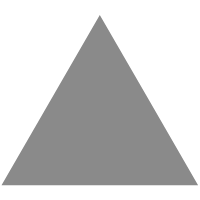
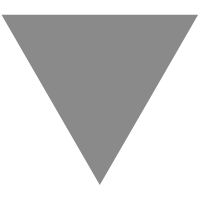
How to create a movie details app using Flutter: part 1
source link: https://medium.com/flutter-community/how-to-create-a-movie-details-app-using-flutter-part-1-7cad1102d07c
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
How to create a movie details app using Flutter: part 1
Hi All, It is nice to be back with a new flutter blog, this is a series of features that will be added to the movie app, and below are the features to be covered in this part.
NB: It is mainly focused on beginner level only.
- Show trending Movies.
- Show Top Rated movies.
- Show upcoming movies.
- Able to search for movies.
- Able to fetch the data from API.
- Use of env variables for storing API keys.
- Show Similar movies.
Cool, now let us get our hands dirty.
We will be splitting the work into chunks.
- Create a flutter app and try out a sample API.
- Add ENV variable using the TMDB API.
- Project Structure
- Implement main.dart
- Create a details page
- Create a top-rated movie component
- Upcoming movie component
- Search movie
1. Create a flutter app and try out a sample API.
Assuming the reader will have the basic idea for creating an app using flutter, I will not going to that part, in our basic main.dart
file we will add the code from flutter.dev. and run our app to check.
2. Add ENV variable using the TMDB API.
It is the most important part, here we will create an account in the tmdb, It is a movie data platform that supports images and movie details, and most of our requirements are covered here. Will use this API for education purposes only. A big thanks to tmdb for trying out the API free of cost. Link
They also provide the link to the trailer video which we will add that later in the tutorial.

After that, we need to test this API is working or not, we can use the same code as existing, but some tweaks are needed.
- Create an ENV file to keep the env key.
- Fetch the key from env and use it in the API.
- Check the response from tmdb API.
1. Create an ENV file to keep the env key.
Since the key from tmdb is personnel to our account, we can’t put that key in the public repository, what will do is install the package to support env files and copy the ENV key to that file. for that, will use the package called flutter_dotenv
. Link
We need to add the key to .env
file and also need to register this file to the pubspec.yaml
then only the flutter will understand.


2. Fetch the key from env and use it in the API.
Now, we have successfully added the API key, we need to use this key when fetching the data. For that, we need to import the flutter_dotenv file to the necessary file.
We Will use the same code, the only change here is the API URL change and the token is used from the env file only.

3. Check the response from tmdb API.
We need to parse the response from the tmdb API. for that, will use the same code as mentioned above.
What we are doing here, once the API is a success, will fetch the title of the movie and display that value in the app’s interface for now. Currently, the id passed in the API 551
is the movie name posieden , we have expected this value after execution.

Awesome, we completed our first step, Kudos, now proceed to the next part.
GitHub commit link
3. Project Structure.
It is better to show a picture rather than explains it a lot.
Important: Please read this section, then only you will get an idea about how the code works.

As you can see we have a main.dart which will load a page which will have 2 pages, the first one is our home page which will have 3 components or sub-pages namely,
- Which are the current trending movies right now.
- Top-rated movies right now.
- upcoming movies right now.
All these data are coming from the tmdb API, we don’t have to manipulate anything here. It will load the data once the API is correctly called.
All these components are actually showing like below,
One thing to understand here is that on the home page, we are showing trending, top-rated and upcoming movies. But all the structure remains the same, so we need to populate only the data based on the page where we stand.
horizontal_cards component.

So, what we need is a horizontal scroll component that was able to scroll to right for more data, this horizondel_cards.dart
will be used in all the places like trending_movies, top_rated_movies and upcoming_movies.
We need to call the API and pass the response to the horizontal cards only, they will display accordingly.
details.dart
On clicking on each movie poster will be redirected to another page where we will display all the details about this movie and another image if available.

on this page, will have another 2 components there. actors.dart
and similar_movies.dart
. I hope you will understand all those details.
similar_movies.dart

This is the part that we are doing in this blog. Hope everyone understands the concepts here, will proceed with the implementation.
4. Implement main.dart
It is the first page when loading this application, as explained above this page will have 2 tabs. Each one has its own components also.
- Load the dependencies
- Convert the class from stateless to stateful.
- Bottom navigation bar.
1. Load the dependencies
We need to load the dot_env first, which we need to load initially, then only it can be used in the components.
import './pages/home_page.dart';import './pages/search_movies.dart';
Both these are our pages, which will be changed to another on tapping the bottom navigation bar.
2. Convert the class from stateless to stateful.
Initially, our class was stateless, we will convert this to the stateful. The reason for this is, we need to load this page as somewhat like conditional widgets only.
The reason for AutomaticallyKeepAliveClientMixin
it is once the page is moved from tab1 to tab2, the page automatically load and raise another API call, which is unnecessary, this case we can remove that logic.
3. Bottom navigation bar.
bottomNavigationBar: BottomNavigationBar(type: BottomNavigationBarType.fixed,items: <BottomNavigationBarItem>[],currentIndex: _selectedIndex,onTap: _onItemTapped,),
The above code handles the changes from one page to another, on tapping each button like home or Search will call the _onItemTapped
and will change the index and causes to refresh the page and our corresponding page loaded.
_onItemTapped(int index) {setState(() { this._selectedIndex = index;});}
5. Create a details page
Here what we are going to do is create a detail page that pops up on click on each movie to display the details for that particular movie like cast and some other details. So, the outline is something like this. (Explained in the project structure discussion)

To do that, we need to have some components which can be re-used anywhere in the application. Also need to pass the movie object along with the ids for the details.
In this code, the mentioned Actors
, SimilarMovies
are components that can be found in the components folder in our application. actors.dart
will display the cast working with this movie and the SimilarMovies will also have similar movies related to this genre, to avoid redundancy, we are not going to explain here, they are similar in functionality.
Code: detail.dart
6. Create a top-rated movie component
On the home page, we have a component that can be scrolled horizontally,

This is basically API provided by the tmdb, we need to only call this and display the data accordingly. Once we click on each image, need to redirect to load the details page, which is created above.
https://api.themoviedb.org/3/trending/movie/day?api_key=XXXX
To display this trending movies component, we have created a HorizontalCards
subcomponent, we just call this component and pass the necessary data viola, all set.
7. Upcoming movie component.
similar to our top-rated, we will call another API which is provided by tmdb to display the upcoming movies.

https://api.themoviedb.org/3/movie/upcoming?api_key=XXXX
That is the benefit of components here, we don't need to repeat the codes anywhere, just call the API and fetch the response and pass those values to the HorizontalCards
component.
8. Search movie

Here is an entirely new page that can be used to search for the movie and will list the movies and similar to our other workflow, on clicking on each movie, will be redirected to the detail page as well.


That’s it. We have a working App which is up and running. Please do a clap if you like this. Comments are welcome. Will be having a part 2 to add the youtube video trailers.
If this story helps you to learn anything, please feel free to buy me a coffe
Links
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK