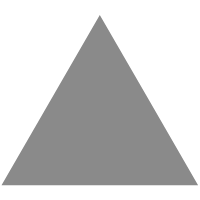
8
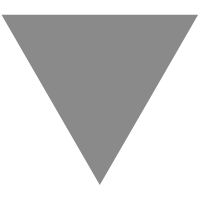
LeetCode-374-猜数字大小
source link: https://segmentfault.com/a/1190000040748785
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
LeetCode-374-猜数字大小
发布于 9 月 28 日
猜数字大小
解法一:二分查找法题目描述:猜数字游戏的规则如下:
- 每轮游戏,我都会从 1 到 n 随机选择一个数字。 请你猜选出的是哪个数字。
- 如果你猜错了,我会告诉你,你猜测的数字比我选出的数字是大了还是小了。
你可以通过调用一个预先定义好的接口 int guess(int num) 来获取猜测结果,返回值一共有 3 种可能的情况(-1,1 或 0):
- -1:我选出的数字比你猜的数字小 pick < num
- 1:我选出的数字比你猜的数字大 pick > num
- 0:我选出的数字和你猜的数字一样。恭喜!你猜对了!pick == num
返回我选出的数字。
示例说明请见LeetCode官网。
来源:力扣(LeetCode)
链接:https://leetcode-cn.com/probl...
著作权归领扣网络所有。商业转载请联系官方授权,非商业转载请注明出处。
典型的二分查找问题,首先low初始值为1,high初始值为num,二分查找过程如下:
- 循环的前提是low不大于high;
- 然后mid的值为
low + (high - low) / 2
,然后调用guess(num)
方法进行判断;- 如果返回-1,则high的值置为
mid - 1
,然后进行下一轮处理;- 如果返回1,则low的值置为
mid + 1
,然后进行下一轮处理;- 如果返回0,则返回mid值,该值即为选出的数字。
public class LeetCode_374 extends GuessGame { /** * 二分查找 * * @param num * @return */ public static int guessNumber(int num) { int low = 1, high = num; while (low <= high) { int mid = low + (high - low) / 2; if (guess(mid) == -1) { high = mid - 1; } else if (guess(mid) == 0) { return mid; } else if (guess(mid) == 1) { low = mid + 1; } } return -1; } public static void main(String[] args) { System.out.println(guessNumber(2126753390)); } } class GuessGame { /** * 假设1702766719是最终的结果 * Forward declaration of guess API. * * @param num your guess * @return -1 if num is lower than the guess number * 1 if num is higher than the guess number * otherwise return 0 */ public static int guess(int num) { if (num > 1702766719) { return -1; } else if (num == 1702766719) { return 0; } else { return 1; } } }
【每日寄语】 总之岁月漫长,然而值得等待。
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK