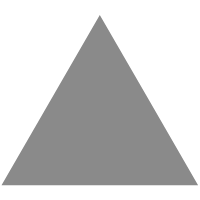
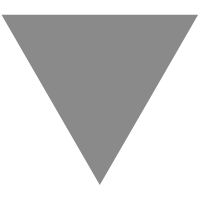
An easier way to generate PDFs from HTML templates
source link: https://developers.redhat.com/articles/2022/03/11/easier-way-generate-pdfs-html-templates
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
An easier way to generate PDFs from HTML templates Skip to main content
Applications frequently are required to generate invoices, reports, ID cards, and much more in PDF format. There are Java libraries and tools that developers can use to generate PDFs, including the popular JasperReports. While sophisticated, using these programs can be complicated because they support a wide range of documents.
This article introduces a simpler tool, the open source wkhtmltopdf utility. I will show you how to use wkhtmltopdf
to solve a common scenario: You have an HTML form, parameterized to accept input data, and you need to produce a PDF from the data in that form. You will learn how to set up your data and make a call to the wkhtmltopdf
utility from a Spring Boot web application. We'll use Red Hat's Universal Base Image (UBI) 8 as a base image to simplify the application build, then deploy the final image into Red Hat Openshift 4.
Note: You can find the code for the example in my GitHub repository.
Configuration using Maven
Configuration using Maven
Let's start with a Maven file. I'm using a Snowdrop bill of materials (BOM) on my Maven project object model (POM) file instead of a community version of Spring Boot:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.edw</groupId>
<artifactId>SpringBootAndPdf</artifactId>
<version>1.0-SNAPSHOT</version>
<repositories>
<repository>
<id>redhat-early-access</id>
<name>Red Hat Early Access Repository</name>
<url>https://maven.repository.redhat.com/earlyaccess/all/</url>
</repository>
<repository>
<id>redhat-ga</id>
<name>Red Hat GA Repository</name>
<url>https://maven.repository.redhat.com/ga/</url>
</repository>
</repositories>
<pluginRepositories>
<pluginRepository>
<id>redhat-early-access</id>
<name>Red Hat Early Access Repository</name>
<url>https://maven.repository.redhat.com/earlyaccess/all/</url>
</pluginRepository>
<pluginRepository>
<id>redhat-ga</id>
<name>Red Hat GA Repository</name>
<url>https://maven.repository.redhat.com/ga/</url>
</pluginRepository>
</pluginRepositories>
<properties>
<snowdrop-bom.version>2.4.9.Final-redhat-00001</snowdrop-bom.version>
<spring-boot.version>2.1.4.RELEASE-redhat-00001</spring-boot.version>
<maven.compiler.source>11</maven.compiler.source>
<maven.compiler.target>11</maven.compiler.target>
</properties>
<dependencyManagement>
<dependencies>
<dependency>
<groupId>dev.snowdrop</groupId>
<artifactId>snowdrop-dependencies</artifactId>
<version>${snowdrop-bom.version}</version>
<type>pom</type>
<scope>import</scope>
</dependency>
</dependencies>
</dependencyManagement>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
<build>
<finalName>app</finalName>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<version>${spring-boot.version}</version>
<configuration>
<mainClass>com.edw.Main</mainClass>
</configuration>
<executions>
<execution>
<goals>
<goal>repackage</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
</project>
The Spring Boot program
The Spring Boot program
Our main class in Java is:
package com.edw;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class Main {
public static void main(String[] args) {
SpringApplication.run(Main.class, args);
}
}
The following Controller
class displays a generated PDF. One of the class's most important tasks is to display the PDF properly in a browser; this task is achieved by setting up a proper MediaType
that produces an application/pdf
content-type header. Another important task is to call an external process that runs wkhtmltopdf
to trigger the conversion from HTML to PDF:
package com.edw.controllers;
import org.springframework.http.MediaType;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestParam;
import org.springframework.web.bind.annotation.ResponseBody;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.Scanner;
import java.util.UUID;
@Controller
public class ReportController {
@GetMapping(
value = "/generate-report",
produces = MediaType.APPLICATION_PDF_VALUE
)
public @ResponseBody byte[] generateReport(@RequestParam(value = "name") String name,
@RequestParam(value = "address") String address) throws Exception {
String uuid = UUID.randomUUID().toString();
Path pathHtml = Paths.get("/tmp/" + uuid + ".html");
Path pathPdf = Paths.get("/tmp/" + uuid + ".pdf");
try {
// read the template and fill the data
String htmlContent = new Scanner(getClass().getClassLoader().getResourceAsStream("template.html"), "UTF-8")
.useDelimiter("\\A")
.next();
htmlContent = htmlContent.replace("$name", name)
.replace("$address", address);
// write to html
Files.write(pathHtml, htmlContent.getBytes());
// convert html to pdf
Process generateToPdf = Runtime.getRuntime().exec("wkhtmltopdf " + pathHtml.toString() + " " + pathPdf.toString() );
generateToPdf.waitFor();
// deliver pdf
return Files.readAllBytes(pathPdf);
} finally {
// delete temp files
Files.delete(pathHtml);
Files.delete(pathPdf);
}
}
}
Formatting the HTML data
Formatting the HTML data
The following HTML template generates a report with input data consisting of names (the $name
parameter) and addresses (the $address
parameter):
<html>
<head>
<style>
<!-- put your css here -->
</style>
</head>
<body>
<div class="container" style="padding-top: 100px;">
<div class="row justify-content-center">
<div class="col-md-6">
<table class="table table-bordered">
<thead class="thead-light">
<tr>
<th>Name</th>
<th>Address</th>
</tr>
</thead>
<tbody>
<tr>
<td>$name</td>
<td>$address</td>
</tr>
</tbody>
</table>
</div>
</div>
</div>
</body>
</html>
Installing the wkhtmltopdf program
Installing the wkhtmltopdf program
This next part is where the magic happens. First, you need to download wkhtmltopdf
from GitHub and extract the program. After that, you can create a wkhtml
folder within your Java project and copy wkhtmltopdf
to the application's folder from the bin
folder:
$ wget https://github.com/wkhtmltopdf/wkhtmltopdf/releases/download/0.12.4/wkhtmltox-0.12.4_linux-generic-amd64.tar.xz
$ tar -xf wkhtmltox-0.12.4_linux-generic-amd64.tar.xz
$ mkdir /code/wkhtml
$ cp wkhtmltox/bin/wkhtmltopdf /code/wkhtml/
The resulting directory structure looks like:
+--- .gitignore
+--- Dockerfile
+--- pom.xml
+--- src
| +--- main
| | +--- java
| | | +--- com
| | | | +--- edw
| | | | | +--- controllers
| | | | | | +--- HelloWorldController.java
| | | | | | +--- ReportController.java
| | | | | +--- Main.java
| | +--- resources
| | | +--- application.properties
| | | +--- template.html
| +--- test
| | +--- java
+--- wkhtml
| +--- wkhtmltopdf
Building the image
Building the image
The following Dockerfile uses UBI 8 as the base image:
FROM registry.access.redhat.com/ubi8/openjdk-11-runtime:1.10
USER root
ENV LANG='en_US.UTF-8' LANGUAGE='en_US:en' TZ='Asia/Jakarta'
RUN microdnf update && \
microdnf install tzdata libXrender libXext fontconfig && \
ln -sf /usr/share/zoneinfo/$TZ /etc/localtime && \
microdnf clean all
COPY wkhtml/wkhtmltopdf /usr/local/bin/
EXPOSE 8080
USER 185
COPY target/app.jar /deployments/app.jar
ENTRYPOINT [ "java", "-jar", "/deployments/app.jar" ]
Now, build your image and run it:
$ docker build -t springboot-and-pdf .
$ docker run -p 8080:8080 springboot-and-pdf
In your browser, enter the generate-report
URL with a name and address as parameters, and you get a PDF ready to download and print, as shown in Figure 1.
Conclusion
The streamlined process shown in this example is useful in a variety of situations where you want to generate a report with tabular data or another structured PDF based on input data. Feel free to leave comments on this article to discuss where you might use this approach and any questions you may have.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK