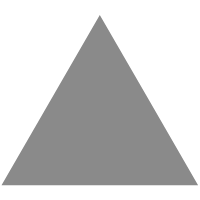
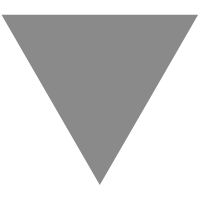
JSON.parse() Reviver for Dates
source link: https://cwestblog.com/2022/02/07/json-parse-reviver-for-dates/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Did you know that you can pass a function as the second parameter to JSON.parse()
? Doing this causes every key/value pair to be passed to this function for further processing. You can read all about how JSON.parse()
and the reviver function works here on MDN’s site.
Example Reviver
We can actually leverage this functionality to turn all date strings into proper Date
objects in JavaScript. The following is the JavaScript function that we can use as our “reviver” function:
/**
* The reviver function that can be passed as the second argument to
* `JSON.parse()` so that all date strings will be parsed as proper dates.
*/
function
dateReviver(key, value) {
// If the value is a string and if it roughly looks like it could be a
// JSON-style date string go ahead and try to parse it as a Date object.
if
(
'string'
===
typeof
value && /^\d{4}-[01]\d-[0-3]\dT[012]\d(?::[0-6]\d){2}\.\d{3}Z$/.test(value)) {
var
date =
new
Date(value);
// If the date is valid then go ahead and return the date object.
if
(+date === +date) {
return
date;
}
}
// If a date was not returned, return the value that was passed in.
return
value;
}
How To Use It
In order to use it, it is really as simple as passing it as the second argument to JSON.parse()
. Here is an example showing how to use the reviver function:
var
dtNow =
new
Date();
var
jsonDtNow = JSON.stringify(dtNow);
var
parsedDt = JSON.parse(jsonDtNow, dateReviver);
console.log(dtNow.getTime() === parsedDt.getTime());
If you run the dateReviver
along with the above code you will see that the date/time values of dtNow
and parsedDt
are exactly the same. This same concept works for nested structures as shown below:
var
person = {
name:
"John Smith"
,
dateOfBirth:
new
Date(1950, 1, 1)
};
var
jsonPerson = JSON.stringify(person);
var
parsedPerson = JSON.parse(jsonPerson, dateReviver);
console.log(person.dateOfBirth.getTime() === parsedPerson.dateOfBirth.getTime());
That’s pretty much all I have to say about JSON.parse()
and the reviver function. Let me know if you have any questions or comments below and as always happy coding!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK