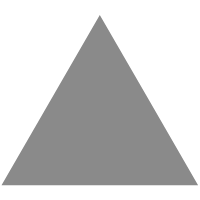
4
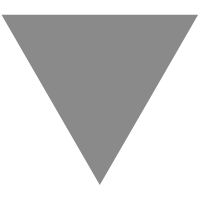
LeetCode-039-组合总和
source link: https://segmentfault.com/a/1190000040826497
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
LeetCode-039-组合总和
解法一:穷举法题目描述:给定一个无重复元素的数组 candidates 和一个目标数 target ,找出 candidates 中所有可以使数字和为 target 的组合。
candidates 中的数字可以无限制重复被选取。
- 所有数字(包括 target)都是正整数。
- 解集不能包含重复的组合。
示例说明请见LeetCode官网。
来源:力扣(LeetCode)
链接:https://leetcode-cn.com/probl...
著作权归领扣网络所有。商业转载请联系官方授权,非商业转载请注明出处。
类似构造一棵多叉树,最大深度为candidates数组的长度,然后获取所有可能的路径,最大路径是由根节点到叶子节点,判断所有的路径之和是否等于target,如果相等,则加到结果集中,最后需要判重,把重复的组合去掉,最后返回。
import java.util.*; public class LeetCode_039 { /** * 穷举法 * * @param candidates * @param target * @return */ public static List<List<Integer>> combinationSum(int[] candidates, int target) { // 结果集 List<List<Integer>> result = new ArrayList<>(); // 所有可能的组合情况 Queue<List<Integer>> allPossibleCombinations = new LinkedList<>(); // 初始化所有的情况 for (int candidate : candidates) { List<Integer> onePossibleCombination = new ArrayList<>(); onePossibleCombination.add(candidate); allPossibleCombinations.add(onePossibleCombination); } while (!allPossibleCombinations.isEmpty()) { List<Integer> temp = allPossibleCombinations.poll(); int sum = 0; for (Integer num : temp) { sum += num; } if (sum == target) { result.add(temp); } else if (sum < target) { for (int candidate : candidates) { // List复制方法 List<Integer> toAdd = new ArrayList<>(Arrays.asList(new Integer[temp.size()])); Collections.copy(toAdd, temp); toAdd.add(candidate); allPossibleCombinations.add(toAdd); } } } // 去重后的结果 List<List<Integer>> result1 = new ArrayList<>(); for (int i = 0; i < result.size(); i++) { boolean isRepeated = false; List<Integer> one = result.get(i); Collections.sort(one); for (int j = i + 1; j < result.size(); j++) { List<Integer> two = result.get(j); Collections.sort(two); if (one.size() != two.size()) { continue; } boolean equals = true; for (int x = 0; x < one.size(); x++) { if (!one.get(x).equals(two.get(x))) { equals = false; continue; } } if (equals) { isRepeated = true; } } if (!isRepeated) { result1.add(one); } } return result1; } public static void main(String[] args) { int[] candidates = new int[]{8, 10, 6, 3, 4, 12, 11, 5, 9}; for (List<Integer> integers : combinationSum(candidates, 28)) { for (Integer integer : integers) { System.out.print(integer + " "); } System.out.println(); } } }
【每日寄语】 不要急着让生活给予所有的答案,有时我们需要耐心的等待。相信过程,坦然前行,不负生活,生活也必不负你。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK