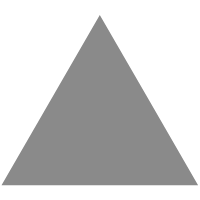
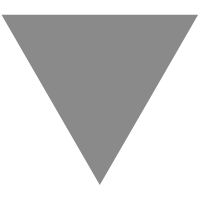
#JavaScript 根据需要动态加载脚本并设置自定义参数
source link: https://xmanyou.com/javascript-dynamically-load-script-and-set-parameters/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
#JavaScript 根据需要动态加载脚本并设置自定义参数
在html文件里,加载javascript脚本,可以通过添加<script src="js脚本"></script>
的方法来加载。
但是,有时候想要根据需要动态加载不同的脚本,或者设置不同的参数,需要怎么做呢?
可以利用DOM document的接口来实现。具体步骤:
- 1). 创建script元素
let scriptElement = document.createElement('script')
- 2). 设置script的脚本
scriptElement.src = "js脚本路径"
- 3). 设置脚本的参数
scriptElement.setAttribute(key, value)
- 4). 添加到document树上
( document.getElementsByTagName("head")[0] || document.documentElement ).appendChild( scriptElement );
注意
- 1). 设置Script的自定义参数使用的是setAttribute方法,而不是直接
scriptElement[key] = value
。 - 2). 如果要异步加载,需要设置
scriptElement.async = true
HTMLScriptElement 参考
https://developer.mozilla.org/en-US/docs/Web/API/HTMLScriptElement
完整示例(TypeScript)
async function loadJsAsync(src:string, async:boolean=false, options?:any) {
return new Promise<void>((resolve, reject) => {
const script = document.createElement('script');
script.src = src;
script.async = async;
if(options) {
for(const key in options) {
script.setAttribute(key, options[key]);
}
}
let onload = () => {
script.removeEventListener('load', onload);
// 非异步加载的话,需要等待onload返回
if(!async) {
resolve();
}
};
script.addEventListener('load', onload);
script.addEventListener('error', (err) => {
console.error(err);
reject(new Error(`Failed to load ${src}`));
});
( document.getElementsByTagName("head")[0] || document.documentElement ).appendChild.appendChild(script);
// 异步加载直接返回
if(async){
resolve();
}
});
}
loadJsAsync(url, true, attributes);
gist地址
https://gist.github.com/zhangzhibin/9bfc7debf08a5300c0101e1c1f19a904
// JavaScript 根据需要动态加载脚本并设置参数 // refer to https://xmanyou.com/javascript-dynamically-load-script-and-set-parameters/ async function loadJsAsync(src:string, async:boolean=false, options?:any) { return new Promise<void>((resolve, reject) => { const script = document.createElement('script'); script.src = src; script.async = async; if(options) { for(const key in options) { // script[key] = options[key]; script.setAttribute(key, options[key]); } }
let onload = () => { script.removeEventListener('load', onload); // resolve in onload callback for async loading if(!async) { resolve(); } };
script.addEventListener('load', onload); script.addEventListener('error', (err) => { console.error(err); reject(new Error(`Failed to load ${src}`)); });
// document.head.appendChild(script); ( document.getElementsByTagName("head")[0] || document.documentElement ).appendChild( script );
// resolve immediately for sync loading if(async){ resolve(); } }); }
export { loadJsAsync }
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK