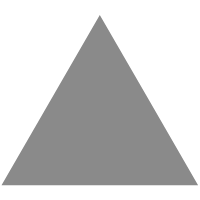
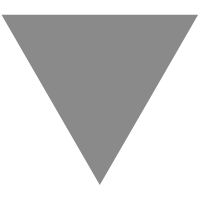
JavaScript 中优雅处理对象的 6 个方法
source link: https://www.techug.com/post/six-methods-of-handling-objects-gracefully-in-javascript.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
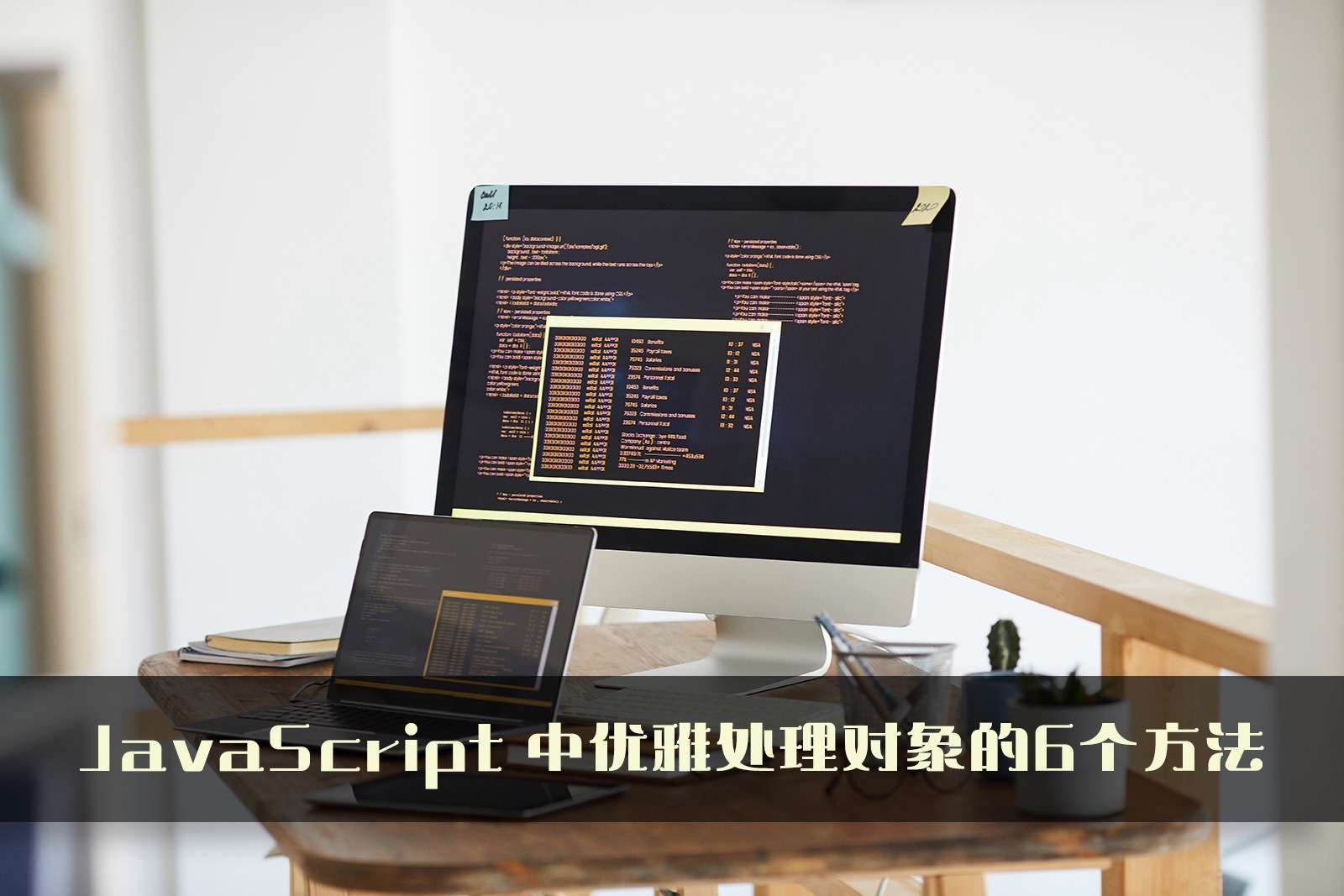
和其他编程语言一样,JavaScript 有自己的数据类型,如数字、字符串、数组、对象等。而对象在 JavaScript 中是一种非常重要的数据类型,它们有很多有用的方法,在平常项目开发中可以使用这些方法容易地处理对象。本文介绍 6 个在项目中用得上的方法,趁此机会加深其使用方法。
1、Object.freeze()
Object.freeze()
方法可以防止对象中的数据被修改,即冻结一个对象,这样不能向这个对象 添加、更新或删除属性。
const author = { name: "Quintion", city: "Shenzhen", age: 18, validation: true, }; Object.freeze(author); author.name = "QuintionTang"; author.province = "Guangdong"; delete author.age; console.log(author); // { name: 'Quintion', city: 'Shenzhen', age: 18, validation: true }
如上面的代码,更新属性name
、新增属性province
、删除属性age
,最终对象都没有任何改变。
2、Object.seal()
Object.seal()
方法有点类似于 Object.freeze()
。阻止向对象添加新的属性和删除属性,但允许更改和更新现有属性。
const author = { name: "Quintion", city: "Shenzhen", age: 18, validation: true, }; Object.seal(author); author.name = "QuintionTang"; author.province = "Guangdong"; delete author.age; console.log(author); // { name: 'QuintionTang', city: 'Shenzhen', age: 18, validation: true }
从上面代码可以看到,新增属性和删除属性都无效,只有更新属性name
生效了。
3、Object.keys()
Object.keys()
方法会返回一个数组,该数组包含参数对象的所有键的名称,数组中属性名的排列顺序和正常循环遍历该对象时返回的顺序一致 。
看看下面的代码:
const author = { name: "Quintion", city: "Shenzhen", age: 18, validation: true, }; console.log(Object.keys(author)); // [ 'name', 'city', 'age', 'validation' ]
可以看到上面的代码中打印的结果是一个包含键作为输出的数组。输出的结果可以使用数组的方法进行处理或者迭代。
console.log(Object.keys(author).length); // 4
4、Object.values()
Object.values()
和 Object.keys()
类似,不过Object.values()
是获取对象内素有属性的值,返回值组成的数组。
const author = { name: "Quintion", city: "Shenzhen", age: 18, validation: true, }; console.log(Object.values(author)); // [ 'Quintion', 'Shenzhen', 18, true ]
5、Object.create()
Object.create()
基于现有对象的原型__proto__
创建一个新对象,先来看下面代码:
const author = { firstName: "Quintion", lastName: "Tang", fullName() { return `${this.firstName} ${this.lastName}`; }, }; const newAuthor = Object.create(author); console.log(newAuthor); // {} newAuthor.firstName = "Ronb"; newAuthor.lastName = "Joy"; console.log(newAuthor.fullName()); // Ronb Joy
在上面的代码中,使用object. create()
创建一个具有author
对象原型的新对象newAuthor
。这样在新对象newAuthor
中可以像改变author
对象所拥有的属性值一样改变相应的属性值,这个看起来是不有点像继承,没错, 使用 Object.create
可以实现类式继承。
6、Object.entries()
Object.entries() 允许获取对象的键和值,返回一个多维数组,其中每一维包含每个键和值,如 [键 , 值]
const author = { firstName: "Quintion", lastName: "Tang", fullName() { return `${this.firstName} ${this.lastName}`; }, }; console.log(Object.entries(author));
输出的结果如下:
[ [ 'firstName', 'Quintion' ], [ 'lastName', 'Tang' ], [ 'fullName', [Function: fullName] ] ]
本文对对象常见的 6 个方法做了简单的介绍,并提供了相应的示例代码,在实际编码处理对象的过程中,使用上面的方法可以让代码变得更加优雅。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK