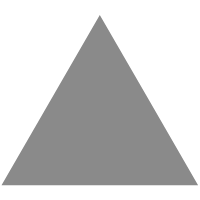
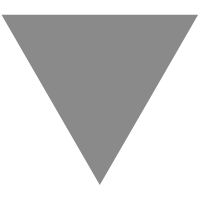
Reactive Programming in Angular | The RxJs way
source link: https://blog.knoldus.com/reactive-programming-in-angular-the-rxjs-way/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Reactive programming is an essential part of modern web apps. However, few popular programming languages come equipped with the reactive API by default (Angular). RxJS allows you to create reactive programs with JavaScript to better serve your users. RxJS is a library used to create asynchronous programs using observable sequences.
Today, we’ll explore an overview of reactive programming and RxJS in Angular.
What is reactive programming?
Almost every online application today generates immense amounts of real-time, interactive data. Applications are expected to make changes across the application in response to events and remain fully functional during the process. The reactive paradigm was made to handle these “events” with real-time updates across the program.
Reactive programs are structured around events rather than sequential top-down execution of iterative code. This allows them to respond to a trigger event regardless of when what stage the program is on.
Reactive programming is often combined with functional programming and concurrency to create stable, scalable, and event-driven programs.
Advantages of reactive programming
The main advantage of reactive programming is that it allows a program to remain responsive to events regardless of the program’s current task.
Other advantages include:
- Highly scalable
- Clean and readable
- Easy to add new event or response support
- Improved user experience due to little downtime
- Helpful community
The reactive paradigm can also be combined with other paradigms to form a blend, such as object-oriented reactive programming (OORP) or functional reactive programming (FRP). This quality makes reactive programming a versatile paradigm that can be altered to fit a variety of purposes.
What is RxJS?
The reactive paradigm is available for many languages through reactive extensions, or rxjs angular. These libraries are downloadable APIs that add support for essential reactive tools like observers and reactive operators. With reactive extensions, developers can convert normally iterative languages like JavaScript, Python, C++, etc, to reactive languages.
RxJS is more specifically a functional reactive programming tool featuring the observer pattern and the iterator pattern. It also includes an adapted form of the JavaScript’s array functions (reduce, map, etc.) to handle asynchronous events as collections.
JavaScript’s Rx-library is called RxJS. RxJS has become very popular because it simplifies JavaScripts async implementation. Without extensions, JavaScript’s async is difficult to use and underdeveloped. RxJS makes async more achievable with tools built specifically for reactive and asynchronous programming.
Many web application frameworks, like Angular , are based on RxJS structures. As a result, you may have indirectly used RxJS already!
Next, we’ll break down the core components of RxJS and show you how to implement them in your own code.
RxJS Observables
Observables are parts of our program that generate data over time. An observable’s data is a stream of values that can then be transmitted synchronously or asynchronously.Stream of data can be anything.It can be a stream of video similar to Youtube videos , or it can be a stream of music just like Spotify.
Consumers can then subscribe to observables to listen to all the data they transmit. Consumers can be subscribed to multiple observables at the same time. This data can then be transformed as it moves through the data pipeline toward the user.
An Observable sets up an observer (we’ll learn more about this) and connects it to the “thing” we want to get values from. This “thing” is called a producer and is a source of values – perhaps from a click
or input
event in the DOM (or even be something more complex such as async logic).
Better mental model for understanding observable is consumer, publisher pattern. Consumers can asynchronously and continuely receive messages as long as subscribed to publishers. Consumers that subscribes to the Observable keeps receiving values until the Observable is completed or the consumer unsubscribes from the observable.
Lets see how we can create our first observable.
import { Injectable } from ‘@angular/core’;
import {Observable} from ‘rxjs’;
@Injectable({
providedIn: ‘root’
})
export class ObservableService {
constructor() { }
public getNewObservable() {
return new Observable((observer) => observer.next(‘Hello My First Observable’);
}
}
RxJS Observer
An observer is an object with next()
, error()
, and complete()
methods that is called when there’s an interaction with the observable. They are the objects that subscribe to observables. Apart from that we have huge bunch of rxjs operators in angular available in this https://rxjs.dev/api/operators
Example : Consider a football game. Many supporters are watching the game. We split the supporters into two categories by age, young and old. When their team scores a goal the supporters react differently according to their age and their excitement level.
- The game is the subject and the supporters are the observers.
- All observers are attached/registered to the subject and they are notified when their football team scores (the trigger-event is if their team scores).
- The observers update their behaviour depending on the notification received.
Wrapping up and next steps
Today we have learned about Reactive Programming in Angular using RxJS. Why and when to opt for RxJs in Angular. What is Observable and how does observer interacts with subscriber.The RxJS library can help you handle async implementation in a flexible and efficient way.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK