have you use SRS for? Would be great to see the comprehensive list for inspiration.
Just foreign language vocabulary, which is how most people use it. — Derek - kraker (2016-04-27) #
Revisiting spaced repetition for learning clojure after shelving the method. I think this is the key to long-term learning vs. short term expediency. Thanks again.
- Dan Dickson (2016-05-04) #
God bless you Derek! I have craved information on learning & RETAINING programming knowledge since I was a kid (now I'm 37). I learned more in this one article than in years of searching and reading about metacognition and memorization. Thank you! Thank you! Thank you!
- Oliver (2016-08-30) #
How'd you format your cards? I tried some code but all of the anki clients that I'm using center all of the text.
Edit the basic card template in settings, to remove the centering. You'll find it. — Derek - Wang Peng from China (2016-10-18) #
So cool~ I saw Chinese,too many foreigner said Chinese is too difficult, but in eyes, English is too.
return too this passage,if you ask me 'do you have a teacher?'
I will answer:'是的,你知道吗,我的老师是Derek Sivers'
thats mean:‘yeah,you know,my teacher is the Derek Sivers’ :)
☺ — Derek - kevin (2016-11-07) #
Hey Derek, you're a good guy. I think I visited your site a while back, looking up books to learn javascript. Almost a year ago. I forgot about the anki method. I'm going to try it again and keep at it this time. Very helpful, thanks kevin.
- Mikel Gonzalez (2017-02-05) #
I know this is a bit old, but I wanted to weigh in on this. I've been looking for something better than anki and more geared toward programming. I couldn't find anything so I decided to build out my own solution. It's still in the early stages, but it works well.
https://codecardio.herokuapp.com - Vanden Bussche, Johan (2017-02-06) #
There is a brand new software title out now: "My Personal Study Coach" (http://www.mypersonalstudycoach.com).
One of it's most interesting new features is that it not only supports FlashCards but (among others) also "FlashText". This allows users to paste programming code and yellow highlight sections within that code. When user interrogate themselves they then see 80% of the code and just have to train themselves "filling in the blanks" (the yellow highlighted sections). That seems to be a very effective and easy way to practice specific code segments.
The software also supports spaced repetition in a unique way: each item turns "green" when mastered and turns back to orange after a period of time, when rehearsing is recommended. - Mike (2017-03-22) #
Great article, thank you :)
- Kurt Anderson (2017-05-24) #
I went searching for an alternative flashcard app that runs either web native or within chrome since I exclusively use a Chromebook.
I remembered that Duolino recently came out with tinycards, which uses the same type of algo to prompt you to review your flashcards. The very best part is that you can create your owns decks, and share them.
I'm always looking for a way to not use 'software' but rather services! - Ilya Kushlianski (2017-07-14) #
Amazing article, I've been doing the same for about 6 years now and one year ago I decided to learn programming and become a developer, I started with Javascript and Anki is the tool I'm using!
I already have 8338 cards on various topics, like PHP, nodejs, gulp, css, jquery, SQL and so on!
It's hard sometimes to make yourself start learning something but once I start it just becomes part of my life. Whenever I have 5-10 free minutes I do the repetition and that helps me a lot!
Cool! — Derek - Sean Crawford (2017-10-18) #
For comment # 94, which is I suppose is correct about learning Japanese, I think it is wrong about why Richard Feynman quit trying to learn Japanese.
As I understand it, it was not because of Japanese saying one thing in many different ways, but because the language was undemocratic, with built-in honourifics.
This was the man, remember, who learned to jump down stairs so that when he received his Nobel prize from the king he wouldn't have to bow. I am the same: I gave up learning Esperanto after I found out they put a gender onto all men and women.
For example, not fire fighter, but fireman and firewoman.
In contrast, the hero-narrator in the future science fiction series Chtorr Wars will note a couple soldiers in the background, and only a couple paragraphs later casually mention what gender they are. - somebody (2018-02-17) #
For learning programing, I would prefer to type the answer. And it's easily achievable with Anki and its cards' type.
# create a template
go to --> "Add" (a card)
click on --> "Cards" (ctrl + l)
in the field "Front Template" put (exactly as it's written):
{{Front}}
{{type:Back}} - GeeGeeX (2018-04-22) #
This is only good for learning the syntax and conditional statements.
Run-time and compiler errors will still have to be reviewed and fixed by hand. You will still have to know the difference between identifiers and reserved words. - steve adwell (2019-03-12) #
Hey Derek, Thank You for the tips over the years. I am about to start learning Italian and remembered your article. It only took a few minutes to find and I have looked up Anki. I am about to download it and install it. I will have to actually use it to get the benefit of course. If I stick with it and actually can master another language I will post a note here. I assume that you get notified when people post something on the older articles.
I hope you are well,
Steve - Jaroslav (2019-08-14) #
I was so glad to read advices, which I found out by myself! E.g. make it daily in the very beginning of a day. Thanks for the short but deep tour into memorizing techniques!
- Alex Kraker (2019-11-08) #
Mr. Sivers,
Just a friendly note that the above link '"Janki Method Refined" by Jack Kinsella' is broken.
But you can find it here: https://www.jackkinsella.ie/articles/janki-method-refined
Thanks as always for the extremely useful content.
Cheers,
Alex - Joana Mufume (2020-02-07) #
I beleave this is a perpect method fo me to learn English.
I love this method - Beau (2020-05-01) #
Any insights and/or revisions to this after ~7y? I've used Anki off and on for years for different things, curious about your long-term experience.
Nothing new, no. — Derek - Brenton (2020-12-17) #
I can also attest to this method. It allows you to make connections that only come with seeing and understanding patterns deeply.
I've always used physical flash cards so I can take them anywhere and they incorporate different learning modalities.
I'm curious what your preference is. It seems to be Anki, or other software. I know you're a big proponent of tech independence, so how do you factor that in? Use the software and back it up in a personal database?
Brent
Anki, yeah. It's open source, installed on my private encrypted OpenBSD laptop, offline, so I have the database of all cards right here. No cloud. — Derek
Your thoughts?
Please leave a reply:
Your Name
Your Email
(private for my eyes only)
Comment
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK