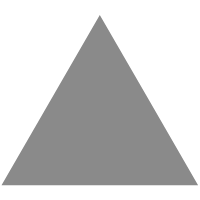
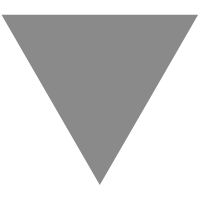
Google Auth/Signin in React Native without Firebase.
source link: https://dev.to/suyashvash/google-authsignin-in-react-native-without-firebase-43n
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Google auth is one of the most commonly used Authentication methods in Mobile and web apps. Well, it is also a tricky one as compared to normal user email and password auth.
In this post, we will learn how to integrate Fully working Google auth with React Native (Without Firebase).
1. Creating you React Native app
Let's start by creating our react-native project.
npx react-native init myapp
Now install the @react-native-google-signin/google-signin
module using npm i @react-native-google-signin/google-signin
in your project folder.
2. Setting up Android environment
After installing the required module, let's set up some android files to make it work properly.
- Update
android/build.gradle
with the following configuration:
Add classpath 'com.google.gms:google-services:4.3.10'
into your dependencies
in buildscript
.
- Update
android/app/build.gradle
with the following configuration:
Add
apply plugin: 'com.android.application'
at the top of your build gradle (android/app).Add
implementation 'com.google.android.gms:play-services-auth:20.0.0'
in dependencies in the same.
implementation "androidx.browser:browser:1.2.0"
`
3. Importing module in our App
Now import the installed module like this
import { GoogleSignin, statusCodes } from '@react-native-google-signin/google-signin';
then after importing the module let's set up a configuration code for our sign-up.
React.useEffect(() => { GoogleSignin.configure({ webClientId: "Your-web-client-id", offlineAccess: true }); }, [])
Let's not worry about the client Id, for now, we will generate it later on in this tutorial.
Now after configuration is done, it's time to make our sign-up function using the Google Auth module we just imported.
const GoogleSingUp = async () => { try { await GoogleSignin.hasPlayServices(); await GoogleSignin.signIn().then(result => { console.log(result) }); } catch (error) { if (error.code === statusCodes.SIGN_IN_CANCELLED) { // user cancelled the login flow alert('User cancelled the login flow !'); } else if (error.code === statusCodes.IN_PROGRESS) { alert('Signin in progress'); // operation (f.e. sign in) is in progress already } else if (error.code === statusCodes.PLAY_SERVICES_NOT_AVAILABLE) { alert('Google play services not available or outdated !'); // play services not available or outdated } else { console.log(error) } } };
Now Connect this function to your button with onPress={GoogleSingUp}
prop
``
4. Generating Web client ID and SHA1 key for Sign up
Now, this is the main part where most of the developer gets stuck or get a pretty common and irritating error -
Error: Status{statusCode=DEVELOPER_ERROR}
But what is causing this error?. It is quite simple, the SHA1 key of Debug Keystore. Yeah, according to google, you have to put your Release Keystore key in Google Console. But most blogs and articles forget to mention this point which is very important no matter which module you are using.
1) Generate Release keystore and it's SHA
Now assuming you have already installed JDK in your system, let's move to generating the Release key. In your android/app run this command in cmd -
keytool -genkey -v -keystore my_release_key.keystore -alias my_key_alias -keyalg RSA -keysize 2048 -validity 10000
It will ask for some detail so fill them in carefully and remember the password you entered in it.
This command will generate a release key in your android/app
directory.
-
Now in your
android/gradle.properties
addMYAPP_UPLOAD_STORE_FILE=my_release_key.keystore MYAPP_UPLOAD_KEY_ALIAS= my_key_alias MYAPP_UPLOAD_STORE_PASSWORD=yourPassword MYAPP_UPLOAD_KEY_PASSWORD=yourPassword
-
And this in your
android/app/build.gradle
signingConfigs { debug { // storeFile file('debug.keystore') // storePassword 'android' // keyAlias 'androiddebugkey' // keyPassword 'android' storeFile file(MYAPP_UPLOAD_STORE_FILE) storePassword MYAPP_UPLOAD_STORE_PASSWORD keyAlias MYAPP_UPLOAD_KEY_ALIAS keyPassword MYAPP_UPLOAD_KEY_PASSWORD } release { if (project.hasProperty('MYAPP_UPLOAD_STORE_FILE')) { storeFile file(MYAPP_UPLOAD_STORE_FILE) storePassword MYAPP_UPLOAD_STORE_PASSWORD keyAlias MYAPP_UPLOAD_KEY_ALIAS keyPassword MYAPP_UPLOAD_KEY_PASSWORD } } }
Now this will generate release SHA as your debug keystore SHA, so you don't have to worry about that error
Now generate release SHA1 using this command in android/app
keytool -list -v -keystore app/my_release_key.keystore -alias my_key_alias
Copy that SHA1 key and paste it somewhere for the next step.
``
2) Inserting SHA1 key in Google Developer Console.
Now, after doing that much hard work, let's do this final part. We have to paste this key into the Google Developer console to tell google about our app and its authentication.
- After signing up in the Google Developer console or Google cloud platform, head over to the Credential tab. There you will find a button saying "Create Credential", choose OAth Client ID.
- Then choose Application type- Android and enter the package name and then the SHA1 key you copied in the previous step. Hit create and your Android API is ready.
- Now Similarly create an OathID for Web client instead of Android and leave all other fields as it is.
- Now copy the Web client ID from the Oath you just created.
That's it we got the Web client ID we needed for Step 3 above.
5. Finalizing
Now paste this Web Client ID into your Google config object in Step 3 above and run the app.
After pressing the Sign-in Button, A pop-up should appear. Select the account you want to login with and after selecting the account it will return an object containing IdToken and some other stuff.
If you did everything exactly the same and didn't mess up anywhere, we should see some results like this
{ "idToken": "Your-google-auth-token", "scopes": [ "https://www.googleapis.com/auth/userinfo.email", "https://www.googleapis.com/auth/userinfo.profile" ], "serverAuthCode": "your-server-auth-code", "user": { "email": "[email protected]", "familyName": "your-last-name", "givenName": "your-first-name", "id": "some-id", "name": "your-full-name", "photo": "a-url-for-profile-photo" } }
And with that, we successfully integrated the Google auth into our React Native app.
Please leave a comment if you liked the post or just want to add something valuable.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK