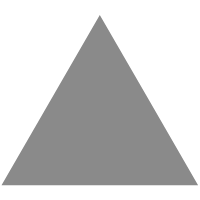
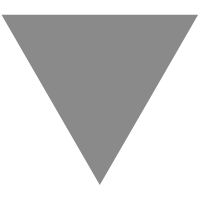
How to Delete By Id in Mongoose
source link: https://masteringjs.io/tutorials/mongoose/delete-by-id
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
How to Delete By Id in Mongoose
There is currently no method called deleteById()
in mongoose.
However, there is the deleteOne()
method with takes a parameter, filter
, which indicates which document to delete.
Simply pass the _id
as the filter
and the document will be deleted.
const testSchema = new mongoose.Schema({
name: String
});
const Test = mongoose.model('Test', testSchema);
async function run() {
const entry = await Test.create({ name: 'Masteringjs.io' });
console.log(await Test.countDocuments({ _id: entry._id })); // 1
// Delete the document by its _id
await Test.deleteOne({ _id: entry._id });
console.log(await Test.countDocuments({ _id: entry._id })); // 0
}
run();
Using an Instance Method
You could also make deleteById()
a Mongoose static on your schema, which will make deleteById()
a function on your model as shown below.
const testSchema = new mongoose.Schema({
name: String
});
testSchema.statics.deleteById = function(_id) {
return this.deleteOne({ _id: _id })
};
const Test = mongoose.model('Test', testSchema);
async function run() {
const entry = await Test.create({ name: 'Masteringjs' });
console.log(await Test.countDocuments({ _id: entry._id })); // 1
await Test.deleteById(entry._id);
console.log(await Test.countDocuments({ _id: entry._id })); // 0
}
Want to become your team's MongoDB expert? "Mastering Mongoose" distills 8 years of hard-earned lessons building Mongoose apps at scale into 153 pages. That means you can learn what you need to know to build production-ready full-stack apps with Node.js and MongoDB in a few days. Get your copy!
More Mongoose Tutorials
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK