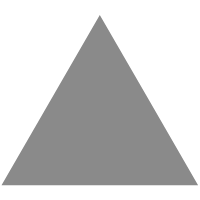
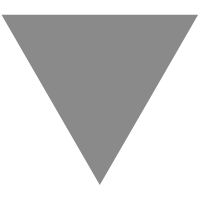
Let's Create a Simple PDF to IMG Converter in Python
source link: https://dev.to/rohankiratsata/lets-create-a-simple-pdf-to-img-converter-in-python-3glc
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
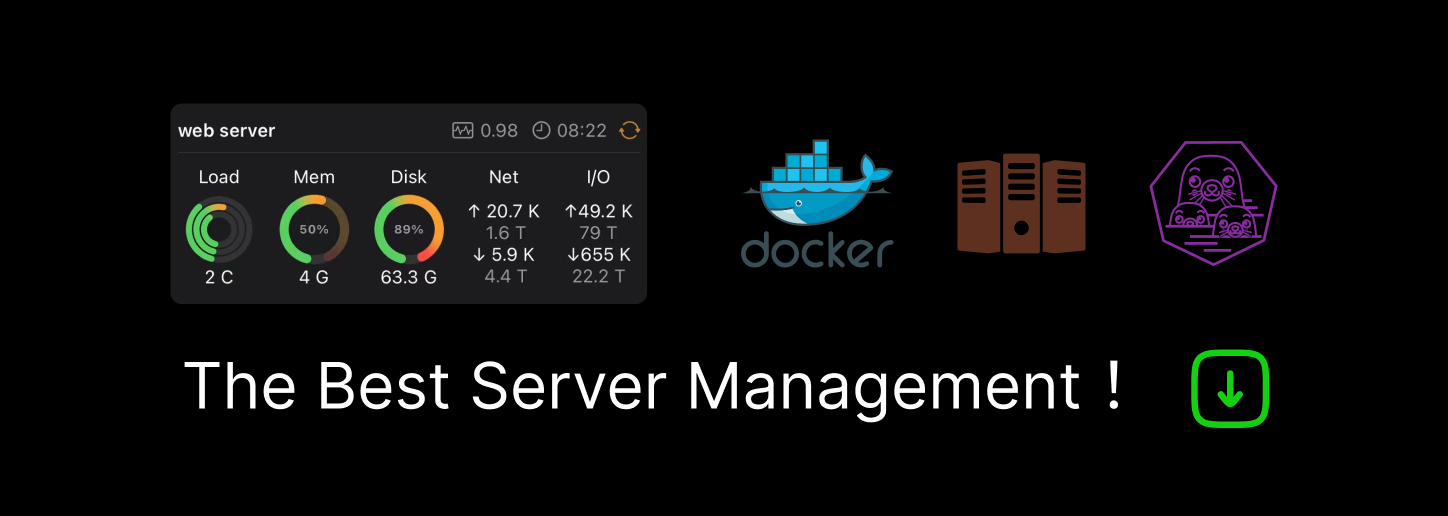
Hello Developers ! Today I'm going to show you how to make a simple GUI PDF to Image converter in python.But before we start let's see what will final GUI app will look like.
So let's get started.
Prerequisites:
Some basic knowledge of tkinter and python.
PDF2Image Package
First we need to install python library called pdf2img
.pdf2img
is command line tool to convert PDF to a PIL Image List.
To install, type pip install pdf2img
in terminal/cmd.
Poppler Package
Windows users will have to build or download poppler for Windows. Download the latest release from here.
You will then have to add the bin/
folder to PATH
or use poppler_path = r"C:\path\to\poppler-xx\bin" as an argument
in convert_from_path
.
If you don't know how to set PATH then check out here
For Mac and Linux user check out here
First we need to import the modules in python script.
# Importing Tkinter(GUI for Python)
from tkinter import *
from tkinter import messagebox
from tkinter.filedialog import askopenfilename
# Importing PDF2Image Package
from pdf2image import convert_from_path
So Now Let's create GUI for the program.
# Creating GUI Window
mainWindow = Tk()
# Title for Window
mainWindow.title("PDF2IMG CONVERTER")
# This will prevent program window to resize.
mainWindow.resizable(width=False, height=False)
# Title Heading for Program Name
titleHeading = Label(mainWindow, text="PDF2IMG CONVERTER", font=("New Times Roman", 20, "italic"))
# .grid is used to place widgets in grid, column and row. We just have to mention row and column value.
titleHeading.grid(row=0, column=0, columnspan=3, padx=10, pady=10)
# Label (Text) for Choosing File.
chooseFileLabel = Label(mainWindow, text="Choose File").grid(row=1, column=0)
# Entry widget is used to get input from user, in this case we will use it get the PDF file location.
entry = Entry(mainWindow, width=30)
entry.grid(row=1, column=1)
# Button widget for browsing the file explorer and locating file.
btnOpenFile = Button(mainWindow, text="Browse", command=lambda: source_filename())
btnOpenFile.grid(row=1, column=2)
# Button which will convert PDF to Image on click.
btnConvert = Button(mainWindow, text="CONVERT", command=pdf2img_converter, font=("New Times Roman", 12, "bold"))
btnConvert.grid(row=3, column=1, padx=5, pady=5)
# mainloop method is used when you program is ready to run.
# mainloop() is infinite loop which runs until program is not closed
mainWindow.mainloop()
So now let's create two function:
- Function which will convert pdf to image
- Function which will take the source file(i.e PDF file)
Function which will convert pdf to image:
def pdf2img_converter():
try:
# convert_from_path will get the PDF file from the entry widget we created in GUI.
imgs = convert_from_path(str(entry.get()))
for i in range(len(imgs)):
# Saves the images as JPEG File format using .save function
imgs[i].save('page'+str(i)+'.jpg', 'JPEG')
# If error or exception is found then it will show the error message box.
except Exception as e:
# Prints the exception in console.
print(e)
# Shows the result message box saying "No PDF Found"
messagebox.showinfo("Result", "NO PDF Found")
else:
# else if no error found, then it show success message box.
messagebox.showinfo("Result", "Converted to Image Successfully")
Function which will take the source PDF File.
# Function which will browse for pdf file.
def source_filename():
# configures the state of entry to normal
entry.configure(state='normal')
# clears the input in the entry
entry.delete(0, END)
# ask for file open with file types only PDF.
src = askopenfilename(
title="Choose a File",
filetypes=[('PDF Files', '.pdf')])
# Inserts the path of PDF file in the entry
entry.insert(0, src)
# again configures the state of entry to disabled.
entry.configure(state='disabled')
Now you may wonder what is the need of entry.configure
and entry.delete
?
Consider a case, when user selects the wrong pdf file. If user selects the file second time the both the paths (1st one (the wrong one) and the 2nd one) will be inserted into entry together and will throw error. So we need to delete
the previous entry and insert new one.
entry.configure(state='disabled')
is used because entry should be read only
.
Successfully Converted to Image
File Browser Opener
If PDF not Found
Full Code:
from tkinter import *
from tkinter import messagebox
from tkinter.filedialog import askopenfilename
from pdf2image import convert_from_path
def pdf2img_converter():
try:
# convert_from_path will get the PDF file from the entry widget we created in GUI.
imgs = convert_from_path(str(entry.get()))
for i in range(len(imgs)):
# Saves the images as JPEG File format using .save function
imgs[i].save('page'+str(i)+'.jpg', 'JPEG')
# If error or exception is found then it will show the error message box.
except Exception as e:
# Prints the exception in console.
print(e)
# Shows the result message box saying "No PDF Found"
messagebox.showinfo("Result", "NO PDF Found")
else:
# else if no error found, then it show success message box.
messagebox.showinfo("Result", "Converted to Image Successfully")
# Function which will browse for pdf file.
def source_filename():
# configures the state of entry to normal
entry.configure(state='normal')
# clears the input in the entry
entry.delete(0, END)
# ask for file open with file types only PDF.
src = askopenfilename(
title="Choose a File",
filetypes=[('PDF Files', '.pdf')])
# Inserts the path of PDF file in the entry
entry.insert(0, src)
# again configures the state of entry to disabled.
entry.configure(state='disabled')
# Creating GUI Window
mainWindow = Tk()
# Title for Window
mainWindow.title("PDF2IMG CONVERTER")
# This will prevent program window to resize.
mainWindow.resizable(width=False, height=False)
# Title Heading for Program Name
titleHeading = Label(mainWindow, text="PDF2IMG CONVERTER", font=("New Times Roman", 20, "italic"))
# .grid is used to place widgets in grid, column and row. We just have to mention row and column value.
titleHeading.grid(row=0, column=0, columnspan=3, padx=10, pady=10)
# Label (Text) for Choosing File.
chooseFileLabel = Label(mainWindow, text="Choose File").grid(row=1, column=0)
# Entry widget is used to get input from user, in this case we will use it get the PDF file location.
entry = Entry(mainWindow, width=30)
entry.grid(row=1, column=1)
# Button widget for browsing the file explorer and locating file.
btnOpenFile = Button(mainWindow, text="Browse", command=lambda: source_filename())
btnOpenFile.grid(row=1, column=2)
# Button which will convert PDF to Image on click.
btnConvert = Button(mainWindow, text="CONVERT", command=pdf2img_converter, font=("New Times Roman", 12, "bold"))
btnConvert.grid(row=3, column=1, padx=5, pady=5)
# mainloop method is used when you program is ready to run.
# mainloop() is infinite loop which runs until program is not closed
mainWindow.mainloop()
# This code is contributed by Rohan Kiratsata.
Source: Python Docs and My Big Brain Times.
This code is also published at my GitHub
If you found any error or spell mistakes you can do pull request on GitHub.
So, that's it for today guys, you can even add your own features like selecting output file location or making it as .exe program, in this program output file will be saved in same directory as script file. Just give it a try.. till then
KEEP CODING...KEEP HUSTLING..
Recommend
-
146
Dompdf Dompdf is an HTML to PDF converter At its heart, dompdf is (mostly) a CSS 2.1 compliant HTML layout and rendering engine written in PHP. It is a style...
-
12
object-case-converter decamelize const object = { one: 1, twoThree: 23, fourFiveSix: { four: 4, fiVe: 5, sixSeven: 67, } } const res = decamelize(object) /* { one: 1, two_three: 23, four_fiv...
-
7
Best PDF to Image Converter on Windows and MacPDF has undoubtedly become a commonly-seen file format, however there are still times we need to convert PDF documents to images. For instance, if you are a SEOer or blogger, converting PDFs into...
-
3
This article is a collaboration with UPDIVISION, an outsourcing company building complex software for companies all over the world. Read the
-
18
Save 70% off a lifetime license to PDF Converter Pro...
-
7
Support is great. Feedback is even better."We hope you are enjoying your recent purchase from our store. We would love to hear your feedback about your experience with the product. Your feedback is essential in helping us improve our...
-
11
Receipt Bot is easy to use. Simply upload your PDF statements, and we'll do the rest. In just a few minutes,
-
57
This PDF converter allows you to convert PDF to images and others available formats 100% free.
-
6
A-PDF All to MP3 Converter 2.0.0 - DEP Bypass via HeapCreate + HeapAlloc...
-
7
Image To PDF Converter using React ...
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK