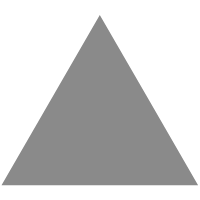
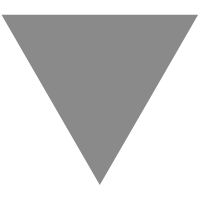
React Responsive Animated Navbar Example
source link: https://www.laravelcode.com/post/react-responsive-animated-navbar-example
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
React Responsive Animated Navbar Example
In this article, I will share with you how to create a responsive animated in react application and how to built your navbar in your react application. in all applications, we want to make one navbar and there show some important links called a menu. so how to make it in your first react application. here I will show you how to create a simple responsive animated navbar in react application and how to built your navbar functionality in your react application.
You can also download all code from GitHub.
Preview
Create React App
First we need to create our react application using the following command in terminal
sudo npx create-react-app navbar --use-npm
After done or create React.js application in your local system. application structure looks like bellow.
navbar
├── node_modules
├── public
│ ├── favicon.ico
│ ├── index.html
│ └── manifest.json
├── src
│ ├── App.css
│ ├── App.js
│ ├── App.test.js
│ ├── index.css
│ ├── index.js
│ ├── logo.svg
│ └── serviceWorker.js
├── .gitignore
├── package.json
└── README.md
make the above files folder structure like that and remove un-necessary files and older.
navbar
├── node_modules
├── public
│ ├── favicon.ico
│ ├── index.html
│ └── manifest.json
├── src
│ ├── components
│ ├── navbar
│ ├── Brand.js
│ ├── BurgerMenu.js
│ ├── CollapseMenu.js
│ ├── Navebar.js
│ ├── style
│ ├── Global.js
│ ├── App.css
│ ├── App.js
│ ├── App.test.js
│ ├── index.css
│ ├── index.js
│ ├── logo.svg
│ └── serviceWorker.js
├── .gitignore
├── package.json
└── README.md
Install-Package
after the create one fresh react application then install the following package in your react application's root directory.
npm install react-spring styled-components
// OR
nmp install react-spring
nmp install styled-components
you can also install packages in both ways.
Create Global.js
First, we need to create a Global.js file in the following directory path.
src/style/Global.js
import { createGlobalStyle } from 'styled-components';
const GlobalStyles = createGlobalStyle`
@import url('https://fonts.googleapis.com/css?family=Montserrat:400,600&display=swap');;
*,
*::after,
*::before {
margin: 0px;
padding: 0px;
box-sizing: inherit;
}
html {
font-size: 62.5%;
}
body {
box-sizing: border-box;
font-family: 'Montserrat', sans-serif;
}
`;
export default GlobalStyles;
Create Brand.js
src/components/navbar/Brand.js
import React from 'react'
import styled from "styled-components";
import logo from "../../logo.svg";
const Brand = () => {
return (
<Image src={logo} alt="Company Logo" />
)
}
export default Brand
const Image = styled.img`
height: 85%;
margin: auto 0;
`;
Create BurgerMenu.js
src/components/navbar/BurgerMenu.js
import React from 'react';
import styled from "styled-components";
const Burgermenu = (props) => {
return (
<Wrapper onClick={props.handleNavbar}>
<div className={ props.navbarState ? "open" : "" }>
<span> </span>
<span> </span>
<span> </span>
</div>
</Wrapper>
);
}
export default Burgermenu;
const Wrapper = styled.div`
position: relative;
padding-top: .7rem;
cursor: pointer;
display: block;
& span {
background: #fdcb6e;
display: block;
position: relative;
width: 3.5rem;
height: .4rem;
margin-bottom: .7rem;
transition: all ease-in-out 0.2s;
}
.open span:nth-child(2) {
opacity: 0;
}
.open span:nth-child(3) {
transform: rotate(45deg);
top: -11px;
}
.open span:nth-child(1) {
transform: rotate(-45deg);
top: 11px;
}
`;
Create CollapseMenu.js
src/components/navbar/CollapseMenu.js
import React from 'react';
import styled from 'styled-components';
import { useSpring, animated } from 'react-spring';
const CollapseMenu = (props) => {
const { open } = useSpring({ open: props.navbarState ? 0 : 1 });
if (props.navbarState === true) {
return (
<CollapseWrapper style={{
transform: open.interpolate({
range: [0, 0.2, 0.3, 1],
output: [0, -20, 0, -200],
}).interpolate(openValue => `translate3d(0, ${openValue}px, 0`),
}}
>
<NavLinks>
<li><a href="/" onClick={props.handleNavbar}>link n1</a></li>
<li><a href="/" onClick={props.handleNavbar}>link n2</a></li>
<li><a href="/" onClick={props.handleNavbar}>link n3</a></li>
<li><a href="/" onClick={props.handleNavbar}>link n4</a></li>
</NavLinks>
</CollapseWrapper>
);
}
return null;
};
export default CollapseMenu;
const CollapseWrapper = styled(animated.div)`
background: #2d3436;
position: fixed;
top: 4.5rem;
left: 0;
right: 0;
`;
const NavLinks = styled.ul`
list-style-type: none;
padding: 2rem 1rem 2rem 2rem;
& li {
transition: all 300ms linear 0s;
}
& a {
font-size: 1.4rem;
line-height: 2;
color: #dfe6e9;
text-transform: uppercase;
text-decoration: none;
cursor: pointer;
&:hover {
color: #fdcb6e;
border-bottom: 1px solid #fdcb6e;
}
}
`;
Create Navbar.js
src/components/navbar/Navbar.js
import React from 'react'
import styled from "styled-components";
import { useSpring, animated, config } from "react-spring";
import Brand from "./Brand";
import BurgerMenu from "./BurgerMenu";
import CollapseMenu from "./CollapseMenu";
const Navbar = (props) => {
const barAnimation = useSpring({
from: { transform: 'translate3d(0, -10rem, 0)' },
transform: 'translate3d(0, 0, 0)',
});
const linkAnimation = useSpring({
from: { transform: 'translate3d(0, 30px, 0)', opacity: 0 },
to: { transform: 'translate3d(0, 0, 0)', opacity: 1 },
delay: 800,
config: config.wobbly,
});
return (
<>
<NavBar style={barAnimation}>
<FlexContainer>
<Brand />
<NavLinks style={linkAnimation}>
<a href="/">link n1</a>
<a href="/">link n2</a>
<a href="/">link n3</a>
<a href="/">link n4</a>
</NavLinks>
<BurgerWrapper>
<BurgerMenu
navbarState={props.navbarState}
handleNavbar={props.handleNavbar}
/>
</BurgerWrapper>
</FlexContainer>
</NavBar>
<CollapseMenu
navbarState={props.navbarState}
handleNavbar={props.handleNavbar}
/>
</>
)
}
export default Navbar
const NavBar = styled(animated.nav)`
position: fixed;
width: 100%;
top: 0;
left: 0;
background: #2d3436;
z-index: 1;
font-size: 1.4rem;
`;
const FlexContainer = styled.div`
max-width: 120rem;
display: flex;
margin: auto;
padding: 0 2rem;;
justify-content: space-between;
height: 5rem;
`;
const NavLinks = styled(animated.ul)`
justify-self: end;
list-style-type: none;
margin: auto 0;
& a {
color: #dfe6e9;
text-transform: uppercase;
font-weight: 600;
border-bottom: 1px solid transparent;
margin: 0 1.5rem;
transition: all 300ms linear 0s;
text-decoration: none;
cursor: pointer;
&:hover {
color: #fdcb6e;
border-bottom: 1px solid #fdcb6e;
}
@media (max-width: 768px) {
display: none;
}
}
`;
const BurgerWrapper = styled.div`
margin: auto 0;
@media (min-width: 769px) {
display: none;
}
`;
Change in App.js
src/App.js
import React, { Component } from 'react'
import Navbar from "./components/navbar/Navbar";
import GlobalStyle from './style/Global';
class App extends Component {
state = {
navbarOpen: false
}
handleNavbar = () => {
this.setState({ navbarOpen: !this.state.navbarOpen });
}
render() {
return (
<>
<Navbar
navbarState={this.state.navbarOpen}
handleNavbar={this.handleNavbar}
/>
<GlobalStyle />
</>
)
}
}
export default App
i hope you like this article. if you want to download code then click here GitHub.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK