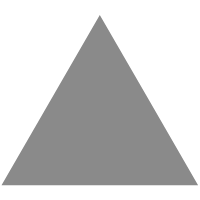
0
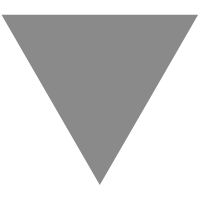
PHP cheatsheet
source link: https://devhints.io/wip/php
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
Hello world
hello.php
<?php
function greetMe($name): string
{
return "Hello, " . $name . "!";
}
$message = greetMe($name);
echo $message;
All PHP files start with <?php
.
See: PHP tags
Objects
<?php
$fruitsArray = array(
"apple" => 20,
"banana" => 30
);
echo $fruitsArray['banana'];
Or cast as object
<?php
$fruitsObject = (object) $fruits;
echo $fruitsObject->banana;
Inspecting objects
Classes
class Person
{
public $name = '';
}
$person = new Person();
$person->name = 'bob';
echo $person->name;
Getters and setters
class Person
{
private $name = '';
public function getName(): string
{
return $this->name;
}
public function setName(string $name)
{
$this->name = $name;
return $this;
}
}
$person = new Person();
$person->setName('bob');
echo $person->getName();
isset vs empty
$options = [
'key' => 'value',
'blank' => '',
'nothing' => null,
];
var_dump(isset($options['key']), empty($options['key'])); // true, false
var_dump(isset($options['blank']), empty($options['blank'])); // true, true
var_dump(isset($options['nothing']), empty($options['nothing'])); // false, true
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK