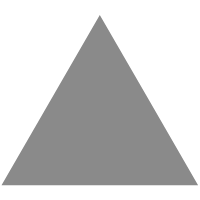
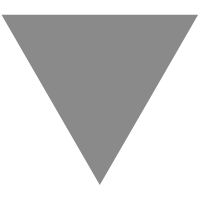
【JavaScript实用技巧(一)】循环遍历与跳出循环遍历
source link: https://segmentfault.com/a/1190000040856680
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
【JavaScript实用技巧(一)】循环遍历与跳出循环遍历
博客说明
文章所涉及的资料来自互联网整理和个人总结,意在于个人学习和经验汇总,如有什么地方侵权,请联系本人删除,谢谢!
一想到想到循环,顺其自然就想到了for,while就在这里先不讲(因为本身它就有循环退出的条件),但其实Js的循环不止这个for,这里来详细讲讲Js的几种类for循环。
一想到想到循环的跳出,立马就会想到三个关键:break
、return
、continue
,在业务中也会需要在遍历的时候退出循环。
for类循环遍历数组
1、for循环
const arr = [1, 2, 3, 4, 5, 6] for (let i = 0; i < arr.length; i++) { console.log(i) }
这是最简单的一种,也是使用的频率最高的一种,性能相对其他的要好,所以看见别人源码底层用这个千万别xxx,三思而行!
不过这里有一个细节可以优化,将长度作为临时变量存起来,不必每次计算,在数据量大的时候提升较为明显。
const arr = [1, 2, 3, 4, 5, 6] for (let i = 0, len = arr.length; i < len; i++) { console.log(i) }
2、for...in循环
const arr = [1, 2, 3, 4, 5, 6] for (let i in arr) { console.log(i) }
在数组上应用for...in循环,不仅仅会包含所有数值索引,还会包含所有可枚举属性。所以一般用于对象的遍历上。且这个方式的循环遍历的效率最低
3、forEach循环
const arr = [1, 2, 3, 4, 5, 6] arr.forEach((item, index) => { console.log(item) })
forEach是数组自带的,使用的频率比较高,但是性能比for要低一些
由于forEach是数组自带的,所以在其他类似数组类型下需要做个变化,它的性能比forEach弱一些
const arr = [1, 2, 3, 4, 5, 6] Array.prototype.forEach.call(arr, (item) => { console.log(item) })
4、map遍历
const arr = [1, 2, 3, 4, 5, 6] arr.map((item) => { console.log(item) })
map的方式用的原来越多,使用十分方便和优雅,但是效率比较低(相对于forEach)
5、for...of遍历
const arr = [1, 2, 3, 4, 5, 6] for (let i of arr) { console.log(i) }
for...of是es6的新语法,性能比for...in好一些,但是比不上普通for循环
上面提到跳出循环时想到的三个关键词:break
、return
、continue
,for和for...in能够响应这三个关键词,但是forEach不行。先讲一下这个三个关键词吧
for循环跳出循环
- break:break语句会使运行的程序立刻退出包含在最内层的循环或者退出一个switch语句。
- continue:continue语句和break语句相似。所不同的是,它不是退出一个循环,而是开始循环的一次新迭代。continue语句只能用在while语句、do/while语句、for语句、或者for/in语句的循环体内, 在其他地方使用都会引起错误。
- return:return语句就是用于指定函数返回的值。return语句只能出现在函数体内,出现在代码中的其他任何地方造成语法错误。
const arr = [1, 2, 3, 4, 5, 6] for (let i = 0; i < arr.length; i++) { console.log(i) if (i === 2) { return; // breack; } }
forEach跳出循环
在forEach中不响应这个跳出循环的语句,所以它不行,但一定要让它行,行不行?行!
有一个骚操作,直接来个抛错,我不终止,我抛错!
try { const arr = [1, 2, 3, 4, 5, 6] // 执行到第4次,结束循环 arr.forEach(function(item,index){ if (item === 4) { throw new Error("EndIterative"); } console.log(item);// 1,2,3 }); } catch(e) { if(e.message!="EndIterative") throw e; }; // 下面的代码不影响继续执行 console.log(10);
但是这种方式不推荐,咱没必要,直接用for循环就好,速度又快
感谢
万能的网络
公众号-归子莫,小程序-小归博客
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK