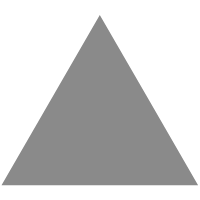
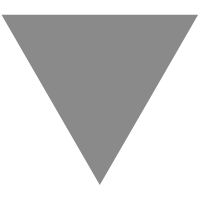
直接拿来用!盘点一些拯救头发的 JS 单行代码,网友:摸鱼必备啊
source link: https://segmentfault.com/a/1190000040782397
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
直接拿来用!盘点一些拯救头发的 JS 单行代码,网友:摸鱼必备啊
作者:Ahmad
译者:前端小智
来源:ishadee
有梦想,有干货,微信搜索 【大迁世界】 关注这个在凌晨还在刷碗的刷碗智。
本文 GitHub https://github.com/qq449245884/xiaozhi 已收录,有一线大厂面试完整考点、资料以及我的系列文章。
废话不多说,直接上。
检查一个元素是否被聚焦
const hasFocus = (ele) => ele === document.activeElement
获取一个元素的所有兄弟元素
const siblings = (ele) => .slice.call(ele.parentNode.children).filter((child) => child !== ele);
获取选中文本
const getSelectedText = () => window.getSelection().toString();
回到上一页
history.back(); // Or history.go(-1);
清除所有 cookies
const cookies = document.cookie.split(';').map((item) => item.split('=')).reduce((acc, [k, v]) => (acc[k.trim().replace('"', '')] = v) && acc, {});
将 cookie 转换为对象
const cookies = document.cookie.split(';').map((item) => item.split('=')).reduce((acc, [k, v]) => (acc[k.trim().replace('"', '')] = v) && acc, {});
比较两个数组
// `a` 和 `b` 都是数组 const isEqual = (a, b) => JSON.stringify(a) === JSON.stringify(b); // 或者 const isEqual = (a, b) => a.length === b.length && a.every((v, i) => v === b[i]); // 事例 isEqual([1, 2, 3], [1, 2, 3]); // true isEqual([1, 2, 3], [1, '2', 3]); // false
将对象数组转换为单个对象
const toObject = (arr, key) => arr.reduce((a, b) => ({ ...a, [b[key]]: b }), {}); // Or const toObject = (arr, key) => Object.fromEntries(arr.map((it) => [it[key], it])); // 事例 toObject([ { id: '1', name: 'Alpha', gender: 'Male' }, { id: '2', name: 'Bravo', gender: 'Male' }, { id: '3', name: 'Charlie', gender: 'Female' }], 'id'); /* { '1': { id: '1', name: 'Alpha', gender: 'Male' }, '2': { id: '2', name: 'Bravo', gender: 'Male' }, '3': { id: '3', name: 'Charlie', gender: 'Female' } } */
根据对象数组的属性进行计数
const countBy = (arr, prop) => arr.reduce((prev, curr) => ((prev[curr[prop]] = ++prev[curr[prop]] || 1), prev), {}); // 事例 countBy([ { branch: 'audi', model: 'q8', year: '2019' }, { branch: 'audi', model: 'rs7', year: '2020' }, { branch: 'ford', model: 'mustang', year: '2019' }, { branch: 'ford', model: 'explorer', year: '2020' }, { branch: 'bmw', model: 'x7', year: '2020' }, ], 'branch'); // { 'audi': 2, 'ford': 2, 'bmw': 1 }
检查数组是否为空
const isNotEmpty = (arr) => Array.isArray(arr) && Object.keys(arr).length > 0; // 事例 isNotEmpty([]); // false isNotEmpty([1, 2, 3]); // true
检查多个对象是否相等
const isEqual = (...objects) => objects.every((obj) => JSON.stringify(obj) === JSON.stringify(objects[0])); // 事例 console.log(isEqual({ foo: 'bar' }, { foo: 'bar' })); // true console.log(isEqual({ foo: 'bar' }, { bar: 'foo' })); // false
从对象数组中提取指定属性的值
const pluck = (objs, property) => objs.map((obj) => obj[property]); // Example const aa = pluck([ { name: '小智', age: 20 }, { name: '大志', age: 25 }, { name: '王大志', age: 30 }, ], 'name'); // [ '小智', '大志', '王大志' ]
反转对象的键和值
const invert = (obj) => Object.keys(obj).reduce((res, k) => Object.assign(res, { [obj[k]]: k }), {}); // 或 const invert = (obj) => Object.fromEntries(Object.entries(obj).map(([k, v]) => [v, k])); // 事例 invert({ a: '1', b: '2', c: '3' }); // { 1: 'a', 2: 'b', 3: 'c' }
从对象中删除所有 null 和 undefined 的属性
const removeNullUndefined = (obj) => Object.entries(obj) .reduce((a, [k, v]) => (v == null ? a : ((a[k] = v), a)), {}); // 或 const removeNullUndefined = (obj) => Object.entries(obj) .filter(([_, v]) => v != null) .reduce((acc, [k, v]) => ({ ...acc, [k]: v }), {}); // 或 const removeNullUndefined = (obj) => Object.fromEntries(Object.entries(obj).filter(([_, v]) => v != null)); // 例子 removeNullUndefined({ foo: null, bar: undefined, fuzz: 42} ); // { fuzz: 42 }
根据对象的属性对其进行排序
Object.keys(obj) .sort() .reduce((p, c) => ((p[c] = obj[c]), p), {}); // 事例 const colors = { white: '#ffffff', black: '#000000', red: '#ff0000', green: '#008000', blue: '#0000ff', }; sort(colors); /* { black: '#000000', blue: '#0000ff', green: '#008000', red: '#ff0000', white: '#ffffff', } */
检查一个对象是否是Promise
const isPromise = (obj) => !!obj && (typeof obj === 'object' || typeof obj === 'function') && typeof obj.then === 'function';
检查一个对象是否是一个数组
const isArray = (obj) => Array.isArray(obj);
检查路径是否是相对路径
const isRelative = (path) => !/^([a-z]+:)?[\\/]/i.test(path); // 例子 isRelative('/foo/bar/baz'); // false isRelative('C:\\foo\\bar\\baz'); // false isRelative('foo/bar/baz.txt'); // true isRelative('foo.md'); // true
将字符串的第一个字符改为小写
const lowercaseFirst = (str) => `${str.charAt(0).toLowerCase()}${str.slice(1)}`; // 例子 lowercaseFirst('Hello World'); // 'hello World'
重复一个字符串
const repeat = (str, numberOfTimes) => str.repeat(numberOfTimes); // 例子 repeat('ab', 3) // ababab
Dates
给一个小时添加“am/pm”后缀
// `h` is an hour number between 0 and 23 const suffixAmPm = (h) => `${h % 12 === 0 ? 12 : h % 12}${h < 12 ? 'am' : 'pm'}`; // 例子 suffixAmPm(0); // '12am' suffixAmPm(5); // '5am' suffixAmPm(12); // '12pm' suffixAmPm(15); // '3pm' suffixAmPm(23); // '11pm'
计算两个日期之间的不同天数
const diffDays = (date, otherDate) => Math.ceil(Math.abs(date - otherDate) / (1000 * 60 * 60 * 24)); // 例子 diffDays(new Date('2014-12-19'), new Date('2020-01-01')); // 1839
检查日期是否有效
const isDateValid = (...val) => !Number.isNaN(new Date(...val).valueOf()); isDateValid("December 17, 1995 03:24:00"); // true
检查代码是否在Node.js中运行
const isNode = typeof process !== 'undefined' && process.versions != null && process.versions.node != null;
检查代码是否在浏览器中运行
const isBrowser = typeof window === 'object' && typeof document === 'object';
将URL参数转换为对象
const getUrlParams = (query) =>Array.from(new URLSearchParams(query)).reduce((p, [k, v]) => Object.assign({}, p, { [k]: p[k] ? (Array.isArray(p[k]) ? p[k] : [p[k]]).concat(v) : v }),{}); // 例子 getUrlParams(location.search); // Get the parameters of the current URL getUrlParams('foo=Foo&bar=Bar'); // { foo: "Foo", bar: "Bar" } // Duplicate key getUrlParams('foo=Foo&foo=Fuzz&bar=Bar'); // { foo: ["Foo", "Fuzz"], bar: "Bar" }
黑暗检测模式
const isDarkMode = window.matchMedia && window.matchMedia('(prefers-color-scheme: dark)').matches;
拷贝到剪切板
const copyToClipboard = (text) => navigator.clipboard.writeText(text); // 例子 copyToClipboard("Hello World");
将RGB转换为十六进制
const rgbToHex = (r, g, b) => "#" + ((1 << 24) + (r << 16) + (g << 8) + b).toString(16).slice(1); // 例子 rgbToHex(0, 51, 255); // #0033ff
生成一个随机的十六进制颜色
const randomColor = () => `#${Math.random().toString(16).slice(2, 8).padEnd(6, '0')}`; // 或者 const randomColor = () => `#${(~~(Math.random() * (1 << 24))).toString(16)}`;
生成随机IP地址
const randomIp = () => Array(4).fill(0) .map((_, i) => Math.floor(Math.random() * 255) + (i === 0 ? 1 : 0)) .join('.'); // 例子 randomIp(); // 175.89.174.131
使用 Node crypto 模块生成随机字符串
const randomStr = () => require('crypto').randomBytes(32).toString('hex');
~~完,我是刷碗智,准备去打个点滴,我们下期见!
代码部署后可能存在的BUG没法实时知道,事后为了解决这些BUG,花了大量的时间进行log 调试,这边顺便给大家推荐一个好用的BUG监控工具 Fundebug。
https://javascript.plainengli...
https://javascript.plainengli...
有梦想,有干货,微信搜索 【大迁世界】 关注这个在凌晨还在刷碗的刷碗智。
本文 GitHub https://github.com/qq44924588... 已收录,有一线大厂面试完整考点、资料以及我的系列文章。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK