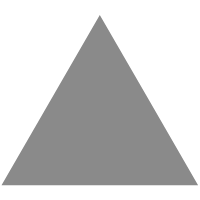
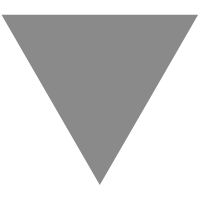
5 Useful Features of the Java 8 Date and Time API
source link: https://www.makeuseof.com/java-date-and-time-api-features/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
5 Useful Features of the Java 8 Date and Time API
Published 33 minutes ago
Dealing with dates and times can be tricky since there are many edge cases and complexities. Fortunately the Java 8 date and time API is here to help.
In 2014 the release of Java 8 introduced a new date and time API. You can easily access this API by importing the java.time package.
With the Java 8 date and time API, developers have easy access to dates, times, time zones, instants, durations, and periods. These features are available through an extensive list of classes in the java.time package.
In this tutorial, you’ll learn five useful features of the Java 8 date and time API.
1. Getting Your Local Time
The Java 8 date and time API comes with a class called LocalTime. This class provides a time in the hour-minute-second format.
Related: What Does API Stand For? Examples of How to Use APIs
To get your current time using this class, you’ll first have to create a new object of the LocalTime class. Then pass the object retrieved from the now() method to it. The newly created object will then store the current time of the program’s execution. This is because the now() method retrieves the current time from the system clock that is on your computer.
Using the LocalTime Class Example
import java.time.LocalTime;
public class DateTimeAPI {
public static void main(String[] args) {
// create a new time object that stores the local time
LocalTime time = LocalTime.now();
System.out.println("The current hour is: " + time.getHour());
System.out.println("The current minute is: " + time.getMinute());
System.out.println("The current second is: " + time.getSecond());
System.out.println("The current time to the nanosecond is: " + time);
}
}
The code above accomplishes several simple tasks using different LocalTime methods. Executing the code above produces the following output in the console.
The current hour is: 10
The current minute is: 45
The current second is: 19
The current time to the nanosecond is: 10:45:19.324014300
2. Get Your Local Date
You can get your local date through the Java 8 date and time API, in much the same way as you retrieve your local time. The only difference is that you use the LocalDate class instead of the LocalTime class.
Related: Learn How to Create Classes in Java
The LocalDate class uses the year-month-day structure to display the system date on your computer, with the help of the now() method. The LocalDate class provides access to over 50 different methods, but the ones that you might use more often are as follows.
- now() (takes no arguments and returns the current date)
- getYear() (takes no arguments and returns the current year)
- getMonth() (takes no arguments and returns the current month)
- getDayOfWeek() (takes no arguments and returns the current day of the week)
- getDayOfMonth() (takes no arguments and returns the current day of the month)
- getDayOfYear() (takes no arguments and returns the current day of the year)
Using the LocalDate Class Example
import java.time.LocalDate;
public class DateTimeAPI {
public static void main(String[] args) {
// create a new date object that stores the local date
LocalDate date = LocalDate.now();
System.out.println("The current year is: " + date.getYear());
System.out.println("The current month is: " + date.getMonth());
System.out.println("The current day of the week is: " + date.getDayOfWeek());
System.out.println("The current day of the Month is: " + date.getDayOfMonth());
System.out.println("The current day of the year is: " + date.getDayOfYear());
System.out.println("The current date is: " + date);
}
}
The code above produces the following output in the console.
The current year is: 2021
The current month is: SEPTEMBER
The current day of the week is: TUESDAY
The current day of the Month is: 14
The current day of the year is: 257
The current date is: 2021-09-14
3. Check if the Current Year Is a Leap Year
Among the many methods in the LocalDate class is the isLeapYear() method. You can use this method to check if a given year is a leap year. To check the current year, you can create a new LocalDate object with the now() method, and use the isLeapYear() method on the newly created object.
Using the isLeapYear() method on a LocalDate object will return a boolean value, which will be true if the year is a leap year and false otherwise.
Using the isLeapYear() Method Example
import java.time.LocalDate;
public class DateTimeAPI {
public static void main(String[] args) {
// create an object to store the current date.
LocalDate date = LocalDate.now();
// check if the current year is a leap year.
boolean isLeap = date.isLeapYear();
// get the current year from the current date.
int year = date.getYear();
// tell the user if the current year is a leap year.
if (isLeap) {
System.out.println(year + " is a leap year.");
} else {
System.out.println(year + " is not a leap year.");
}
}
}
The code above will produce the following output in the console.
2021 is not a leap year.
4. Get Your Local Date and Time
There’s a class in the Java 8 date and time API called LocalDateTime. As its name suggests, you can use this class to get the date and time at your current location.
The class has several important methods, but the now() method might be the one that you want to get familiar with first. The now() method of the LocalDateTime class returns the date and time of your current location. It uses the year-month-day T hour:minutes:seconds structure; where the date appears first and a capital t separates it from the time.
Using the LocalDateTime Class Example
import java.time.LocalDateTime;
public class DateTimeAPI {
public static void main(String[] args) {
// create a new date object that stores the local date
LocalDateTime dateTime = LocalDateTime.now();
// the current time
System.out.println("The current hour is: " + dateTime.getHour());
System.out.println("The current minute is: " + dateTime.getMinute());
System.out.println("The current second is: " + dateTime.getSecond());
// the current date
System.out.println("The current year is: " + dateTime.getYear());
System.out.println("The current month is: " + dateTime.getMonth());
System.out.println("The current day of the week is: " + dateTime.getDayOfWeek());
System.out.println("The current day of the Month is: " + dateTime.getDayOfMonth());
System.out.println("The current day of the year is: " + dateTime.getDayOfYear());
// the current date and time
System.out.println("The current date and time is: " + dateTime);
}
}
The code above produces the following output in the console.
The current hour is: 11
The current minute is: 9
The current second is: 30
The current year is: 2021
The current month is: SEPTEMBER
The current day of the week is: TUESDAY
The current day of the Month is: 14
The current day of the year is: 257
The current date and time is: 2021-09-14T11:09:30.009675800
As you can see from the output above, the LocalDateTime class can generate a date and time combination, as well as the individual values (such as the current month), that you can get from the LocalDate and LocalTime classes.
5. Get the Current Date and Time of a Location Using Its Time Zone
As you’ve seen so far, the Java 8 date and time API has many useful features. Another useful one is its ability to get the time zone of your current location, or the date and time at a location using its time zone.
Using the ZonedDateTime Class Example
import java.time.ZoneId;
import java.time.ZonedDateTime;
public class DateTimeAPI {
public static void main(String[] args) {
// create a new object to store your local date, time, and time-zone.
ZonedDateTime zDateTime = ZonedDateTime.now();
// create a zoneId object to store your local time-zone
ZoneId localTimeZone = zDateTime.getZone();
// create a new zoneId object to store the zoneId provided by the user
ZoneId newZoneId = ZoneId.of("GMT-03:00");
// create an object to store the current date, time, and time-zone
// retrieved using the zoneId provide by the user
ZonedDateTime newLocation = ZonedDateTime.now(newZoneId);
System.out.println("The date, time, and time-zone at your current location is: " + zDateTime);
System.out.println("The time-zone at your current location is: " + localTimeZone);
System.out.println("The date, time, and time-zone at the new time-zone is: " + newLocation );
}
}
A lot is happening in the code above. Like the previous classes, the ZoneDateTime class uses the now() method, which in this case returns an object with the current date, time, and time zone.
However, unlike the previous examples, the ZoneDateTime class takes a time zone from the user and returns the appropriate date and time.
The code above will produce the following output in the console.
The date, time, and time-zone at your current location is: 2021-09-14T15:00:17.029010300-05:00[GMT-05:00]
The time-zone at your current location is: GMT-05:00
The date, time, and time-zone at the new time-zone is: 2021-09-14T17:00:17.042989-03:00[GMT-03:00]
As you can see from the output above, the GMT-03:00 time zone is two hours ahead of the GMT-05:00 time zone.
Learn More About APIs
In this tutorial, you’ve learned about five top features that come with the Java 8 date and time API. This list is by no means complete, and there are other features of the API that you can explore.
However, if you’re curious about how APIs work in general, there are resources to help you with that too.
About The Author
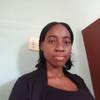
Kadeisha Kean (26 Articles Published)
Kadeisha Kean is a Full-Stack Software Developer and Technical/Technology Writer. She has the distinct ability to simplify some of the most complex technological concepts; producing material that can be easily understood by any technology novice. She is passionate about writing, developing interesting software, and traveling the world (through documentaries).
Subscribe to our newsletter
Join our newsletter for tech tips, reviews, free ebooks, and exclusive deals!
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK