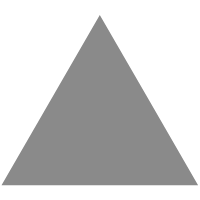
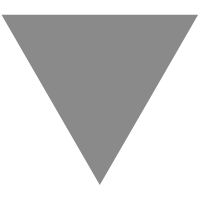
Random numbers of an array in another array with different rules of occurrence i...
source link: https://www.codesd.com/item/random-numbers-of-an-array-in-another-array-with-different-rules-of-occurrence-in-java.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Random numbers of an array in another array with different rules of occurrence in java
I need help for an exercice in school.
I need to create an array with 6 random integers from the following array : montab[] = {1,2,3,4,5,6,7,8,9,10,25,50,75,100}
and with the following rules :
- numbers
25,50,75,100
can only occur once each in the array - numbers
1
to10
can only occur twice each in the array
I tried the first rule for now but in rare cases I still get the number more than once.
Here is my code :
public class Exo7bis {
public static void main (String[] args){
Random random = new Random();
int montab[] = {1,2,3,4,5,6,7,8,9,10,25,50,75,100};
int[] ar1 = new int[6];
int j = 0, compteur25 = 0, compteur50 = 0, compteur75 = 0, compteur100 = 0;
for (int i = 0; i < ar1.length; i++) {
ar1[i] = (montab[new Random().nextInt(montab.length)]);
if (ar1[i] == 25) {
compteur25++;
if (compteur25 > 1) {
while (ar1[i] == 25)
ar1[i] = (montab[new Random().nextInt(montab.length)]);
}
}
if (ar1[i] == 50) {
compteur50++;
if (compteur50 > 1) {
while (ar1[i] == 50)
ar1[i] = (montab[new Random().nextInt(montab.length)]);
}
}
if (ar1[i] == 75) {
compteur75++;
if (compteur75 > 1) {
while (ar1[i] == 75)
ar1[i] = (montab[new Random().nextInt(montab.length)]);
}
}
if (ar1[i] == 100) {
compteur100++;
if (compteur100 > 1) {
while (ar1[i] == 100)
ar1[i] = (montab[new Random().nextInt(montab.length)]);
}
}
}
for (int i = 0; i < ar1.length; i++) {
System.out.print(ar1[i] +" ⎢ " + "\t");
}
}
}
I know that my tests are not totally right, I identified the problem but I can't find the proper solution.
If someone could help me or advise me, that would be cool.
Thanks in advance!
Jeremy
There are many ways to achieve this but since you are just using arrays I will advise you making a function that counts how many time 1-10
is repeated. For the first the condition you could just replace the element with 0
so it will not repeat next time. I think it's easy to explain in code so have a look at what you could change in your code:
public static void main(String[] args) {
Random random = new Random();
int montab[] = { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 25, 50, 75, 100 };
int[] ar1 = new int[6];
int j = 0, count = 0;
while (j < ar1.length) {
count = 0;
int index = random.nextInt(montab.length);
int num = montab[index];
if (num >= 25) { //adds any number greater or equal to 25
ar1[j] = num;
j++;
montab[index] = 0; // replace the origianl array with 0.
} else if (num != 0) {
if(!isRepeated(ar1,num)){ //checks if the array has more than two of the number.
ar1[j] = num;
j++;
}
}
}
for (int i = 0; i < ar1.length; i++) {
System.out.print(ar1[i] + " ⎢ " + "\t");
}
}
public static boolean isRepeated(int[] arr, int num) { //method that verifies if the array has a number repeated twice or not.
int count = 0;
for (int i = 0; i < arr.length; i++) {
if (arr[i] == num)
count++;
}
return count==2 ? true : false;
}
I haven't tested it but I'm pretty sure it will work!!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK