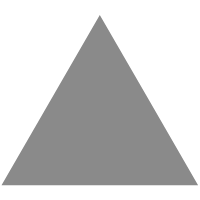
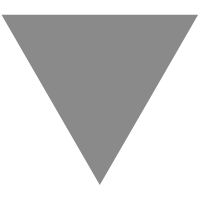
GitHub - garronej/cra-envs: 🛠 Environment variables in create-react-app
source link: https://github.com/garronej/cra-envs
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Use environment variables in create-react-app projects
Safely bundle server environment variables into statically served React SPA.
Motivation
We want to be able to do docker run --env FOO="xyz" my-org/my-create-react-app
then access FOO
in the app like process.env["FOO"]
.
Create react app provides no official way to inject environment variable from the server into the page.
When you run yarn build
create react app does bundle all the variables prefixed by REACT_APP_
and expose them under process.env
(see here).
The problem, however, is that you likely don't want to build your app on the server.
For this use case the CRA team suggests to introduce placeholders in the public/index.html
and do the substitution on the server before serving the app. This solution involves a lot of hard to maintain scripting.
This module abstract away the burden of managing environment variable injection as well as providing a type-safe way to access them in your code.
Step by step guide
Start by installing the tool:
yarn add cra-envs
Then declare all the allowed environment variables into the .env
file of your project
Example
REACT_APP_FOO="Default value of foo" REACT_APP_BAR= REACT_APP_BAZ= REACT_APP_FIZZ=
Once it's done run the script npx generate-env-getter
( Use npx generate-env-getter js
if you your project don't use TypeScript)
It will generate src/env.ts
( or src/env.js
) looking like:
/* * Automatically generated by cra-envs. * If you wish to declare a new environment variable add it in the .env file * then run 'npx generate-env-getter'. This file will be updated. */ import { getEnvVarName } from "cra-envs"; export function getEnv() { return { "FOO": getEnvVarName("FOO"), "BAR": getEnvVarName("BAR"), "BAZ": getEnvVarName("BAZ"), "FIZZ": getEnvVarName("FIZZ") } as const; }
Now let's test it by creating a .env.local
file like:
REACT_APP_BAR="Value of bar defined in .env.local"
And let's do this somewhere in our code:
import { getEnv } from "./path/to/env.ts" console.log(getEnv());
Now if we run REACT_APP_BAZ="Value of baz passed inline" yarn start
we get this
in the console:
{ "FOO": "Default value of foo", "BAR": "Value of bar defined in .env.local", "BAZ": "Value of baz passed inline", "FIZZ": "" }
Now if you run yarn build
then BAZ="Value of baz on the server" npx embed-environnement-variables
the value of BAZ
will be injected in build/index.html
(or html/index.html
) so that if you
start statically serving
the build/
dir, for example with serve -s build
you will get this in the console:
{ "FOO": "Default value of foo", "BAR": "Value of baz on the server", "BAZ": "", "FIZZ": "" }
Note that on the server the environment variable names don't need to be prefixed with REACT_APP_
(they can though).
Also note that the script runs very fast and thus represent virtually no overhead when starting your container.
By default embed-environnement-variables
does not embed variables defined in .env.local
, if you want to include
them use: --includes-.env.local
or -i
.
The next step is to set up a clean Dockerfile where there is both node and Ngnix available.
Node for being able to run npx embed-environnement-variables
and Ngnix for serving the app.
It is also important to make sure cra-envs
is not bootstraped by npx
in the entrypoint.
Clean setup example
Find here a demo setup to help you integrate cra-envs
in your app.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK