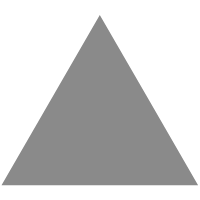
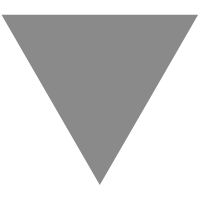
Using the Promise all(), race(), allSettled() and any() in Javascript
source link: http://www.js-craft.io/blog/using-the-promise-all-race-allsettled-and-any-in-javascript/
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
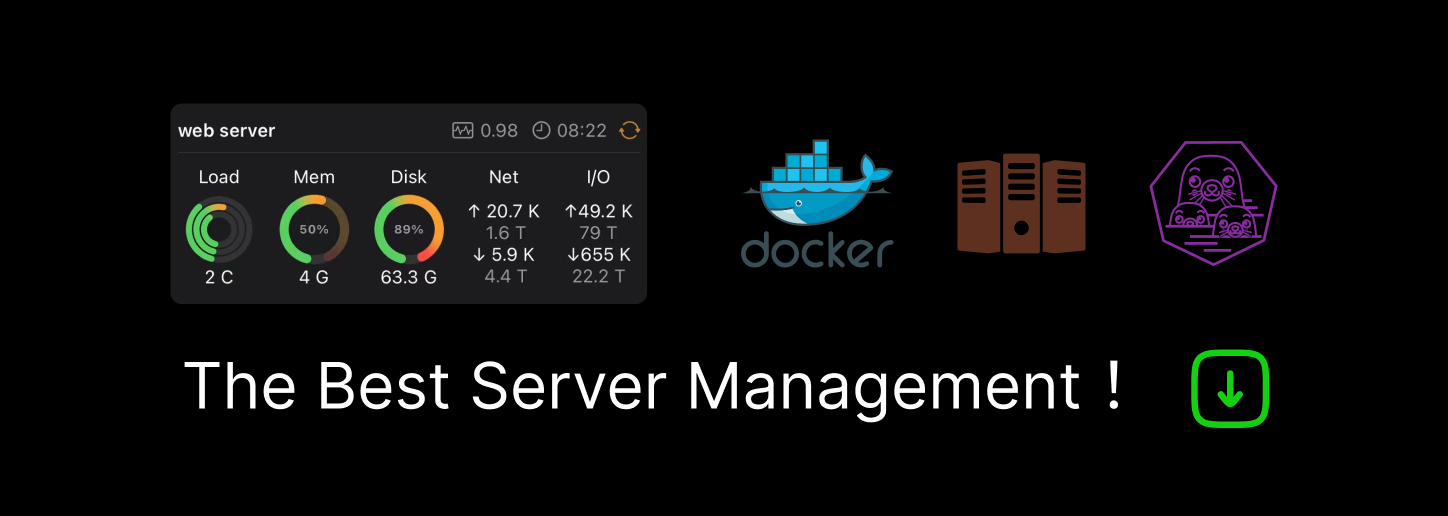
Using the Promise all(), race(), allSettled() and any() in Javascript
With the many options for solving multiple multiple concurrent promises things can get a bit confusing. Let's see the options one by one:
-
Promise.all()
- resolves only when all of the given promises are resolved. It rejects immediately if one promises fails and returns the first rejection message.Promise.all([getUserById(1),getUserById(2), getUserById(3)]) .then((users) => { // will return all the users }) .catch((error) => { // will fail when the first promise fails });
-
Promise.allSettled()
- it will resolve when all of the given promises have either been fulfilled or rejected.Promise.allSettled([getUserById(1), getUserById(2), getUserById(3)]) .then((results) => { // the items in results can be either a user or an error // it waits for all the promises to have a result. })
-
Promise.race()
- will resolve when the first of the given concurrent promises fulfills or rejects.Promise.race([getUserById(1), getUserById(2), getUserById(3)]) .then(([user]) => { // will return the first retrevied user }) .catch((error) => { // will fail at the first rejected promise });
-
Promise.any()
- It will resolve as soon as one of the promises is fulfilled, but it won’t reject until it’s done resolving all of the promises. It tries to fulfill at least one promise.Promise.any([getUserById(1), getUserById(2), getUserById(3)]) .then(([user]) => { // will return the first retrevied user }) .catch((error) => { // will fail only when all the promises have failed });
I hope you have enjoyed this article and if you would like to get more articles about React and frontend development you can always sign up for my email list.
Newsletter subscribe:
Recommend
-
46
Photo by Ryan Franco on
-
33
I’ve recently read the Promise combinators article in v8 blog. It's about two upcoming...
-
15
I've recently read the Promise combinators article in v8 blog. It's about two upcoming methods in Promise API: Promise.allSettled() and...
-
19
Promises have not been a new concept in the javascript community. They have existed in the ecosystem for a long time. JavaScript promises existed even before they were officially made a part of the ECMAScript spec in ES6....
-
42
Mastering Promise.allSettled in ReactEvery year a new version of javascript comes out. This year’s version, ES2020, released with a bunch of cool new features. While a lot of features got a lot of hype, a ne...
-
9
-
13
Creating a JavaScript promise from scratch, Part 6: Promise.all() and Promise.allSettled() Promise.all() and Promise.allSettled() work on any number of promises to allow you to know when all of the promises have resolved...
-
9
Nick Scialli • May 13, 2021 • 🚀 4 minute readPromise.all and Promise.allSettled are two different ways to handle an array of Promises in JavaScript. Let’s compare them a...
-
6
Promise 对象是ECMAScript 6中新增的对象,主要将 JavaScript 中的异步处理对象和处理规则进行了规范化。前面介绍了《Promise.any() 原理解析及使用指南》和《
-
4
This tutorial will teach you how to use promises to wait in JavaScript. In this tutorial, I will teach you about the Promise.all() and Promise.allSettled() methods and how you can use them to work with multiple pro...
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK