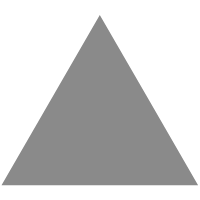
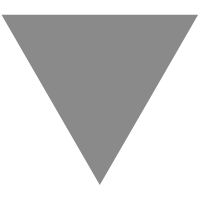
How to Export Large CSV using Laravel LazyCollection with Example?
source link: https://www.laravelcode.com/post/how-to-export-large-csv-using-laravel-lazycollection-with-example
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
How to Export Large CSV using Laravel LazyCollection with Example?
In this article, i will share with you how to export a very large CSV file in laravel using the lazycollection and cursor with example. you all face many time issues with an export very large file. if you try to export 10M record in one call then the PHP/apache
did not handle this type of large data with one call because of some memory issue.
But laravel provides a very helpful functionality for how to handle this type of issue and run time manage memory help of LazyCollection and cursor.
Here, i provide a simple code for how to export/download a very large CSV in 10sec.
I test this example with the 10M records.
Step - 1 : Create Route
In the first step, we need to create one route in our laravel route file.
// Route for export csv
Route::get('download-csv', [ExportCSVController::class, 'download'])->name('download-csv');
Step - 2 : Create Controller
Now, we need to create ExportCSVController.php
file in app/Http/Controllers
directory and write there the following code.
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\User;
class ExportCSVController extends Controller
{
public function download(Request $request)
{
// get the request value
$input = request()->all();
// set header
$columns = [
'Name',
'Email',
'Phone No.',
'Created Date',
];
// create csv
return response()->streamDownload(function() use($columns, $input) {
$file = fopen('php://output', 'w+');
fputcsv($file, $columns);
$data = User::select(
'name',
'email',
'phone_no',
'created_at'
)
->orderBy('id', 'desc');
$data = $data->cursor()
->each(function ($data) use ($file) {
$data = $data->toArray();
fputcsv($file, $data);
});
fclose($file);
}, 'AllUsersCSV'.date('d-m-Y').'.csv');
}
}
Help of above code you can download million of records with a single click.
i hope you like this article.

Hi, My name is Harsukh Makwana. i have been work with many programming language like php, python, javascript, node, react, anguler, etc.. since last 5 year. if you have any issue or want me hire then contact me on [email protected]
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK