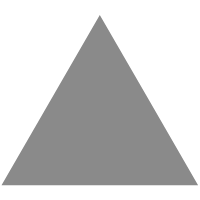
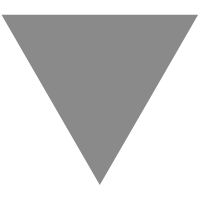
How to build a diagonal matrix using a 2d network in numpy?
source link: https://www.codesd.com/item/how-to-build-a-diagonal-matrix-using-a-2d-network-in-numpy.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
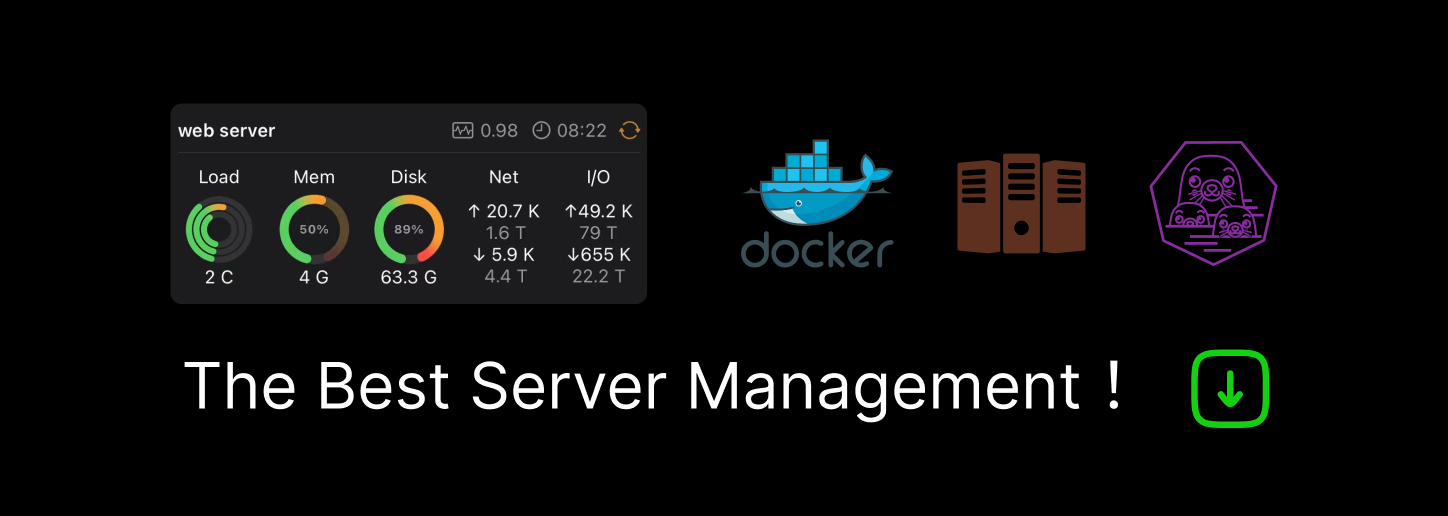
How to build a diagonal matrix using a 2d network in numpy?
Using np.diag
I'm able to construct a 2-D array where a 1-D array is input is returned on the diagonal. But how to do the same if I have n-D array as input?
This works
foo = np.random.randint(2, size=(36))
print foo
print np.diag(foo)
[1 1 1 1 1 1 0 1 0 0 1 1 0 1 1 1 1 1 0 0 0 0 0 0 0 1 0 1 0 1 0 0 0 1 1 0]
[[1 0 0 ..., 0 0 0]
[0 1 0 ..., 0 0 0]
[0 0 1 ..., 0 0 0]
...,
[0 0 0 ..., 1 0 0]
[0 0 0 ..., 0 1 0]
[0 0 0 ..., 0 0 0]]
This won't
foo = np.random.randint(2, size=(2,36))
print foo
[[1 1 0 1 1 0 1 1 1 1 1 0 0 0 0 0 1 0 0 1 0 0 0 0 1 0 0 0 1 0 0 0 0 1 0 0]
[0 1 1 1 0 0 1 1 1 1 0 1 0 1 0 1 0 0 0 0 1 0 1 1 1 1 0 0 1 0 1 1 1 1 0 1]]
do_something(foo)
Should return
array([[[ 1., 0., 0., ..., 0., 0., 0.],
[ 0., 1., 0., ..., 0., 0., 0.],
[ 0., 0., 0., ..., 0., 0., 0.],
...,
[ 0., 0., 0., ..., 1., 0., 0.],
[ 0., 0., 0., ..., 0., 0., 0.],
[ 0., 0., 0., ..., 0., 0., 0.]],
[[ 0., 0., 0., ..., 0., 0., 0.],
[ 0., 1., 0., ..., 0., 0., 0.],
[ 0., 0., 1., ..., 0., 0., 0.],
...,
[ 0., 0., 0., ..., 1., 0., 0.],
[ 0., 0., 0., ..., 0., 0., 0.],
[ 0., 0., 0., ..., 0., 0., 1.]]])
EDIT
Some test based on answers of Alan and ajcr in this post and Saulo Castro and jaime where ajcr refers to. As usual, it all depends on your input. My input has usually the following shape:
M = np.random.randint(2, size=(1000, 36))
with the functions as follows:
def Alan(M):
M = np.asarray(M)
depth, size = M.shape
x = np.zeros((depth,size,size))
for i in range(depth):
x[i].flat[slice(0,None,1+size)] = M[i]
return x
def ajcr(M):
return np.eye(M.shape[1]) * M[:,np.newaxis,:]
def Saulo(M):
b = np.zeros((M.shape[0], M.shape[1], M.shape[1]))
diag = np.arange(M.shape[1])
b[:, diag, diag] = M
return b
def jaime(M):
b = np.zeros((M.shape[0], M.shape[1]*M.shape[1]))
b[:, ::M.shape[1]+1] = M
return b.reshape(M.shape[0], M.shape[1], M.shape[1])
result for me in the following
%timeit Alan(M)
100 loops, best of 3: 2.22 ms per loop
%timeit ajcr(M)
100 loops, best of 3: 5.1 ms per loop
%timeit Saulo(M)
100 loops, best of 3: 4.33 ms per loop
%timeit jaime(M)
100 loops, best of 3: 2.07 ms per loop
def makediag3d(a):
a = np.asarray(a)
depth, size = a.shape
x = np.zeros((depth,size,size))
for i in range(depth):
x[i].flat[slice(0,None,1+size)] = a[i]
return x
Recommend
-
16
第12天。 今天的题目是 Diagonal Traverse : Given a matrix of M x N elements (M rows, N columns), return all elements of the matrix in diag...
-
9
TL/DR: Diagonal layouts are great. You can build them easily with CSS. Take a look at this CodePen to see how it works. Layouts with diagonal sections are q...
-
32
How NumPy Can Simplify Your Code And Increase Its Performance At The Same Time. Broadcasting and SIMD come to rescue!
-
3
Life on the diagonal — adventures in 2-D time by Luke Plant Posted in: — July 19, 2021 12:59
-
9
Never multiply with a large diagonal matrix 4 I love wo...
-
9
The goal In previous posts we’ve talked about how to all with numpy implementations. With these building blocks, we can implement a convolutional neural network (CNN) from...
-
4
In this article, we will learn matrix-vector multiplication using NumPy. Table Of Contents What is a matrix in numpy and how to create it? The numpy stand...
-
7
C++ Program to convert the diagonal elements of the matrix to 0Skip to content C++...
-
11
Anti Diagonal Traversal of MatrixDecember 28, 2023 |500 ViewsPROBLEM OF THE DAY : 27/12/2023 | Anti Diagonal Traversal of MatrixProblem of the Day, matrix, Data Structure and Algorithm
-
2
What is NumPy? Faster array and matrix math in Python Learn how this popular Python library accelerates math at scale, especially when paired with tools...
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK