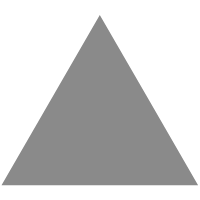
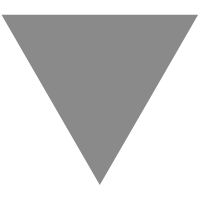
Binary search in std :: vector
source link: https://www.codesd.com/item/binary-search-in-std-vector.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Binary search in std :: vector
I am trying to look for the position of vector elements into another vector. Here i am interested to use an implementation as fast as binary search
. I have different vectors of length 1 million or more, so i am trying to achieve something faster.
Following situations in my case:
1) vector
in which i am searching is sorted.
2) The element i am searching for will always be there i.e i don't have a case of not found
, and i would like to get the index of vector elements in a faster way.
I tried the following code to get the index of vector elements.
#include <iostream>
#include <vector>
#include <algorithm>
template<class Iter, class T>
Iter binary_find(Iter begin, Iter end, T val)
{
Iter i = std::lower_bound(begin, end, val);
return i;
}
int main() {
std::vector<std::string> values = {"AAAAAA","AB", "AD" ,"BCD","CD", "DD" };
std::vector<std::string> tests = {"AB", "CD","AD", "DD"};
for(int i=0 ; i < tests.size(); i++) {
int pos = binary_find(values.begin(), values.end(), tests.at(i))- values.begin();
std::cout << tests.at(i) << " found at: " << pos <<std::endl;
}
return 0;
}
I would like to know if the code matches with the binary search implementation.??
Is there a faster way to get the index of vector elements?
Any further suggestions to improve this code.
binary_find
doesn't return anything despite not declared to return void
, so it has undefined behaviour.
After it is fixed, and assuming that you have no specific knowledge about the contents of the vector other than it is sorted, binary search is pretty much optimal.
There are however, other data structures that are faster for predicate based lookup than a vector. If performance is critical, you should take a look at search trees and hash maps. Since your keys are strings, tries and directed acyclic word graph in particular may be efficient. You may want to measure which is best for your use case.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK