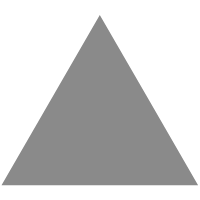
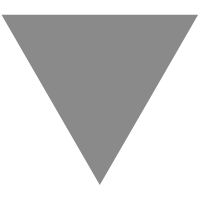
NonZeroU64 in core::num - Rust
source link: https://doc.rust-lang.org/stable/core/num/struct.NonZeroU64.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
pub const fn leading_zeros(self) -> u32
Returns the number of leading zeros in the binary representation of self
.
On many architectures, this function can perform better than leading_zeros()
on the underlying integer type, as special handling of zero can be avoided.
Examples
Basic usage:
let n = std::num::NonZeroU64::new(u64::MAX).unwrap();
assert_eq!(n.leading_zeros(), 0);
Runpub const fn trailing_zeros(self) -> u32
Returns the number of trailing zeros in the binary representation
of self
.
On many architectures, this function can perform better than trailing_zeros()
on the underlying integer type, as special handling of zero can be avoided.
Examples
Basic usage:
let n = std::num::NonZeroU64::new(0b0101000).unwrap();
assert_eq!(n.trailing_zeros(), 3);
Runnonzero_ops
 #84186)Add an unsigned integer to a non-zero value.
Check for overflow and return None
on overflow
As a consequence, the result cannot wrap to zero.
Examples
#![feature(nonzero_ops)]
let one = NonZeroU64::new(1)?;
let two = NonZeroU64::new(2)?;
let max = NonZeroU64::new(u64::MAX)?;
assert_eq!(Some(two), one.checked_add(1));
assert_eq!(None, max.checked_add(1));
Runnonzero_ops
 #84186)Add an unsigned integer to a non-zero value.
Return u64::MAX
on overflow.
Examples
#![feature(nonzero_ops)]
let one = NonZeroU64::new(1)?;
let two = NonZeroU64::new(2)?;
let max = NonZeroU64::new(u64::MAX)?;
assert_eq!(two, one.saturating_add(1));
assert_eq!(max, max.saturating_add(1));
Runnonzero_ops
 #84186)Add an unsigned integer to a non-zero value,
assuming overflow cannot occur.
Overflow is unchecked, and it is undefined behaviour to overflow
even if the result would wrap to a non-zero value.
The behaviour is undefined as soon as
self + rhs > u64::MAX
.
Examples
#![feature(nonzero_ops)]
let one = NonZeroU64::new(1)?;
let two = NonZeroU64::new(2)?;
assert_eq!(two, unsafe { one.unchecked_add(1) });
Runnonzero_ops
 #84186)Returns the smallest power of two greater than or equal to n.
Check for overflow and return None
if the next power of two is greater than the type’s maximum value.
As a consequence, the result cannot wrap to zero.
Examples
#![feature(nonzero_ops)]
let two = NonZeroU64::new(2)?;
let three = NonZeroU64::new(3)?;
let four = NonZeroU64::new(4)?;
let max = NonZeroU64::new(u64::MAX)?;
assert_eq!(Some(two), two.checked_next_power_of_two() );
assert_eq!(Some(four), three.checked_next_power_of_two() );
assert_eq!(None, max.checked_next_power_of_two() );
RunRecommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK