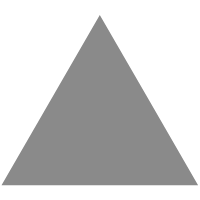
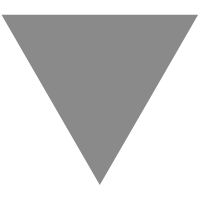
A guide to Animations in Angular
source link: https://blog.knoldus.com/a-guide-to-animations-in-angular/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Introduction
Animations are an important part of your website. It helps us in enhancing the user experience and also calls the user’s attention where it is needed. Animations are created by transforming the styles over time whose timing you can control. In this blog, I will be covering the basics of angular animations and an example of it to understand the concept better.
Animations in Angular
Animations can help you in making a beautiful web design. If you are defining well-structured animations, you can engage the user on your website. An animation can be defined as a transition from one state to another. The angular animation system is built on CSS functionality. In angular, we define different states. For each state, define styles and then we switch between those states to create the animation effect. This is the basic idea of animations in Angular.
States in Angular Animations
Angular provides three states which are listed below:
- Void: this is the state where the element is not yet part of the DOM or removed from DOM.
- Wildcard(*): This is the default state of the element.
- Custom: This state needs to be defined explicitly and we can use any name for it.
Functions in Angular Animations
Now, here are some important Functions used in creating animations.
- State: This function accepts a custom state name and the particular styles for it.
- Trigger: This function includes an array of the states and transitions.
- Style: It includes all the CSS styles which will be applied to the particular state.
- Transition: This function accepts two states between which we want to make transitions.
- Animate: This function defines the length of the transition state and easing of a transition.
Now when you have an overview of all the functions, we can better understand them with the help of an example.
Prerequisites
Firstly, create an Angular project using the CLI command
ng new animationsDemo
Now open the project in your favourite editor and this is how the directory structure looks.
In order to use animations in your angular project, we need to Import the BrowserAnimationsModule in your imports array of the module file(app.module.ts).
import {BrowserAnimationsModule} from '@angular/platform-browser/animations';
imports: [
BrowserModule,
AppRoutingModule,
BrowserAnimationsModule
]
Now we move to the component in which we want to use it and add the following code:
import {
trigger,
state,
style,
animate,
transition,
// ...
} from '@angular/animations';
In order to use these, we are importing them.
In the @Component decorator, we generally have three things. But for animations, we are going to add one like this:
@Component({
selector: 'app-root',
templateUrl: 'app.component.html',
styleUrls: ['app.component.css'],
animations: [
// animation triggers go here
]
})
Demo Example
First, let us create a simple component in which we will apply some transitions. Here is the app.component.html file
<div class="container">
<h3 class="mt-3">Animations Demo in Angular</h3>
<div class="item1">
</div>
<button type="button" class="btn btn-primary mt-3">Click me</button>
</div>
Now let us define the animations. We will be defining a simple animation in which we will be changing the div size and color while moving from one state to another in app.component.ts file.
animations: [
trigger('changeDivStyle', [
state('start', style({
width: '150px',
height:'100px',
background:'blue',
transform:'translateX(0)'
})),
state('end', style({
width: '300px',
height:'150px',
background:'green',
transform:'translateX(50%)'
})),
transition('start<=>end', animate('1500ms'))
]),
]
Here we can see we have defined two states, the start, and the end. You can name it anything. For each state, we have defined some CSS. You can use all the CSS properties inside the style(). Since we will be moving the div in the horizontal direction, I have used the transform property.
And after defining the states, we set the transition time. Here we are setting it to 1500ms which is 1.5 secs. You can change the speed according to your needs. The arrow <=> represents that from start to end or end to start state transition will take 1.5 seconds. If you want to define separately, you can do it like this:
transition('start=>end', animate('1500ms')),
transition('end=>start', animate('2000ms'))
Now, set the initial state like this in the class so that the CSS is applied to that div for the starting state.
export class AppComponent {
title = 'AnimationsDemo';
initialState='start';
}
Now to apply this, we need to use the trigger name with ‘@’ on the HTML element we want.
<div [@triggerName]="expression">...</div>;
The expression here is the initial state we want.
Make the following changes in the div element:
<div class="item1" [@changeDivStyle]=initialState>
We will trigger the animation on the button click, so make the following changes on the button:
<button type="button" class="btn btn-primary mt-3" (click)="toggleState()">Click me</button>
Here we are calling a function toggleState, which will change the state for the animation. So, let us now define this function in the ts file.
toggleState() {
this.initialState = this.initialState === 'start' ? 'end' : 'start';
}
Here we will be checking if the current state is ‘start’ then change it to end, otherwise back to ‘start’ state.
Now you are ready to run your project and you will see the animation working.
Conclusion
So far we have learned all the functions required for creating animations and how we trigger those animations. This was a basic example covering the concept of animations in Angular. You can also create complex animations. To learn about the advanced features, you can refer here. Remember using well-structured animations can give you a better user experience.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK