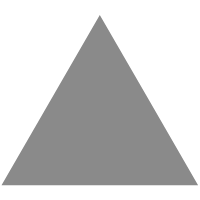
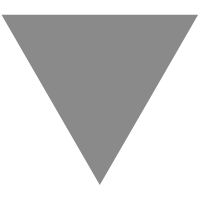
How to get the start and end times of a day
source link: https://www.codesd.com/item/how-to-get-the-start-and-end-times-of-a-day.html
Go to the source link to view the article. You can view the picture content, updated content and better typesetting reading experience. If the link is broken, please click the button below to view the snapshot at that time.
How to get the start and end times of a day
In my c# app, I pass a string variable that is of format yyyymmdd-yyyymmdd that represents a from and to date. I want to get the start and end times for these dates respectively. Currently I have the below code but was wondering if there was more of an elegant solution?
So for pdr = 20090521-20090523 would get "20090521 00:00:00" and "20090523 23:59:59"
private void ValidateDatePeriod(string pdr, out DateTime startDate,
out DateTime endDate)
{
string[] dates = pdr.Split('-');
if (dates.Length != 2)
{
throw new Exception("Date period is of incorrect format");
}
if (dates[0].Length != 8 || dates[1].Length != 8)
{
throw new Exception("Split date periods are of incorrect format");
}
startDate = DateTime.ParseExact(dates[0] + " 00:00:00",
"yyyyMMdd HH:mm:ss", null);
endDate = DateTime.ParseExact(dates[1] + "23:59:59",
"yyyyMMdd HH::mm:ss", null);
}
If you are only worried about .Net precision...
startDate = DateTime.ParseExact(dates[0], "yyyyMMdd");
endDate = DateTime.ParseExact(dates[1], "yyyyMMdd").AddTicks(-1).AddDays(1);
You really don't need to concatenate extra values onto the string for the time portion.
As an addendum, if you are using this for a query against, for example, a database...
startDate = DateTime.ParseExact(dates[0], "yyyyMMdd");
endDate = DateTime.ParseExact(dates[1], "yyyyMMdd").AddDays(1);
With a query of...
WHERE "startDate" >= @startDate AND "endDate" < @endDate
Then the precision issues noted in the comments won't really matter. The endDate in this case would not be part of the range, but the outside boundary.
Recommend
About Joyk
Aggregate valuable and interesting links.
Joyk means Joy of geeK