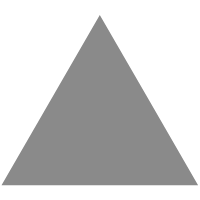
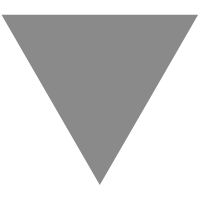
Programming in Swift: Fundamentals
source link: https://www.raywenderlich.com/28092971-programming-in-swift-fundamentals
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Core Concepts
Introduction
4:34 FreeLet’s review what you’ll be learning in this section, and why it’s important.
Swift Playgrounds & Comments
8:34 FreeLearn how to create your first Swift playground, what all the various bits are in playgrounds, and how comments work.
Booleans & Comparison Operators
10:12 FreeLearn how to use a Swift type called Booleans, which represent true or false values.
Challenge: Booleans
4:04 FreePractice using booleans on your own, through a series of hands-on challenges.
Logical Operators
15:37 FreeLearn how to use various logical and comparison operators in Swift to create Booleans.
Challenge: Logical Operators
3:42 FreePractice using logical operators on your own, through a series of hands-on challenges.
Optionals
11:41 FreeLearn about one of the most important aspects of Swift development - optionals - and how to unwrap, force unwrap, and bind optionals.
Challenge: Optionals
3:20 FreePractice using optionals on your own, through a set of hands-on challenges.
Conclusion
1:50 FreeLet’s review where you are with your Swift core concepts, and discuss what’s next.
Beginning Collections
Introduction
1:04Let’s review what you’ll be learning in this section, and why it’s important.
Tuples
6:24Learn to group related data together into a single unit, through the power of a Swift type called Tuples.
Challenge: Tuples
5:05Practice using tuples on your own, through a series of hands-on challenges.
Arrays
5:13Learn how to use arrays in Swift to store an ordered list of values.
Operating on Arrays
10:06See how to manipulate arrays with their built-in methods.
Challenge: Arrays
5:15Practice using arrays on your own, through a hands-on challenge.
Conclusion
0:59Let’s review where you are with your Swift core concepts, and discuss what’s next.
Control Flow
Introduction
1:15Let’s review what you’ll be learning in this section, and why it’s important.
While Loops
6:31Learn how to make Swift repeat your code multiple times with while loops, repeat while loops, and break statements.
Practice using while loops on your own, through a hands-on challenges.
For Loops
10:04Learn how to use for loops in Swift, along with ranges, continue, and labeled statements.
Challenge: For Loops
4:29Practice using for loops on your own, through a hands-on challenge.
Learn how to use your existing knowledge of for loops to iterate over collections.
Practice iterating over collections using loops on your own, through a hands-on challenges.
Learn how to nest one loop inside another, and even terminate a loop early if and when you need to.
Practice working with nested loops and the break and continue statements on your own, through a hands-on challenge.
Conclusion
1:18Let’s review where you are with your Swift core concepts, and discuss what’s next.
More Collections
Introduction
1:09Let’s review what you’ll be learning in this section, and why it’s important.
Learn how to create and populate Dictionaries, a useful collection in Swift.
See how to access the contents of dictionaries and manipulate dictionaries.
Practice using dictionaries on your own, through a hands-on challenge.
Working with Sets
8:02Learn how to create sets, how to populate them with data and retrieve that data, and how to compare sets to each other with their built-in methods.
Challenge: Sets
4:39Practice using sets on your own, through a hands-on challenge.
Conclusion
1:16Let’s review what you learned in this section, and discuss what’s next.
Functions & Named Types
Introduction
3:24Let’s review what you’ll be learning in this section, and why it’s important.
Learn how to write your own functions in Swift, and see for yourself how Swift makes them easy to use.
Functions & Return
9:16See how the “return” keyword is used in Swift, and when it’s required and when it’s optional.
Challenge: Functions
3:34Practice writing functions on your own, through a hands-on challenge.
Structures
10:22Learn how structures work, and all about their properties and methods.
Practice writing structures on your own, through a hands-on challenge.
Classes
9:32Learn about the basics of Swift classes, and study how they are similar to and different from structures.
Challenge: Classes
2:42Practice writing classes on your own, through a hands-on challenge.
Conclusion
1:46Let’s review what you learned in this section, and discuss what’s next for you in this learning path.
Who is this for?
Beginners! If you’ve never created a playground before, or if you aren’t sure what while loops or break statements are, this is a great course to get you started. With easy-to-understand lessons and hands-on practice, soon you’ll be writing your own methods and implementing structures and classes with ease.
You’ll start at the very beginning, creating your first playground and learning about comments, tuples, booleans, and operators. Then, you’ll learn to control the flow of your code. For loops, switch statements, enums, oh my! You’ll build on the basics by implementing functionals and optionals through fun, hands-on challenges.
This course isn’t suited for intermediate or advanced developers. If that’s you, check out our intermediate or advanced video courses for more ways to level-up your developer skills!
Covered concepts
- Playgrounds
- Comments
- Tuples
- Booleans
- Operators
- For loops
- While loops
- Switch statements
- Enumerations
- Functionals
- Optionals
- Collections
- Arrays
- Dictionaries
- Structures
- Properties
- Methods
- Classes/Subclasses
- Protocols
- Closures
- Initializers
- Memory management
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK