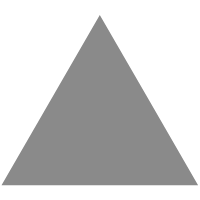
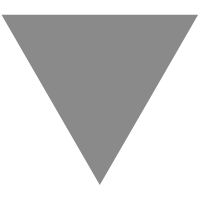
SQLite基础入门教程
source link: http://www.androidchina.net/5226.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
什么是SQLite
SQLite是一款非常轻量级的关系数据库系统,支持多数SQL92标准。SQLite在使用前不需要安装设置,不需要进程来启动、停止或配置,而其他大多数SQL数据库引擎是作为一个单独的服务器进程,被程序使用某种内部进程通信(典型的是TCP/IP),完成发送请求到服务器和接收查询结果的工作,SQLite不采用这种工作方式。使用SQLite时,访问数据库的程序直接从磁盘上的数据库文件读写,没有中间的服务器进程。使用SQLite一般只需要带上一个dll,就可以使用它的全部功能。
SQLite的主要应用场景有作为手机应用的数据库以及小型桌面软件的数据库。
安装使用SQLite
sqlite的官方下载地址为http://www.sqlite.org/download.html,上面提供了多种版本的sqlite,我选择下载名称为sqlite-shell-win32-x86-3080500.zip 的版本。下载后就直接解压到磁盘上,可以看到解压后只有sqlite3.exe这个文件。
接下来需要将sqlite加入到path环境变量中(加入环境变量是为了更加方便地使用sqlite),右键我的电脑-属性-高级系统设置-环境变量,在系统变量中找到Path,将解压的文件夹目录加入到后面(注意是文件夹目录,例如我本机的目录 E:\Tools\sqlite)。打开cmd,输入sqlite3,如果弹出以下消息,就表示成功了。
sqlite常用操作
1. 新建一个数据库文件
>命令行进入到要创建db文件的文件夹位置
>使用命令创建数据库文件: sqlite3 所要创建的db文件名称
>使用命令查看已附加的数据库文件: .databases
2. 打开已建立的数据库文件
>命令行进入到要打开的db文件的文件夹位置
>使用命令行打开已建立的db文件: sqlite3 文件名称(注意:假如文件名称不存在,则会新建一个新的db文件)
3. 查看帮助命令
>命令行直接输入sqlite3,进去到sqlite3命令行界面
>输入.help 查看常用命令
使用sqlite管理工具
shell脚本虽然提供了很强大的功能,但是使用起来还是不够方便,幸运的是,sqlite有很多开源而且优秀的DBMS!
这里我将使用一款叫做SQLiteSPY的软件,官网地址为http://www.yunqa.de/delphi/doku.php/products/sqlitespy/index,这个软件是绿色免安装版,解压直接运行就可以了。
可以看到,SQLiteSpy的界面布局和SQLServer很相近,操作起来很方便,这里就不在继续详细介绍了。(要知道的一点就是单纯使用这个软件也可以创建和使用sqlite数据库,不需要与上面提到的shell工具关联)
C#使用System.Data.SQLite.dll访问数据库
SQLite提供了用于C#调用的dll,下载地址为http://system.data.sqlite.org/index.html/doc/trunk/www/downloads.wiki,注意根据.NET FRAMEWORK版本下载对应的组件。在项目中只要引入System.Data.SQLite.dll这个组件,就可以实现数据库操作了。由于SQLite.dll实现了ADO.NET的接口,所以熟悉ADO.NET的人上手SQLite.dll也是非常快的。DEMO数据库表的结构为:
CREATE TABLE hero ( hero_id INT NOT NULL PRIMARY KEY, hero_name NVARCHAR(10) NOT NULL );
比较需要注意到一点是数据库连接字符串,SQLite使用的连接字符串比较简单,只要写上数据库文件的引用路径就可以了。DEMO是一个控制台应用程序,增删查改的实例代码如下:
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.Threading.Tasks; using System.Data.Common; using System.Data.SQLite; namespace ConsoleApp { class Program { static readonly string DB_PATH = "Data Source=E:/database/sqlite/arena.db"; static void Select() { using (SQLiteConnection con = new SQLiteConnection(DB_PATH)) { con.Open(); string sqlStr = @"SELECT * FROM hero"; using(SQLiteCommand cmd = new SQLiteCommand(sqlStr,con)) { using (SQLiteDataReader dr = cmd.ExecuteReader()) { while (dr.Read()) { Console.WriteLine(dr["hero_id"].ToString() + dr["hero_name"]); } } } } } static void Insert() { using (SQLiteConnection con = new SQLiteConnection(DB_PATH)) { con.Open(); string sqlStr = @"INSERT INTO hero VALUES ( 1, '萨满' )"; using(SQLiteCommand cmd = new SQLiteCommand(sqlStr,con)) { cmd.ExecuteNonQuery(); } } } static void Update() { using (SQLiteConnection con = new SQLiteConnection(DB_PATH)) { con.Open(); string sqlStr = @"UPDATE hero SET hero_name = '盗贼' WHERE hero_id = 1"; using (SQLiteCommand cmd = new SQLiteCommand(sqlStr, con)) { cmd.ExecuteNonQuery(); } } } static void Delete() { using (SQLiteConnection con = new SQLiteConnection(DB_PATH)) { con.Open(); string sqlStr = @"DELETE FROM hero"; using (SQLiteCommand cmd = new SQLiteCommand(sqlStr, con)) { cmd.ExecuteNonQuery(); } } } static void Main(string[] args) { Insert(); Select(); Update(); Select(); Delete(); } } }
转载请注明:Android开发中文站 » SQLite基础入门教程
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK