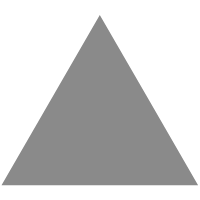
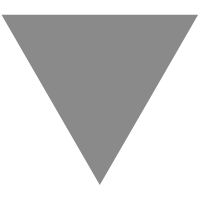
Multiple Inheritance in Java
source link: https://dzone.com/articles/multiple-inheritance-in-java
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Multiple Inheritance in Java
Learn more about multiple inheritance and the use of default interface methods in multiple inheritance.
Join the DZone community and get the full member experience.
Join For FreeEver since Java 8 introduced default interface methods, I felt there had to be a way to use it for multiple inheritance. I have never needed it, but I was bored for a bit today, so decided to try the following idea:
- Create a non-public class
XData
to hold the fields the interface needs to work with, and a public interfaceX
in the sameX.java
source file - The interface has one virtual method
getXData()
that returns theXData
instance - The remaining interface methods are default methods that call
getXData()
to read and write the fields as necessary to perform some useful operations. - Create another interface
Y
and classYData
in the same pattern - Create a class
XY
that implements both interfacesX
andY
- The class
XY
hasXData
andYData
fields, which are returned bygetXData()
andgetYData()
. These are the only two interface methodsXY
is required to implement. - I didn't bother in my example, but
XY
would have to decide how to implement the Object methodshashCode
,equals
, andtoString
. These methods cannot be implemented by interfaces (but they could be implemented inXData
andYData
classes if desired)
The end result is the XY
class is an instance of both X
and Y
interfaces, and inherits the encapsulated behaviors of both X
and Y
default methods - multiple inheritance by any reasonable measure.
It is better than C++ multiple inheritance, in that all constructor logic and declared fields are in the XY
class. The only problem you could get is if X
and Y
interfaces declare the same method that differs only by return type - but this is just a general problem of implementing multiple interfaces.
Here are some code examples using Dog
and Cow
interfaces.
Dog.java:
package mi;
import static java.time.temporal.ChronoField.DAY_OF_MONTH;
import static java.time.temporal.ChronoField.HOUR_OF_DAY;
import static java.time.temporal.ChronoField.MINUTE_OF_DAY;
import static java.time.temporal.ChronoField.MONTH_OF_YEAR;
import static java.time.temporal.ChronoField.NANO_OF_SECOND;
import static java.time.temporal.ChronoField.SECOND_OF_MINUTE;
import static java.time.temporal.ChronoField.YEAR;
import java.time.Instant;
import java.time.OffsetDateTime;
import java.time.ZoneOffset;
/**
* The fields of data that Foo needs to operate on
*/
class DogData {
final String breed;
final String name;
final OffsetDateTime birthDate;
DogData(String breed, String name, OffsetDateTime birthDate) {
this.breed = breed;
this.name = name;
this.birthDate = birthDate;
}
}
public interface Dog {
/**
* The method the class has to implement to return a DogData instance, from one its own fields.
*
* @return dog data
*/
DogData getDogData();
/**
* Return the next time the dog should go to the vet for a checkup,
* which is annually, one week after the dog's birthday.
*
* @return date of next checkup.
*/
default OffsetDateTime nextCheckup() {
final OffsetDateTime now = OffsetDateTime.now();
final OffsetDateTime today = now.
minusHours(now.get(HOUR_OF_DAY)).
minusMinutes(now.get(MINUTE_OF_DAY)).
minusSeconds(now.get(SECOND_OF_MINUTE)).
minusSeconds(now.get(NANO_OF_SECOND));
final OffsetDateTime bday = getDogData().birthDate;
final int thisYear = now.get(YEAR);
final int bdayMonth = bday.get(MONTH_OF_YEAR);
final int bdayDay = bday.get(DAY_OF_MONTH);
OffsetDateTime nextCheckup = OffsetDateTime.
of(thisYear, bdayMonth, bdayDay, 12, 0, 0, 0, ZoneOffset.UTC).
plusDays(7);
if (nextCheckup.isBefore(today)) {
nextCheckup = nextCheckup.plusYears(1);
}
return nextCheckup;
}
default String getBreed() {
return getDogData().breed;
}
default String getName() {
return getDogData().name;
}
default OffsetDateTime getBirthDate() {
return getDogData().birthDate;
}
}
Cow.java:
package mi;
import static java.time.temporal.ChronoField.DAY_OF_MONTH;
import static java.time.temporal.ChronoField.HOUR_OF_DAY;
import static java.time.temporal.ChronoField.MINUTE_OF_HOUR;
import static java.time.temporal.ChronoField.MONTH_OF_YEAR;
import static java.time.temporal.ChronoField.NANO_OF_SECOND;
import static java.time.temporal.ChronoField.SECOND_OF_MINUTE;
import static java.time.temporal.ChronoField.YEAR;
import java.time.Instant;
import java.time.OffsetDateTime;
import java.time.ZoneOffset;
class CowData {
String breed;
OffsetDateTime birthDate;
CowData(String breed, OffsetDateTime birthDate) {
this.breed = breed;
this.birthDate = birthDate;
}
}
public interface Cow {
/**
* The method the class has to implement to return a CowData instance, from one its own fields.
*
* @return cow data
*/
CowData getCowData();
/**
* Return the next time the cow should go to the vet for a shot,
* which is annually, one week before the cow's birthday.
*
* @return date of next checkup.
*/
default OffsetDateTime nextCheckup() {
final OffsetDateTime now = OffsetDateTime.now();
final OffsetDateTime today = now.
minusHours(now.get(HOUR_OF_DAY)).
minusMinutes(now.get(MINUTE_OF_HOUR)).
minusSeconds(now.get(SECOND_OF_MINUTE)).
minusNanos(now.get(NANO_OF_SECOND));
final OffsetDateTime bday = getCowData().birthDate;
final int thisYear = now.get(YEAR);
final int bdayMonth = bday.get(MONTH_OF_YEAR);
final int bdayDay = bday.get(DAY_OF_MONTH);
OffsetDateTime nextCheckup = OffsetDateTime.
of(thisYear, bdayMonth, bdayDay, 12, 0, 0, 0, ZoneOffset.UTC).
minusDays(7);
if (nextCheckup.isBefore(today)) {
nextCheckup = nextCheckup.plusYears(1);
}
return nextCheckup;
}
default String getBreed() {
return getCowData().breed;
}
default OffsetDateTime getBirthDate() {
return getCowData().birthDate;
}
}
DogCow.java:
package mi;
import static java.time.temporal.ChronoField.YEAR;
import java.time.OffsetDateTime;
import java.time.ZoneOffset;
public class DogCow implements Dog, Cow {
private final OffsetDateTime dogCowBdate = OffsetDateTime.of(
OffsetDateTime.now().get(YEAR) - (int)(Math.random() * 5),
(int)(Math.random() * 12) + 1,
(int)(Math.random() * 28) + 1,
(int)(Math.random() * 24),
(int)(Math.random() * 60),
0,
0,
ZoneOffset.UTC
);
private final DogData dogData = new DogData(
"Border Collie",
"Nate",
dogCowBdate
);
private final CowData cowData = new CowData(
"Angus",
dogCowBdate
);
@Override
public DogData getDogData() {
return dogData;
}
@Override
public CowData getCowData() {
return cowData;
}
@Override
public OffsetDateTime nextCheckup() {
// Check both Dog and Cow, choose whichever one comes first
final OffsetDateTime nextDogCheckup = Dog.super.nextCheckup();
final OffsetDateTime nextCowCheckup = Cow.super.nextCheckup();
return nextDogCheckup.isBefore(nextCowCheckup) ? nextDogCheckup : nextCowCheckup;
}
@Override
public String getBreed() {
return Dog.super.getBreed() + " " + Cow.super.getBreed();
}
@Override
public OffsetDateTime getBirthDate() {
return Dog.super.getBirthDate();
}
}
Main.java:
package mi;
public class Main {
public static void main(String[] args) {
for (int i = 1; i < 10; i++) {
final DogCow dc = new DogCow();
System.out.println(
"DogCow " + i +
" is combined breed " +
dc.getBreed() +
" named " +
dc.getName() +
" born on " +
dc.getBirthDate() +
" with next checkup of " +
dc.nextCheckup()
);
}
}
}
Example Main.java output:
DogCow 1 is combined breed Border Collie Angus named Nate born on 2022-11-22T21:40Z with next checkup of 2022-11-15T12:00Z
DogCow 2 is combined breed Border Collie Angus named Nate born on 2018-09-22T01:53Z with next checkup of 2022-09-15T12:00Z
DogCow 3 is combined breed Border Collie Angus named Nate born on 2022-01-26T18:08Z with next checkup of 2022-02-02T12:00Z
DogCow 4 is combined breed Border Collie Angus named Nate born on 2022-02-23T00:32Z with next checkup of 2022-03-02T12:00Z
DogCow 5 is combined breed Border Collie Angus named Nate born on 2019-03-27T04:24Z with next checkup of 2022-03-20T12:00Z
DogCow 6 is combined breed Border Collie Angus named Nate born on 2022-02-04T19:11Z with next checkup of 2022-02-11T12:00Z
DogCow 7 is combined breed Border Collie Angus named Nate born on 2020-02-20T17:13Z with next checkup of 2022-02-27T12:00Z
DogCow 8 is combined breed Border Collie Angus named Nate born on 2018-09-02T18:02Z with next checkup of 2022-08-26T12:00Z
DogCow 9 is combined breed Border Collie Angus named Nate born on 2018-01-07T21:02Z with next checkup of 2022-01-14T12:00Z
See the Java code
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK