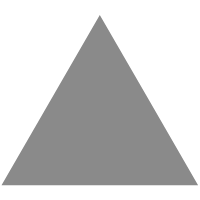
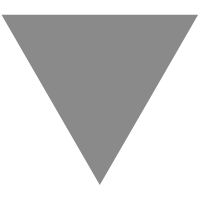
Drag N Drop using Angular 7
source link: https://blog.knoldus.com/drag-n-drop-using-angular-7/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Drag N Drop using Angular 7
Reading Time: 4 minutes
Drag and Drop is one of the new features which is provided by Angular 7. Angular 7 makes it very easy to implement this feature. So here, in this blog, we are going to learn how to drag and drop the items from a list of numbers using a basic example.
Before getting started with the implementation there are some basic pre-requisites which is necessary to be met like if you are still using Angular CLI 6, then, in that case, you have to upgrade your Angular CLI version to Angular CLI 7.
You can check the version of Angular CLI you are using in your system with just one command given below:
ng version
Now one more the basic necessity for implementing Drag and Drop is to install Angular CDK, Animation. You can install this using single command given below:
npm i –save @angular/cdk @angular/animations
So now we are all set to start with the implementation as we already installed all the dependencies.
1. Firstly we need to import the drag and drop module in app.module.ts.
import { BrowserModule } from '@angular/platform-browser'; import { NgModule } from '@angular/core'; import { AppRoutingModule } from './app-routing.module'; import { AppComponent } from './app.component'; import {DragDropModule} from '@angular/cdk/drag-drop'; @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, AppRoutingModule, DragDropModule, ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
2. Now switch to its component file i.e app.component.ts file where we write the business logic. Here, first add numbers(items) in an array like shown below:
import { Component } from '@angular/core'; import { CdkDragDrop } from '@angular/cdk/drag-drop'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent { title = 'Drag-Drop Tutorials'; allNumbers: number[] = []; constructor() { for (let insertNumbers = 0; insertNumbers <= 100; insertNumbers++) { this.allNumbers.push(insertNumbers); } } }
In the above code, we declare an array name allNumbers in which we push or insert the numbers in an array allNumbers from 0 to 100.
3. To display a list of all the number on user Interface we need to write HTML code for that in the app.component.html file.
In this HTML file, we include cdkDroplist and everything here we will do it under cdkDropList. Also, another thing we add here is cdkDrag which helps in dragging the elements to change its place. and for giving some attractive look we write some CSS to it in an app.component.css file to give some designing.
app.component.css
.display-list-of-numbers { height: 100px; width: 100%; border: 3px solid dodgerblue; display: inline-flex; align-items: center; justify-content: center; }
4. So now we need a cdkDropList method with the prefix dropped and providing a drop event here to the method. which ensure the proper drag and drop mechanism, between the list of elements.
5. You can see in the above code there is one method drop($event) which is responsible for drop an item. To define the drop method we need to write the definition of this method in its ts file.
drop(event: CdkDragDrop<number[]>) { moveItemInArray(this.allNumbers, event.previousIndex, event.currentIndex); }
In this code, we define a method name drop and passing a drop event of CdkDragDrop event provided by Angular CDK dependency and pass an array of numbers in it.
6. Moving further we call a built-in method inside the drop method i.e moveItemInArray which is a part of CDK and we are going to pass their 3 things here:
– number array
– prev index
– current index
And, these three things are enough to move the index of an item as you can see the result in the below image.
Now you can see that the number 0 is shifted or dragged in place of 2 and its position is changed as compared with the previous image.
Conclusion: After following this blog, I hope you will be able to drag and drop any element from the list of numbers using Angular 7 with just following the few simple steps.
Thanks For Reading!!!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK