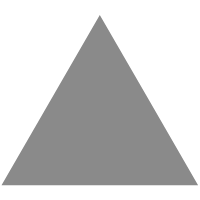
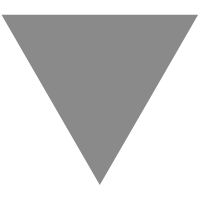
Is C# Getting Too Complex?
source link: https://medium.com/nerd-for-tech/is-c-getting-too-complicated-for-its-own-good-83c149a6faca
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
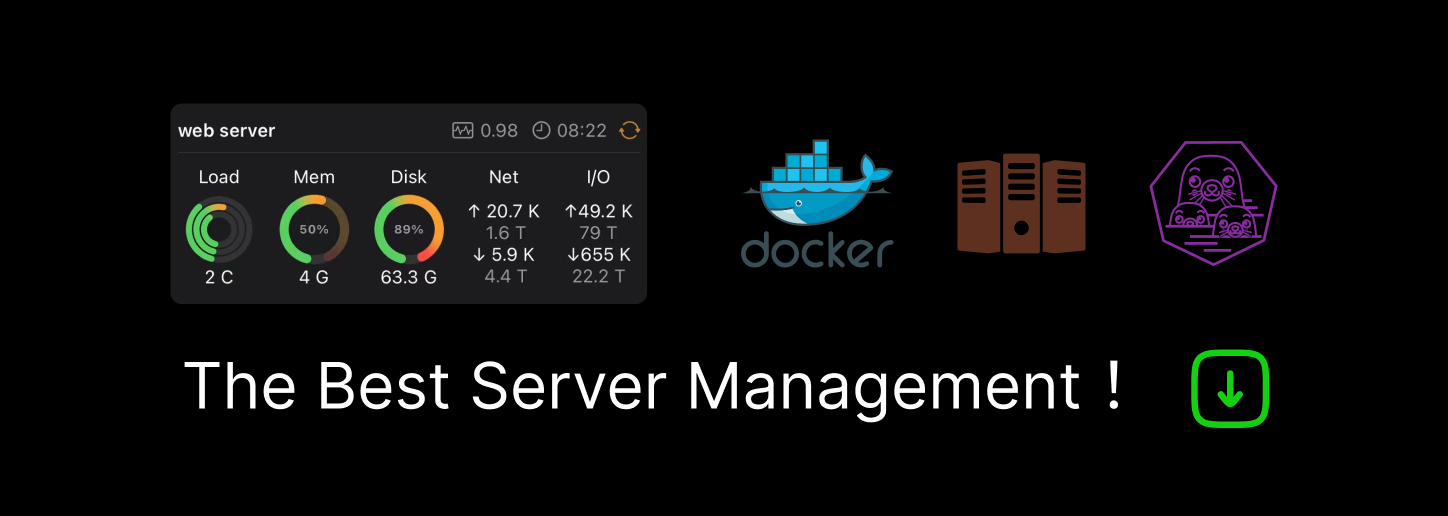
Is C# Getting Too Complex?
I’ll start off by saying that I’m a big fan of C#. It’s the language I recommend for new projects, it’s what I recommend to budding programmers, and it’s the language I think in when problem solving. It wasn’t the first language I learned (that would be Java) but once I started playing with C#, I was hooked. Recently though, I’ve been having a nagging feeling that C# is getting too complex for its own good. Every couple of years Microsoft releases a new version, the language becomes ever more packed with features, and it feels to me that it’s starting to drag the language down. Don’t get me wrong, not all the new stuff is bad. LINQ, for example, is amazing. It was introduced in .NET 3.5, alongside C# 3, and while it’s not a part of the language itself, the interaction between C# and LINQ is so seamless that it might as well be. I use LINQ daily, and I couldn’t imagine giving it up. The trouble is a lot of the new features introduced are not productivity-boosting paradigms on par with LINQ, but syntactical sugar that just add to the complexity of the language without bringing anything to the table besides saving a few lines of code or a couple of curly braces. So what follows is a whirlwind tour of different ways of doing some things in C#. When you reach the end, you can decide for yourself if the language is heading in a good direction.
Assignment
Ask a group of C# developers how to create two integers (I’m being deliberately vague here, to give our hypothetical C# developers some leeway). These are some of the answers you’ll get:
Five ways of doing the same thing, and this is one of the simplest things you could do. Imagine you’re a total newbie to programming, or maybe you’re coming from JavaScript, you’re going to try this and suddenly your program isn’t going to work:
Objects
How do we create objects? Like this?:
Or like this?:
Or maybe?:
Should we use an init only setter?:
A read-only property?:
Should our object not be defined as a class at all, but as a struct?:
Maybe we should make it a readonly struct?:
Or perhaps a…ref struct…?:
Without googling, can you tell me the difference between these two structs?:
Or maybe we should just make it a record?:
It’s kind of ironic that as of this writing the GitHub C# syntax highlighting doesn’t work when records are defined this way. Errors like this one sneak in when things become complex.Of course if we do define Animal
as a record, we can shorten our definition to this:
When should we use a class versus a struct versus a record anyway?
Pass by Value, Pass by Reference
Back when things were simpler, value types were passed by value, and reference types were passed by reference. The following will print 2, followed by 1:
Nowadays you can pass value types by reference, like this:
And that will print two 2's.
We could also return references:
And this prints 2 and 3. If you don’t like that, you can make the returned value ref readonly, and it will print two 2’s again:
You could also do this:
And that will print two 1’s. The in
keyword causes the i
to be passed by reference, but it prevents the method from modifying it.
These are contrived, silly examples, but keep in mind that this is working C# code, and there’s nothing stopping someone from using these features in a real codebase, one that you might have to maintain some day.
Sugar, Sugar
What if you have a list of integers, and you want to create a list of doubles from it? How do you do it? Which way is better?:
What about methods?
Constructors?:
Which seems clearer to you?:
I haven’t even talked about the async/await, nullable types, generics, dynamic types, anonymous types, lambdas, delegates, local functions…but I think I only need to make this article so long to make my point.
Let me count the ways…
You may think I’m being unfair here. After all, the features I’ve covered in this article, and more, are well-documented. You can look up the differences between classes, structs, records, and ref structs. None of this stuff is a mystery. But my point isn’t that this stuff can’t be learned, it’s that you have to take the time to learn it.
Recommend
-
34
For hacking out Software features David Moore MSc...
-
9
-
7
Getting Past Bash Argument list too long Jun 25, 2020 Right now I have a directory with about 750,000 odd files in i...
-
12
The new visual update is currently in the testing stage Microsoft is working on bringing a dark mode to Office apps on all platforms, and the next one in the queue appears to be none other than the...
-
9
Modern CI is Too Complex and Misdirected April 07, 2021 at 09:00 AM | categories:
-
5
March 7, 2022 Tiny worms make complex decisions, too Salk scientists surprised to discover flexible decision-making capabilities in a worm with just 302 neurons...
-
7
Not only is excellent VR shooter, Compound, getting a full PC VR release soon – it’s coming to Quest 2, too. Developer Bevan McKechnie confirmed as much to UploadVR following the reveal of last week’s news that the full version of the game...
-
6
Marc's Blog Getting into formal specification, and getting my team into it too Getting started is the hard part ...
-
7
When is a martech stack too complex?
-
6
It’s Getting Too Hot to Make SnowSome ski resorts rely on machines to keep powder on the slopes. But snow guns guzzle water, are energy-intensive, and need cool temperatures to operate....
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK