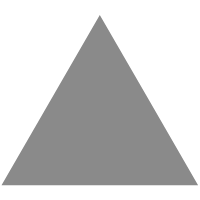
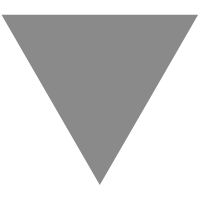
Passing an assembly value to an application in C #
source link: https://www.codesd.com/item/passing-an-assembly-value-to-an-application-in-c.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
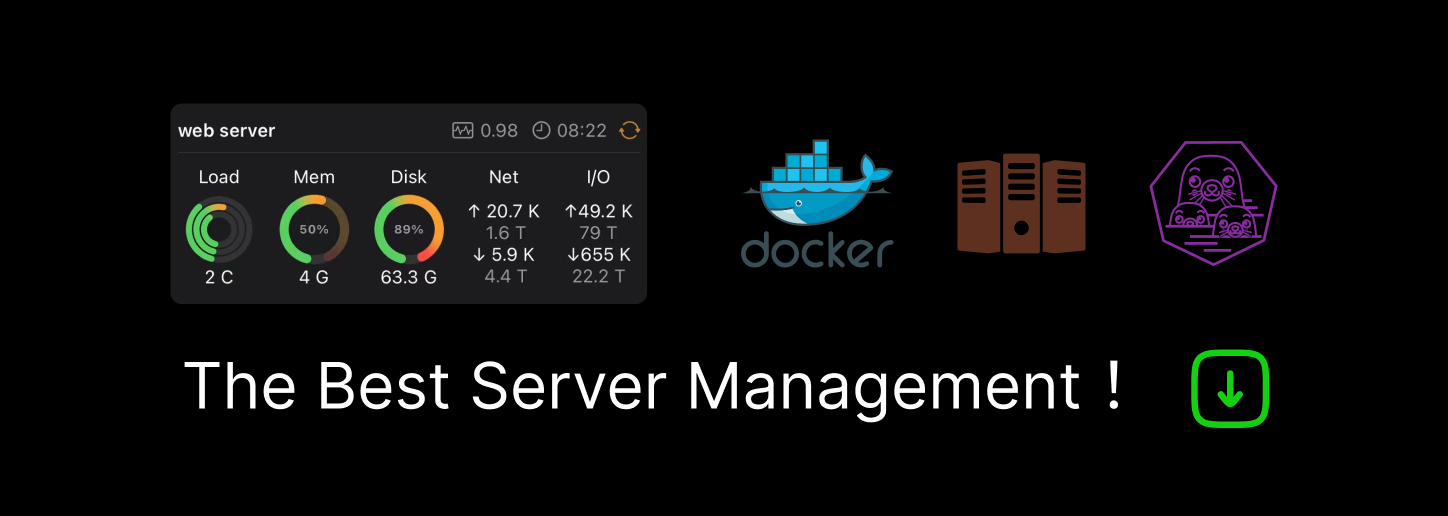
Passing an assembly value to an application in C #
If I have an assembly which contains a function that manipulates a string and then returns that string, and I then have a separate C# application which calls the function from the assembly...
How can I pass the manipulated string from the assembly into the application?
For example, If I have this assembly...
using System;
namespace test
{
public class Class1
{
string inputString = "hello";
string outputString;
public static string Convert(ref inputString, ref outputString)
{
outputString = inputString.ToUpper();
return outputString;
}
}
}
And I have this application which calls the Convert function within the assembly...
using System;
using test;
public class Class2
{
public static void Main()
{
Class1.Convert();
}
}
How can I get the returned outputString into the Class2 application? I can't reference it in the Main() function so how can I pass it in?
It looks like you want to pass in a valid and then return another value? Then get rid of the of properties on your Class1, they are not necessary. Just make the input string the parameter of the Convert function, and then return the output.
using System;
namespace test
{
public class Class1
{
public static string Convert(string inputString)
{
string outputString = inputString.ToUpper();
return outputString;
}
}
}
using System;
using test;
public class Class2
{
public static void Main()
{
string thisIsMyReturnedString = Class1.Convert("whatever the input value should be");
}
}
Recommend
-
216
Java: What to Know About Passing by Value Of course, in Java,...
-
68
-
62
Remember when Huawei tried passing a photo from a $4,500 camera off as one from the then-new P9? Samsung Brazil's just done the same thing, but with a lot... by Richard Gao in News, Samsung
-
64
T here are so many misconceptions and debate on the internet about how JavaScript passes values to functions. So...
-
10
Blast Off with Blazor: Prerender a Blazor Web Assembly application In this post, we speed up initial load time by prerendering our Bla...
-
18
We often come across cases in the Rails application where we need to bulk-insert records. Rails 6 introduced insert_all and upsert_all methods to solve bulk import issues.
-
7
.NET6 Application No Longer Generates A Separate Assembly For Views By The Razor Compiler[.NET6 Feature] In this article, we will understand a .NET6 feature that is in a .NET6 applic...
-
11
To talk about passing/copying values in JavaScript we need to talk about data types. JavaScript data types can be grouped into two categories: Simple data types Complex data types Simple data...
-
3
Passing Any Arbitrary Value From A Power BI Report To A Dynamic M Parameter Dynamic M parameter...
-
8
Sivakami P Subramanian June 9, 2023 1 min...
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK