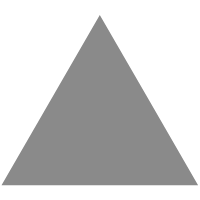
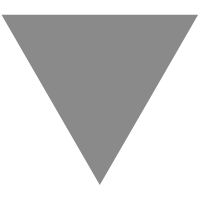
Working with DateTime and format in laravel
source link: https://justlaravel.com/datetime-format-laravel/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Working with DateTime and format in laravel
Hello everyone, Welcome back! Here am with another interesting topic, it is about DateTime and changing the format of it in our applications.
We come across many situations where we need to use some date and also format it, and sometimes also to change the language of it.
Here we display the date in the Arabic language.
Working Demo Project on Github
The simple approach we follow is, initially we add an item, get its actual date using PHP DateTime function, then convert its month to Arabic and finally store it in the database.
Adding data :
So a simple form is needed, to store an item along with created date, the form is as below,
<form action="/" method="POST"> {{ csrf_field() }} <div class="form-group row add"> <div class="col-md-8"> <input type="text" class="form-control" id="name" name="name" placeholder="Enter some name" required> <p class="error text-center alert alert-danger hidden"></p> </div> <div class="col-md-4"> <button class="btn btn-primary" type="submit" id="add"> <span class="glyphicon glyphicon-plus"></span> ADD </button> </div> </div> </form>
Storing data :
To store this data we create a table, in migrations up function,
public function up() { Schema::create('users', function (Blueprint $table) { $table->increments('id'); $table->string('name'); $table->string('email')->unique(); $table->string('password'); $table->rememberToken(); $table->timestamps(); }); }
and a model file for it,
<?php namespace App; use Illuminate\Database\Eloquent\Model; class Data extends Model { protected $table = 'data'; public $timestamps = false; }
Working with DateTime :
So now when the form is submitted. it is routed to,
Route::post ( '/', function (Request $request) { $data = new Data (); $now = new DateTime (); $standard_date = $now->format ( 'j M Y H:i:s A e' ); $standard_months = array ( "Dec", "Nov", "Oct", "Sep", "Aug", "Jul", "Jun", "May", "Apr", "Mar", "Feb", "Jan" ); $arabic_months = array ( "ديسمبر", "نوفمبر", "أكتوبر", "سبتمبر", "أغسطس", "يوليو", "يونيو", "مايو", "أبريل", "مارس", "فبراير", "يناير" ); $arabic_date = str_replace ( $standard_months, $arabic_months, $standard_date ); $data->name = $request->name; $data->date = $arabic_date; $data->save (); return Redirect::back (); } );
Working Demo Project on Github
DateTime with format options :
Here we formatted the date with 'j M Y H:i:s A e'
so it gives
j
– Day of the month without leading zeros ( 1 to 31 )M
– A short textual representation of a month, three letters ( Jan through Dec )Y
– A full numeric representation of a year, 4 digits ( Examples: 1999 or 2003 )H:i:s
– Hours, minutes and secondsA
– AM / PMe
– Time zone ( default UTC )
There are also different formatting options available for, day, week, month, year, time, timezone. The following images show the available formats.
Working with DateTime – justlaravel.com
Week :

Month :

Year :

Time :

Timezone :

Full Date/Time :

Source link: http://php.net/manual/en/function.date.php
Showing the data :
After the data is saved, we get all the data from the database,
Route::get ( '/', function () { $data = Data::all (); return view ( 'welcome' )->withData ( $data ); } );
and will show it,
<div class="table-responsive text-center"> <table class="table" id="table"> <thead> <tr> <th class="text-center">#</th> <th class="text-center">Name</th> <th class="text-center">Created Date</th> </tr> </thead> @foreach($data as $item) <tr> <td>{{$item->id}}</td> <td>{{$item->name}}</td> <td>{{$item->date}}</td> </tr> @endforeach </table> </div>

Working Demo Project on Github
Please feel free to look at some other posts on laravel here.
- Youtube Videos
- Videos
- Databases
- Alert
- Arabic
- Arabic Language
- Array
- Avg internet security
- Blueprint
- Boiler Manuals
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK